Avoiding Null in Java Collections: Best Practices
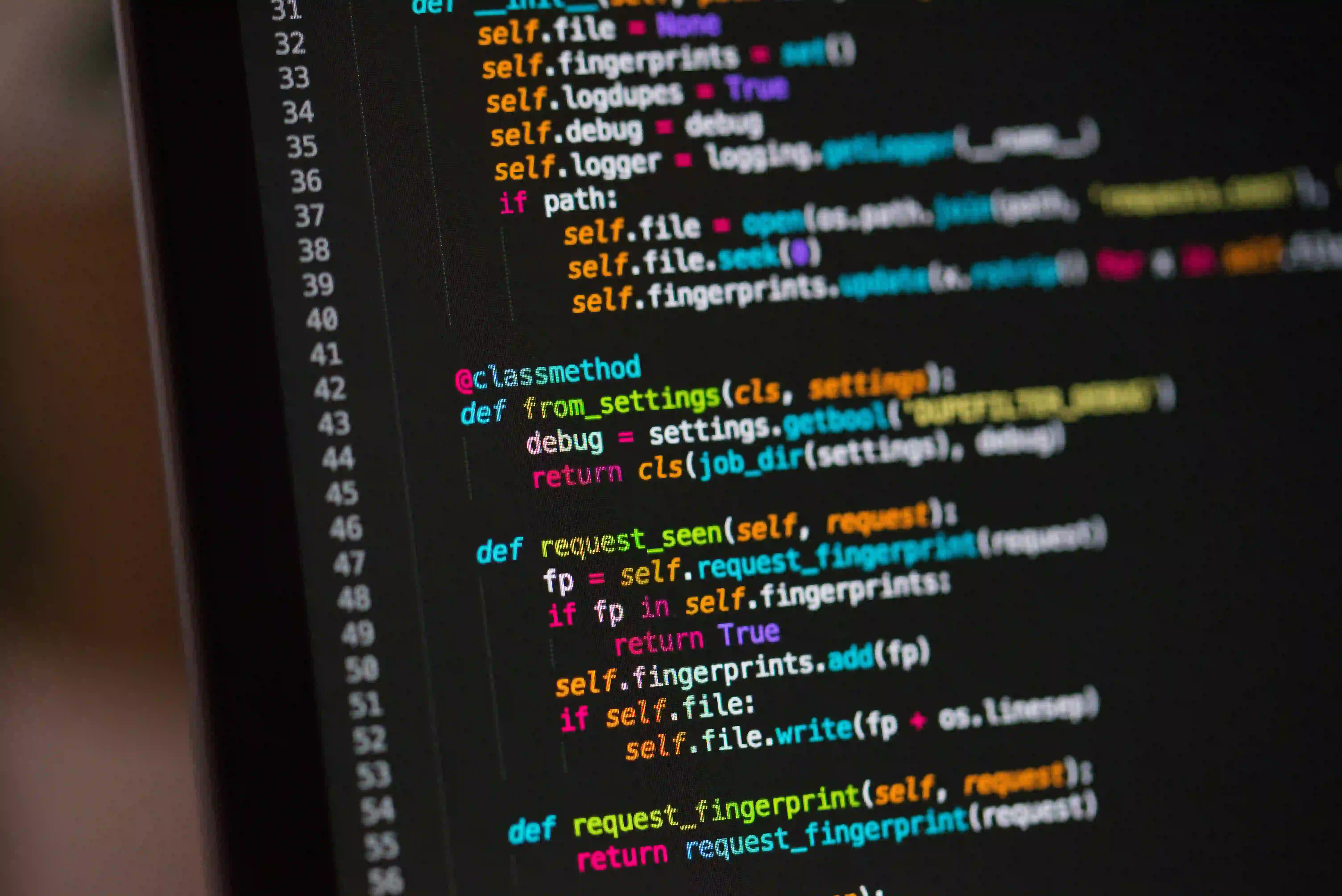
Avoiding Null in Java Collections: Best Practices
Before We Begin
Handling null values in Java, especially within collections, is crucial for creating clean and robust code. When not properly managed, null values can lead to NullPointerExceptions
, increased complexity, and potential bugs. In this article, we will explore best practices for avoiding null in Java collections. We'll cover a range of strategies, from using Optional
to employing the null object pattern, to ensure that developers can work effectively with collections while minimizing the impact of null values.
Understanding Null and Its Impact
The Null Reference in Java
In Java, a null reference signifies the absence of a value. It indicates that a variable does not refer to any object or array. The concept of null originates from the need to represent the lack of a value and has been a part of the Java programming language since its inception.
Problems Associated with Null in Collections
Null values can pose several challenges when they find their way into collections. One of the most common issues is NullPointerException
, which occurs when an operation is attempted on a null-reference object. Handling nulls in collections also increases the complexity of the code and can lead to bugs that are difficult to trace and fix.
Strategies to Avoid Null in Java Collections
Let's delve into a variety of methods and strategies for minimizing or eliminating null values in Java collections.
Using Optional
Introduced in Java 8, Optional
is a class designed to represent the presence or absence of a value. When working with collections, using Optional
can force explicit null checks, thereby reducing the occurrence of NullPointerExceptions
. Here's an exemplary code snippet showcasing how to use Optional
instead of null in collections:
List<Optional<String>> listOfOptionalStrings = new ArrayList<>();
listOfOptionalStrings.add(Optional.of("StringValue"));
listOfOptionalStrings.add(Optional.empty()); // Instead of using null
By leveraging Optional
, developers can improve code readability and reduce the likelihood of encountering null-related issues.
Initializing Collections
A simple yet effective approach to avoiding null in Java collections is to initialize them instead of leaving them null. By explicitly initializing collections to empty instances, developers can eliminate potential NullPointerExceptions
when accessing the collection:
List<String> myStrings = new ArrayList<>(); // Instead of List<String> myStrings = null;
By adopting this practice, developers can ensure that the collections always have a valid instance and minimize the risk of null-related errors.
Using Null Object Pattern
The null object pattern is a design pattern that employs polymorphism to provide a default object instead of null. By creating a null object class that can be added to collections instead of null references, developers can reduce the need for null checks and provide a consistent, non-null behavior.
For instance, a NullString
class could implement the String
interface and provide default or empty implementations for its methods. This approach ensures that the collection always contains objects, even when a null-like behavior is required.
Assertions and Preconditions
Utilizing assertions and preconditions, such as the Objects.requireNonNull()
method, can prevent null values from being added to collections. At the point of insertion, developers can validate that the object is not null before adding it to the collection, catching potential null issues early in the execution process:
List<String> list = new ArrayList<>();
String str = "Hello";
list.add(Objects.requireNonNull(str, "str must not be null"));
By incorporating this approach, developers enforce the presence of non-null values in collections, contributing to the overall null safety of the codebase.
Best Practices for Handling Existing Nulls in Collections
In scenarios where collections already contain null values, there are strategies for effectively dealing with them. Java 8 streams can be leveraged to filter out nulls, providing a streamlined approach to working with collections. Additionally, utilizing external libraries like Apache Commons Collections or Google Guava can offer utilities for managing nulls within collections in a more structured and efficient manner.
Using Java Streams to Filter Nulls
Java 8 streams provide an elegant solution for filtering out null values from collections:
List<String> stringsWithNoNulls = strings.stream()
.filter(Objects::nonNull)
.collect(Collectors.toList());
By employing streams, developers can seamlessly filter out null values from collections, ensuring that only non-null elements are retained.
External Libraries for Null-safe Collections
In addition to native Java functionality, developers can explore external libraries that provide utilities for managing nulls within collections in a null-safe manner. Libraries like Apache Commons Collections and Google Guava offer robust support for null-safe operations, enhancing the overall null safety of collections within Java applications.
Key Takeaways
In conclusion, managing null values within Java collections is critical to ensuring code stability and readability. By employing strategies such as using Optional
, initializing collections, leveraging the null object pattern, and validating input with assertions and preconditions, developers can significantly reduce the impact of null values. Furthermore, handling existing nulls within collections can be effectively addressed through the use of Java streams and external libraries that provide null-safe operations. Embracing these best practices can lead to more resilient and comprehensible codebases, ultimately benefiting both developers and end-users.
For further exploration of null safety in Java, consider referring to the official Java documentation for Optional
and investigating third-party libraries that offer additional support for null-safe collection operations.
Ultimately, by prioritizing the avoidance and effective management of null values in Java collections, developers can cultivate codebases that are more resilient and maintainable, leading to a positive impact on the overall quality and reliability of their software products.