Boosting QA Efficiency: Mastering Test Monitoring & Control
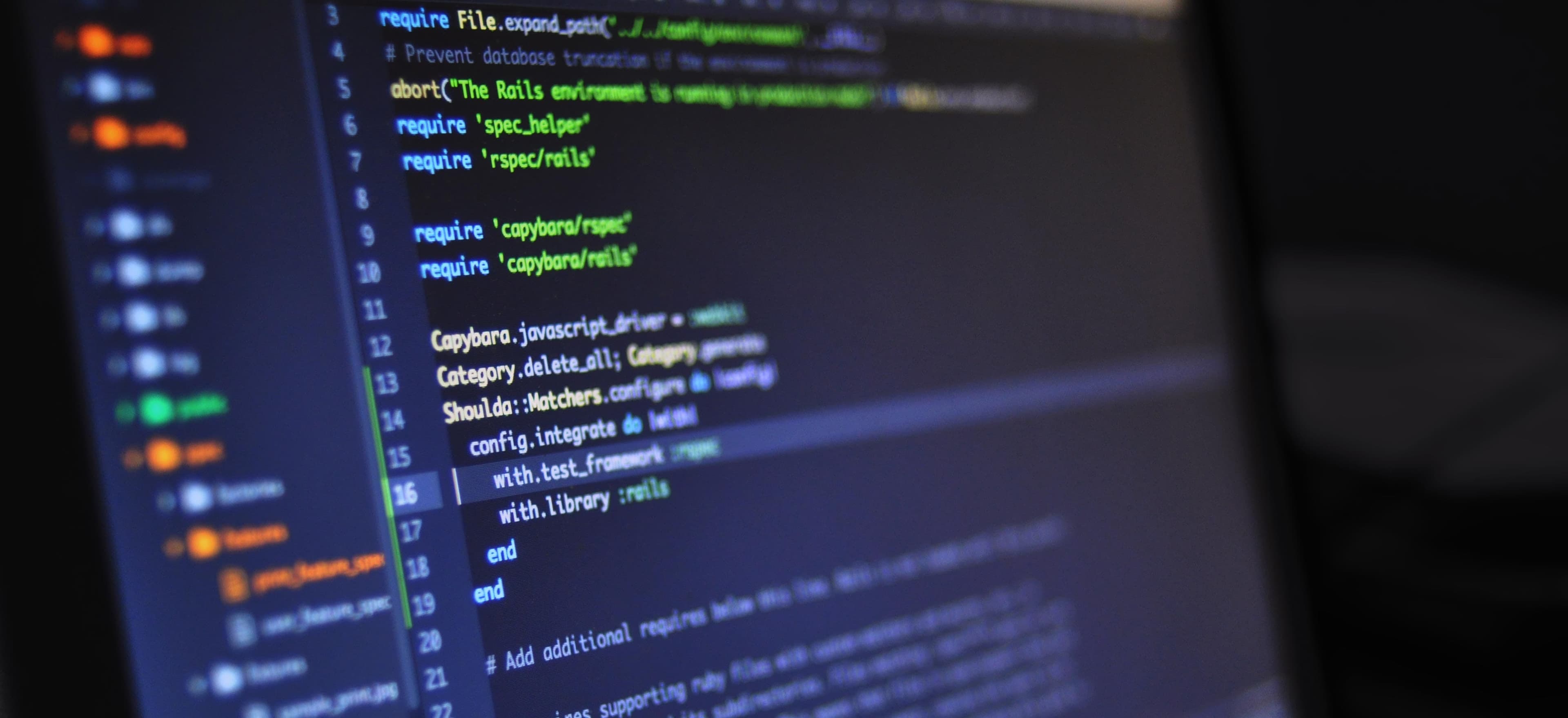
- Published on
Boosting QA Efficiency: Mastering Test Monitoring & Control
In the realm of Software Development, Quality Assurance (QA) plays an indispensable role in ensuring the final product meets the standards of performance, security, and reliability. Test Monitoring and Control are fundamental components of the QA process, enabling teams to efficiently manage, observe, and adjust testing activities. This article delves into the essence of Test Monitoring & Control in QA, highlighting its significance and exploring best practices in Java to elevate QA efficiency.
Understanding Test Monitoring & Control
Test Monitoring involves the real-time observation and tracking of test execution, providing insights into the progress, results, and potential roadblocks. On the other hand, Test Control revolves around the ability to intervene, modify, or optimize test processes based on the monitored data. Together, they form a dynamic duo that empowers QA teams to navigate the complexities of testing scenarios with agility and precision.
Implementing Test Monitoring & Control in Java
1. Logging Frameworks for Comprehensive Monitoring
Logging frameworks like Log4j and SLF4J are instrumental in capturing detailed information during test execution. Leveraging these frameworks to record key events, errors, and debugging data provides valuable visibility into the test process, facilitating effective monitoring.
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class SampleTestClass {
private static final Logger logger = LogManager.getLogger(SampleTestClass.class);
public void performTest() {
logger.info("Initiating test execution");
// Test logic goes here
if (testFails) {
logger.error("Test failed due to reason XYZ");
}
}
}
Why it works: The use of logging frameworks ensures that essential information is captured, aiding in proactive monitoring and issue identification during testing.
2. Real-time Reporting with TestNG
Utilizing the comprehensive reporting capabilities of TestNG allows for effective real-time monitoring of test outcomes. TestNG's in-built reports and listeners offer detailed insights into test results, execution time, and potential bottlenecks, thereby enabling prompt decision-making and control actions.
import org.testng.annotations.Listeners;
import org.testng.annotations.Test;
@Listeners(value = CustomTestListener.class)
public class SampleTestClass {
@Test
public void performTest() {
// Test logic goes here
}
}
Why it works: TestNG's reporting and listener features furnish real-time visibility into test executions, empowering control over the testing process based on live data.
3. Dynamic Test Orchestration with JUnit 5
Incorporating dynamic test orchestration capabilities offered by JUnit 5 allows for flexible control over the flow and sequencing of test scenarios. Leveraging annotations such as @BeforeEach and @AfterEach enables the establishment of pre-test and post-test actions, amplifying the control and monitoring capabilities within the test environment.
import org.junit.jupiter.api.*;
public class SampleTestClass {
@BeforeEach
public void setUp() {
// Actions to be performed before each test
}
@Test
public void performTest() {
// Test logic goes here
}
@AfterEach
public void tearDown() {
// Actions to be performed after each test
}
}
Why it works: JUnit 5's dynamic orchestration features empower precise control and monitoring of test sequences, fostering adaptability within the testing framework.
Best Practices for Optimized Test Monitoring & Control
-
Establish Clear Monitoring Objectives: Define specific key performance indicators (KPIs) and expected outcomes to focus the monitoring efforts and streamline control actions.
-
Automate Monitoring Processes: Integrate automated monitoring tools and scripts into the testing environment to ensure continuous observation without manual intervention.
-
Adapt Control Strategies: Tailor control actions based on real-time monitoring data, allowing for swift adjustments and optimizations during testing.
-
Utilize Visual Dashboards: Implement visual dashboards and reporting mechanisms to present monitoring insights in a clear, intuitive manner, facilitating quick decision-making.
-
Continuous Feedback Loop: Foster a feedback-driven approach by integrating monitoring data into the development cycle, enabling iterative improvements and proactive control adjustments.
My Closing Thoughts on the Matter
In the pursuit of optimizing QA efficiency, mastering Test Monitoring & Control is paramount. Through the integration of robust monitoring frameworks, real-time reporting tools, and dynamic orchestration capabilities, Java empowers QA teams to elevate their testing endeavors. Embracing best practices in test monitoring and control fosters proactive decision-making, rapid issue resolution, and overall enhancement of software quality. As the QA landscape continues to evolve, adept utilization of Test Monitoring & Control in Java is pivotal in shaping resilient and high-performing software products.
Checkout our other articles