Mastering Version Control: Avoid Common pitfalls Easily
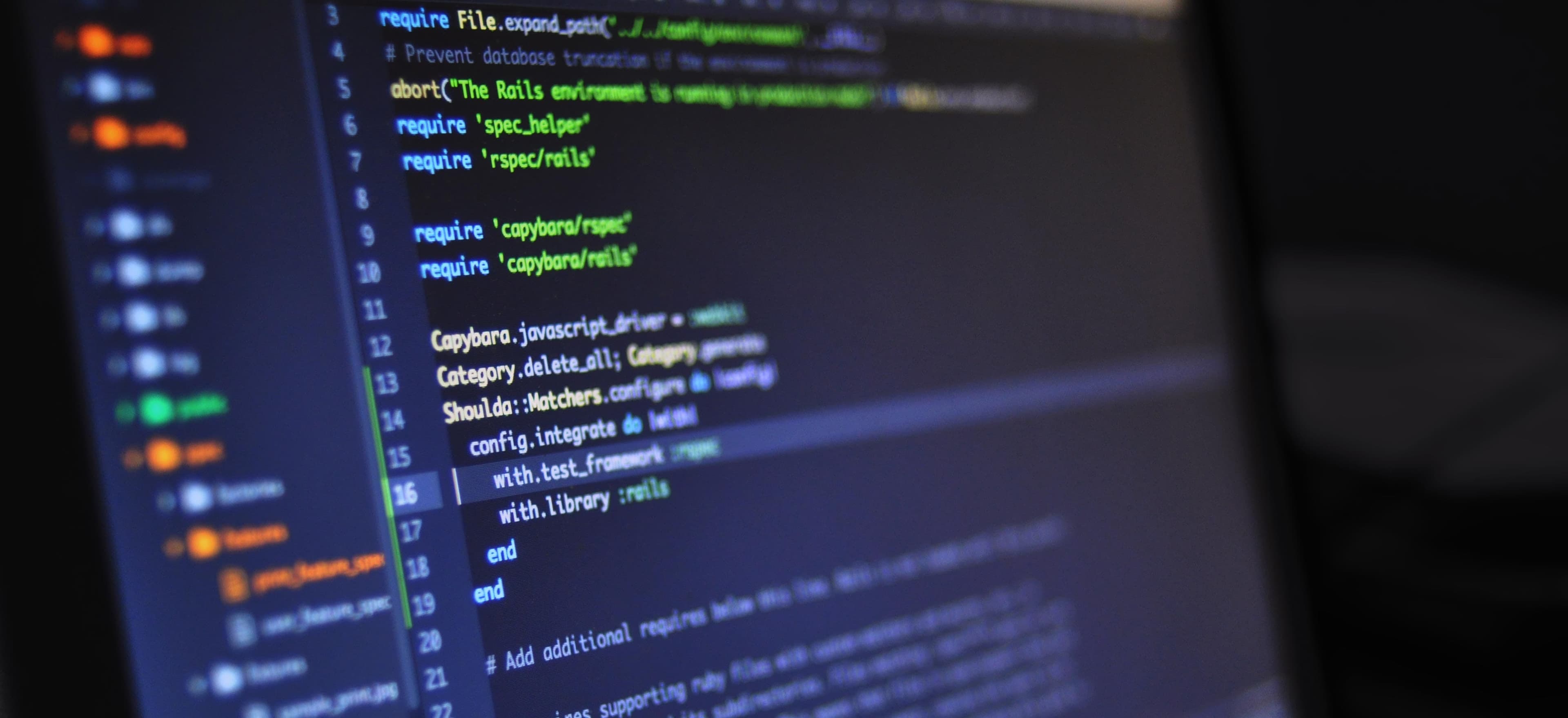
- Published on
Mastering Version Control: Avoid Common Pitfalls Easily
In software development, version control is a fundamental aspect of managing code changes and collaboration among team members. Java developers often rely on version control systems like Git to track changes, coordinate work, and maintain the integrity of their codebase. However, mastering version control requires more than just the ability to commit, push, and pull code. It involves understanding best practices, avoiding common pitfalls, and leveraging advanced features to enhance productivity and code quality.
Why Version Control Matters in Java Development
Version control systems play a crucial role in the Java development process for several reasons:
- Collaboration: It enables multiple developers to work on the same codebase simultaneously, providing a seamless environment for collaboration.
- History Tracking: Developers can track changes, revert to previous versions, and understand the evolution of the codebase over time.
- Branching and Merging: Version control allows for the creation of isolated branches for new features or bug fixes, which can later be merged back into the main codebase.
- Code Accountability: Each commit is tied to the developer who made the change, providing accountability and traceability.
While version control offers these benefits, developers often encounter common pitfalls that can hinder their productivity and code quality.
Common Version Control Pitfalls in Java Development
1. Neglecting Meaningful Commit Messages
Pitfall:
git commit -m "Fixed stuff"
Solution:
Write descriptive and meaningful commit messages that convey the purpose of the change, making it easier for team members to understand the context and history of the codebase.
2. Failing to Utilize Branches Effectively
Pitfall:
Merging code directly into the main branch without creating feature-specific or bug-fix branches.
Solution:
Embrace the use of branches to isolate changes, allowing for independent development and clear separation of concerns.
3. Ignoring Code Review and Pull Requests
Pitfall:
Pushing code directly without seeking review from peers, leading to potential issues and bugs in the codebase.
Solution:
Leverage pull requests and code reviews to solicit feedback, catch potential errors, and ensure code quality before merging changes into the main branch.
4. Overlooking .gitignore Best Practices
Pitfall:
Including sensitive files, build artifacts, or IDE-specific configurations in the repository, leading to bloated repositories and security risks.
Solution:
Adhere to .gitignore best practices to exclude unnecessary files and directories from version control, maintaining a clean and efficient repository.
How to Avoid Version Control Pitfalls in Java Development
Mastering version control in Java development involves adopting best practices and leveraging advanced features to mitigate common pitfalls effectively.
1. Write Meaningful Commit Messages
When making a commit, provide a clear and concise summary of the changes and explain the reasoning behind the modification. A well-crafted commit message serves as a valuable communication tool for team members, enabling them to understand the purpose of the change without scrutinizing the code diff extensively.
Example of a meaningful commit message:
git commit -m "Refactor service layer to improve code readability and maintainability"
2. Embrace Branching Strategies
Utilize branching effectively to isolate changes and support parallel development. Adopt a branching strategy such as Git Flow or GitHub Flow, which promotes the creation of feature branches for new functionality and hotfix branches for addressing critical issues. This approach ensures a structured and manageable workflow, preventing conflicts and chaos within the codebase.
Example of creating and switching to a new branch:
git checkout -b feature/new-feature
3. Leverage Pull Requests and Code Reviews
Before merging code into the main branch, initiate a pull request to solicit feedback from team members. Code reviews provide an opportunity to validate the quality of the changes, identify potential issues, and improve the overall codebase through collaboration. Emphasize the importance of thorough reviews to maintain code integrity and prevent regressions.
Example of creating a pull request using GitHub:
hub pull-request -m "Feature: Implement user authentication"
4. Implement .gitignore Best Practices
Ensure that your Java project repositories exclude irrelevant files and directories by utilizing a well-structured .gitignore file. Exclude build artifacts, temporary files, IDE-specific configurations, and sensitive information to streamline the repository and minimize the risk of inadvertently committing confidential data.
Example of .gitignore for a Java project:
# Java build artifacts
target/
*.class
# IDE-specific configurations
/.idea
*.iml
Advanced Version Control Techniques for Java Developers
Beyond the basics, Java developers can explore advanced version control techniques to further enhance their workflow and code management.
1. Git Rebase for Cleaner Commit History
Integrate the use of git rebase to maintain a clean and linear commit history. Rebase allows developers to reapply their changes on top of the latest codebase, resulting in a more organized and readable commit log compared to traditional merge commits.
2. Git Hooks for Automated Workflows
Implement custom git hooks to automate tasks such as code formatting, linting, or running tests before accepting commits. Git hooks enable developers to enforce project-specific workflows and maintain consistent code quality throughout the repository.
3. Version Control Integration with IDEs
Explore seamless integration between version control systems and Java IDEs such as IntelliJ IDEA or Eclipse. Leverage IDE features to visualize code changes, resolve merge conflicts, and interact with the version control system directly within the development environment.
The Closing Argument
Mastering version control is essential for Java developers to navigate the complexities of code management, collaboration, and productivity. By recognizing common version control pitfalls and adopting best practices, developers can ensure a streamlined and efficient workflow while maintaining the integrity of their codebase. Embracing advanced version control techniques further empowers developers to optimize their development process and elevate the quality of their Java projects.
Remember, version control is not just about tracking changes; it's about fostering a culture of collaboration, accountability, and continuous improvement within the development team.
By understanding the nuances of version control and its impact on Java development, developers can elevate their proficiency and conquer the challenges of code management with confidence and ease.
So, start mastering version control today and unlock the full potential of your Java development endeavors!
The blog post provides actionable insights to navigate version control pitfalls in Java development and offers advanced techniques to optimize the workflow. By emphasizing best practices and real-world examples, it equips Java developers with the knowledge to tackle version control challenges effectively. Additionally, the post encourages continuous improvement and emphasizes the significance of version control beyond mere code tracking. For further context, readers can explore additional resources on branching strategies and Git workflows.