Control Your Sphero with Android: The Heat Is On!
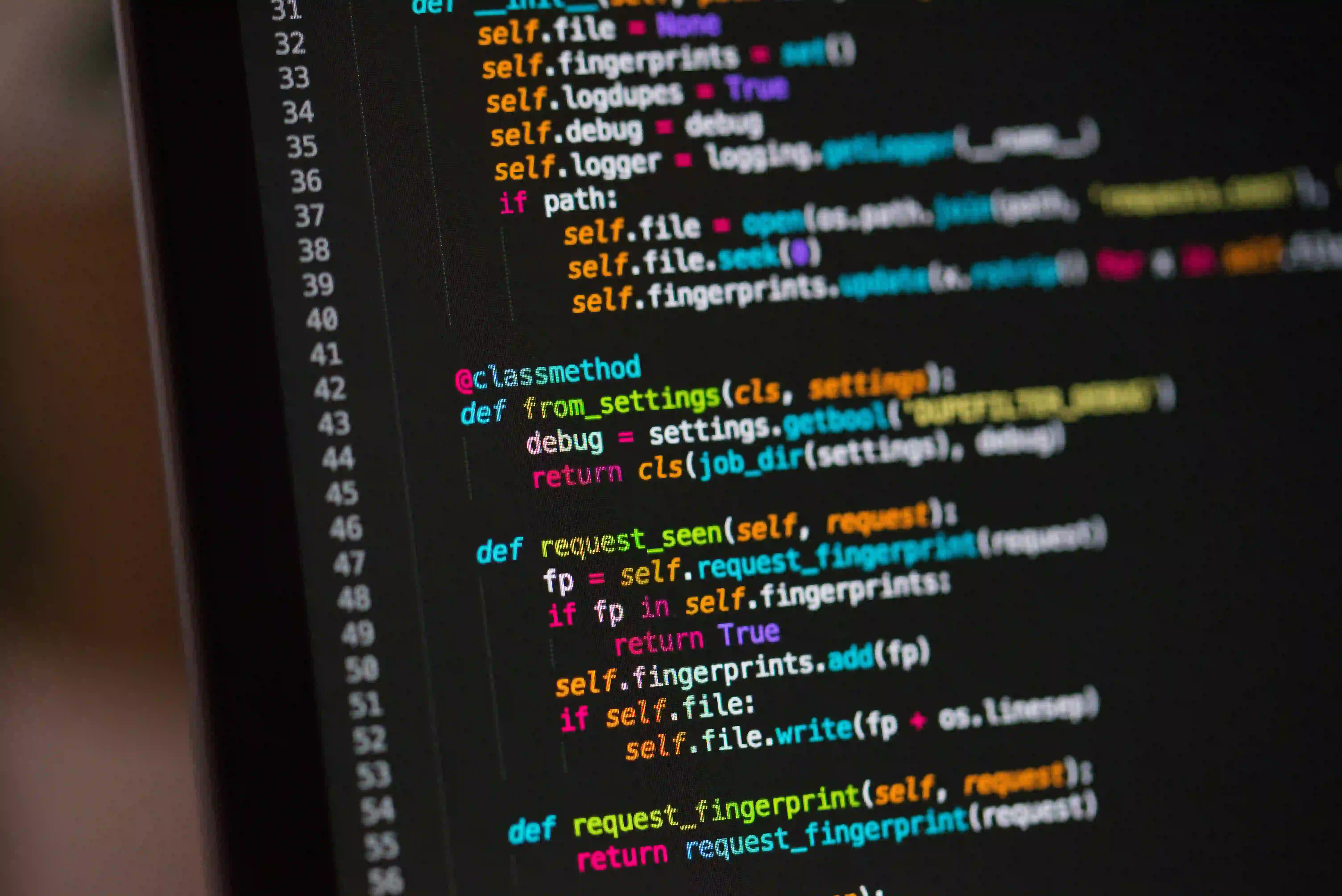
Controlling Your Sphero with Android: The Heat Is On!
Are you ready to take your Android programming to the next level and add some excitement to your mobile applications? Look no further than controlling a Sphero robot with your Android device. Sphero is a robotic ball that you can control using various commands sent from your Android smartphone or tablet. In this guide, we will walk through how to connect to a Sphero robot and send commands to make it move, change colors, and perform other cool tricks.
Getting Started
Before we dive into the code, it's essential to ensure you have the necessary tools to work with Sphero and Android. You will need:
- A Sphero robot
- An Android device with Bluetooth capabilities
- The Sphero SDK for Android
The Sphero SDK for Android provides a set of libraries and code samples to help you communicate with the Sphero robot from your Android app. You can download the SDK from the official Sphero Developer portal.
Connecting to Sphero
To start controlling the Sphero, you need to establish a Bluetooth connection between your Android device and the robot. The following code demonstrates how to discover and connect to the Sphero:
BluetoothAdapter bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
BluetoothDevice spheroDevice = null;
Set<BluetoothDevice> pairedDevices = bluetoothAdapter.getBondedDevices();
for (BluetoothDevice device : pairedDevices) {
if (device.getName().startsWith("Sphero")) {
spheroDevice = device;
break;
}
}
if (spheroDevice != null) {
// Connect to Sphero
// ...
} else {
// Sphero not found
// ...
}
In this code, we first obtain the default Bluetooth adapter on the Android device. We then iterate through the list of paired devices and look for the one with the name starting with "Sphero". Once we find the Sphero device, we can proceed to establish a connection.
Sending Commands
Once the connection is established, you can start sending commands to control the Sphero. The Sphero SDK provides methods to send commands such as roll, set color, and even execute pre-defined animations. Here's a simple example of how to make the Sphero roll in a specific direction:
Sphero sphero = new Sphero(spheroDevice); // Instantiate the Sphero object
sphero.connect(); // Connect to the Sphero
// Roll Sphero in a specific direction
sphero.roll(50, 45); // Speed: 50, Heading: 45 degrees
In this example, we create a new Sphero
object and establish a connection. Then, we use the roll
method to make the Sphero roll at a speed of 50 in the direction of 45 degrees. This would cause the Sphero to move forward at the given speed and direction.
Changing Colors
Controlling the Sphero isn't limited to just movement. You can also change the color of the Sphero's LED lights to create visually appealing effects. The following code snippet demonstrates how to change the Sphero's color:
sphero.setRGBLED(255, 0, 0); // Set the LED color to red
In this example, we use the setRGBLED
method to set the Sphero's LED color to red. The method takes three arguments representing the values of red, green, and blue channels, allowing you to create a wide range of colors.
Listening to Sensor Data
The Sphero robot comes equipped with various sensors, including an accelerometer and a gyroscope. You can leverage these sensors to create interactive experiences by responding to the Sphero's movements. The following code illustrates how to listen to the Sphero's accelerometer data:
sphero.addSensorListener(new SensorListener() {
@Override
public void onAccelerometerData(Sphero sphero, final long interval, final float x, final float y, final float z) {
// React to accelerometer data
// ...
}
});
In this code, we add a SensorListener
to the Sphero object, allowing us to react to accelerometer data. When the Sphero detects acceleration, the onAccelerometerData
method is called, providing the interval and the values of acceleration along the x, y, and z axes.
Lessons Learned
With the Sphero SDK for Android, you can unlock a world of possibilities for creating engaging and interactive applications. Whether you want to build a remote-controlled robot, a dynamic gaming experience, or a colorful light show, the Sphero provides an exciting platform for your creativity to shine.
In this guide, we covered the basics of connecting to a Sphero robot, sending commands to control its movements and LED colors, and listening to sensor data. Armed with this knowledge, you can start building your own Sphero-powered Android apps and delight users with immersive experiences.
Now that you have a taste of controlling a Sphero with Android, grab your robot and start coding to bring your ideas to life!
For more information, you can refer to the official Sphero SDK for Android documentation. Happy coding!