Avoiding Duplicate Updates: REST API Resource Solutions
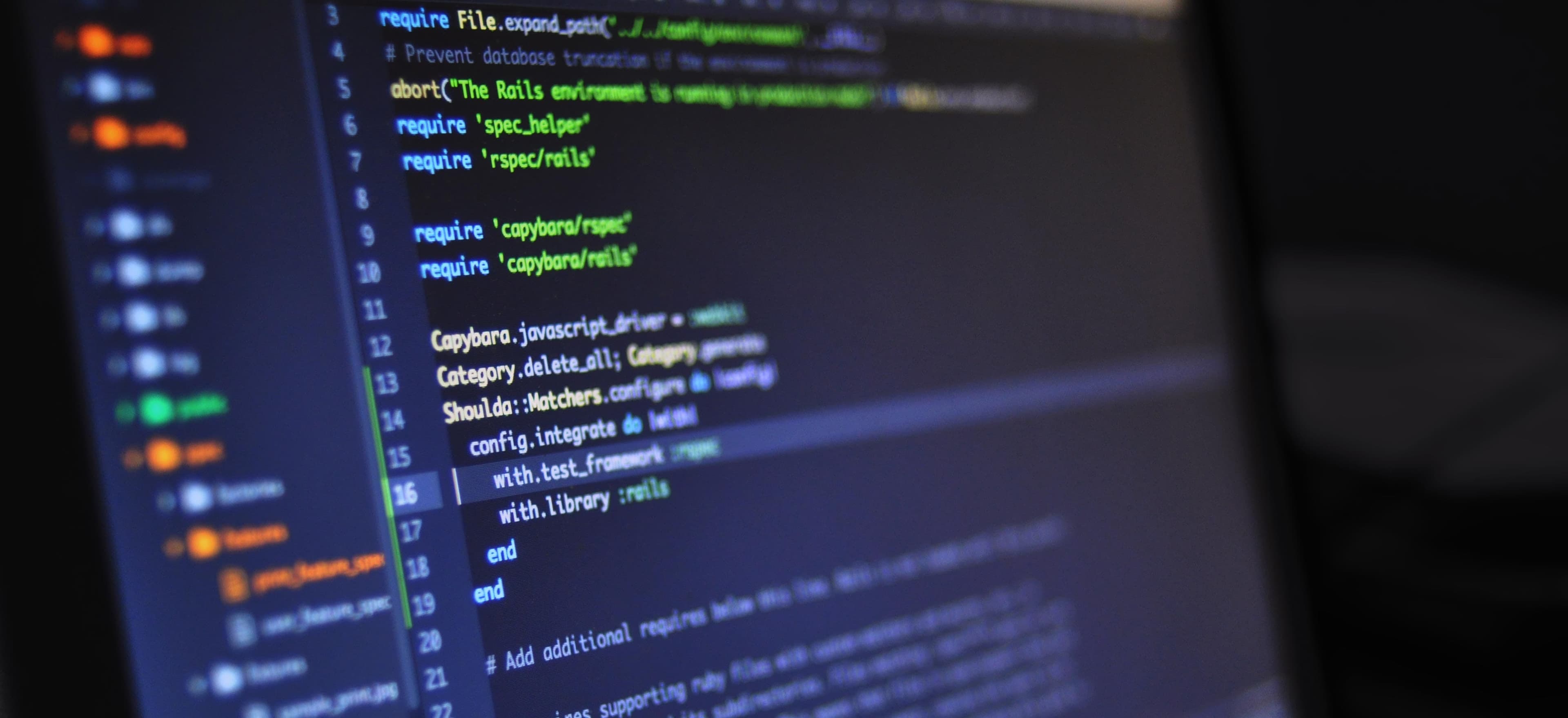
- Published on
Avoiding Duplicate Updates: Mastering REST API Resource Solutions
Do you find yourself tangled in a web of duplicate updates when working with REST APIs? You're not alone. Duplicate updates can lead to data inconsistency, unnecessary load on the server, and a frustrating user experience. In today's digital age, mastering REST API resource solutions to prevent such issues is crucial for developers. This blog post will guide you through practical strategies to avoid duplicate updates, ensuring your application runs smoothly and efficiently.
Understanding the Challenge
Before diving into solutions, let's understand why duplicate updates occur in REST APIs. These usually happen due to:
- Network Latency: Slow network connections can lead users to believe their first request didn't go through, prompting them to send another.
- User Error: A user might unintentionally click a button twice.
- Client-Side Bugs: Issues in the application logic can accidentally trigger multiple updates.
Solutions at Your Fingertips
Avoiding these pitfalls is easier than you think. Below are some effective strategies to keep your application's data integrity in check.
1. Idempotent Requests
Ensuring your HTTP methods are idempotent is key. Idempotency means that no matter how many times a request is repeated, the outcome remains the same. GET, PUT, DELETE, and PATCH can be designed to be idempotent, thus inherently avoiding the risk of duplicate updates.
// Example of a PUT request that updates a user's email
PUT /users/123/email {
"email": "newemail@example.com"
}
In this PUT request example, no matter how many times the request is made, the user's email will be updated to "newemail@example.com".
2. Use of UUIDs
Generate a unique identifier (UUID) for each operation. This UUID must be sent along with the request. The server then checks if it has already processed the request with the same UUID, and if so, it ignores the duplicate.
import java.util.UUID;
public class RequestWithUUID {
public static void main(String[] args) {
UUID requestId = UUID.randomUUID();
// Assuming this UUID is sent with the REST API request
System.out.println("Unique Request ID: " + requestId);
}
}
This Java snippet demonstrates generating a UUID for a request. Remember to store and check these UUIDs on the server side to identify and block duplicate operations.
3. Optimistic Locking
Optimistic locking allows multiple users to access a resource but stipulates that the last one to complete their operation wins. This works well for applications where conflicts are rare but managing them is crucial.
// Example of an optimistic locking strategy
int version = getCurrentVersionFromDatabase();
// Perform update operations...
if (databaseHasVersionChanged(version)) {
throw new ConcurrentModificationException("Resource was modified by someone else.");
}
You'd typically track a version
or lastModified
timestamp in your database. Before committing any updates, you check if the version has changed since you last fetched it.
4. Client-Side Solutions
Prevention is better than cure. Implementing debounce mechanisms on buttons or UI elements that trigger API calls can prevent accidental double submissions.
JavaScript example of a debounced button click event:
button.addEventListener('click', debounce(function() {
// Trigger API call
}, 250));
This ensures the API call won't be made if the button is pressed again within a 250ms window, effectively reducing the chance of duplicate requests.
5. Token-Based Strategy
A token-based strategy involves generating a unique token for every update operation and validating it server-side before processing the request. This approach is similar to using UUIDs but typically involves a one-time token generated for a particular session or transaction.
// Token generation (simplified version)
public class TokenGenerator {
public String generateToken() {
return UUID.randomUUID().toString();
}
}
// Token validation
boolean isValidToken(String token) {
// Implement token validation logic
// This could check a cache or database for token existence and validity
return true;
}
Best Practices to Follow
- Consistency is key: Choose a strategy that best fits your application's needs and apply it consistently across all your update operations.
- Monitor and log duplicate requests: This can help in identifying the root cause, be it a UX issue, network problems, or bugs in the application.
- User feedback: Provide immediate feedback to the user after they initiate an action. A simple loading indicator can prevent impatient re-clicks.
The Closing Argument
Avoiding duplicate updates in REST APIs is essential for maintaining data integrity and providing a seamless user experience. By implementing strategies like idempotent requests, using UUIDs, optimistic locking, client-side debouncing, and token-based solutions, you can significantly reduce the incidence of duplicate updates in your applications.
For further reading on REST API best practices, check out REST API Design Rulebook and explore the OpenAPI Specification for designing high-quality APIs.
As you strive for excellence in your development projects, remember that preventing duplicate updates is just one piece of the puzzle. Stay curious, keep learning, and don't hesitate to experiment with these strategies to find what works best for your specific use case.
By embracing these solutions, you're not just avoiding issues; you're building a foundation for robust, reliable, and user-friendly applications. Happy coding!