Shrink Memory Footprint: Mastering Compressed OOPs in Java
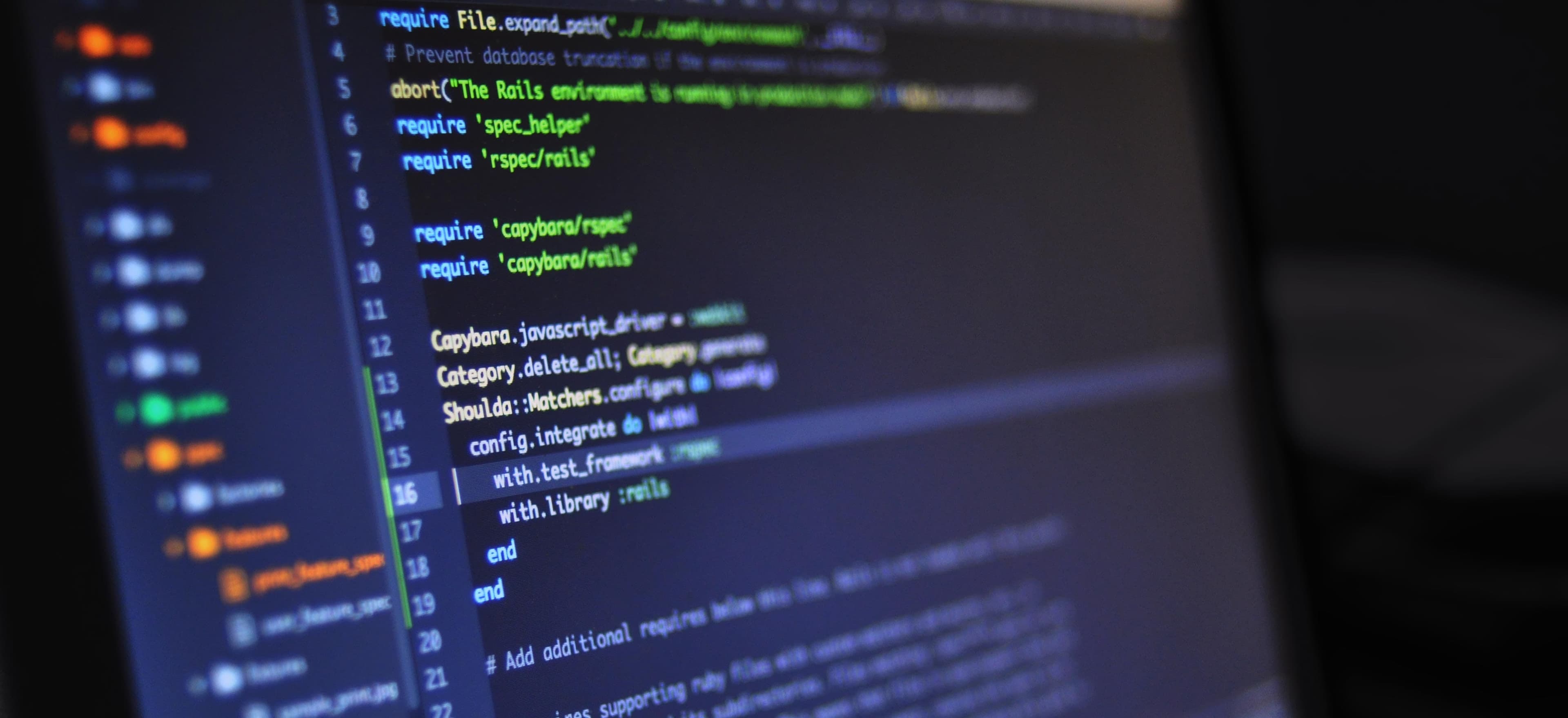
- Published on
Shrink Memory Footprint: Mastering Compressed OOPs in Java
In the world of programming, memory management and optimization play a crucial role in determining the performance of an application. In Java, the memory footprint of objects is a critical factor that directly impacts the overall efficiency and scalability of the program. It becomes increasingly important when dealing with large-scale applications and big data processing.
Understanding Compressed OOPs
Java employs the use of Ordinary Object Pointers (OOPs) for referencing and manipulating objects in memory. These pointers are typically 32 or 64 bits long, depending on the underlying architecture. In most cases, the memory address space provided by 32-bit pointers is more than sufficient for addressing the entire heap space. However, in 64-bit systems, the memory footprint of these pointers becomes a significant overhead, especially when dealing with a large number of objects.
To address this issue, Java offers an optimization technique known as Compressed OOPs, which allows the JVM to use 32-bit references for heap sizes less than or equal to 32 GB, thus reducing the memory overhead associated with 64-bit pointers while still providing access to a large heap.
Enabling Compressed OOPs
By default, Compressed OOPs is enabled in Java 8 and later versions for 64-bit JVMs when the maximum heap size (-Xmx) is set to 32 GB or less. However, it's essential to ensure that the JVM is configured to utilize this optimization, especially when working with memory-constrained environments or when running on older versions of Java.
To explicitly enable Compressed OOPs, the following JVM parameter can be used:
-XX:+UseCompressedOops
By including this parameter in the JVM arguments, you instruct the JVM to utilize Compressed OOPs, thereby reducing the memory footprint of object references. This simple yet powerful optimization can lead to significant improvements in memory utilization and overall performance.
Benefits of Compressed OOPs
The use of Compressed OOPs brings several notable benefits to Java applications, including:
-
Reduced Memory Overhead: By using 32-bit references for heap sizes up to 32 GB, the memory overhead associated with 64-bit pointers is minimized, leading to more efficient memory utilization.
-
Improved Cache Performance: Smaller object references result in better cache utilization, as more references can fit into the CPU cache, reducing the number of cache misses and improving overall performance.
-
Enhanced Scalability: With reduced memory footprint, applications can scale better, especially in memory-constrained environments where efficient memory management is crucial.
Understanding the Limitations
While Compressed OOPs offer significant advantages, it's essential to be cognizant of their limitations. One key limitation is that when the heap size exceeds 32 GB, Java reverts to using 64-bit references, potentially increasing the memory overhead. Therefore, for applications requiring a larger heap, alternative memory management strategies should be considered.
Additionally, not all object types benefit equally from Compressed OOPs. Objects larger than the available 32-bit address space still require 64-bit references, mitigating the optimization's impact on large objects.
Best Practices for Leveraging Compressed OOPs
When leveraging Compressed OOPs in Java applications, several best practices can be followed to maximize the benefits of this optimization:
-
Right-size Your Heap: By carefully analyzing the memory requirements of your application, you can ensure that the heap size remains within the optimal range for Compressed OOPs to be effective. This not only reduces memory overhead but also contributes to better overall performance.
-
Optimize Object Sizes: Since objects larger than the 32-bit address space do not benefit from Compressed OOPs, it's beneficial to optimize the sizes of objects where possible. This includes minimizing unnecessary padding and reducing object header overhead.
-
Regularly Monitor Memory Usage: Continuous monitoring of memory usage and heap behavior can aid in identifying opportunities for optimizing memory utilization. Tools such as Java Mission Control and VisualVM can provide insightful data for performance analysis and tuning.
To Wrap Things Up
Compressed OOPs in Java offer a powerful means of reducing memory overhead and improving the overall efficiency of applications, especially in memory-constrained environments. By understanding the principles behind Compressed OOPs and following best practices for its utilization, developers can significantly enhance the performance and scalability of their Java applications while minimizing the impact of memory management on system resources.
As Java continues to evolve, advancements in memory optimization techniques and JVM enhancements will further contribute to the efficiency and effectiveness of Compressed OOPs, solidifying its role as a critical component in the realm of Java performance tuning and memory management.
In conclusion, mastering Compressed OOPs is not just an optimization technique; it's a fundamental aspect of building robust and high-performing Java applications in modern computing environments.
For more insights on Java memory optimization, take a look at this in-depth analysis of memory optimization techniques in Java.
To delve deeper into JVM tuning, explore this comprehensive guide on JVM performance tuning.