Crafting Thread-Safe ConcurrentHashSet in Java 8: A Guide
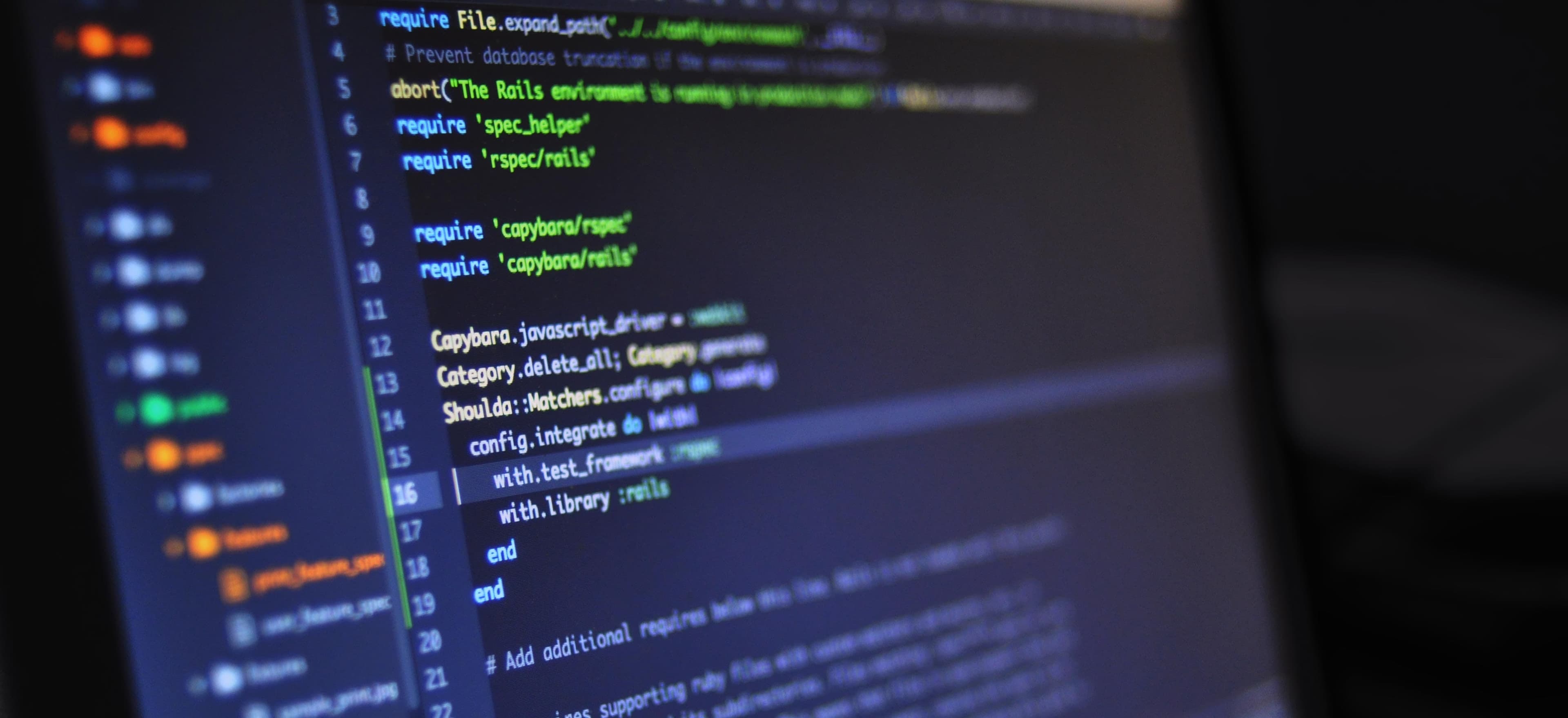
- Published on
Crafting Thread-Safe ConcurrentHashSet in Java 8: A Guide
When it comes to working with concurrent data structures in Java, the ConcurrentHashMap
is often the first one that comes to mind. However, what if we need a concurrent set that carries out operations such as add
, remove
, and contains
in a thread-safe manner?
In this guide, we'll explore how to craft a thread-safe ConcurrentHashSet
in Java 8, allowing us to safely manipulate a set in a multi-threaded environment.
Understanding the Need for a ConcurrentHashSet
Java provides a standard HashSet
implementation, which is not thread-safe. When multiple threads attempt to modify the set concurrently, the behavior is undefined, and the set may become corrupted.
To address this issue, we require a data structure that provides thread-safe operations for adding, removing, and checking existence of elements.
Leveraging ConcurrentHashMap for Thread Safety
The key to crafting a thread-safe ConcurrentHashSet
lies in leveraging the ConcurrentHashMap
. We can harness the powerful concurrency utilities provided by ConcurrentHashMap
to create a thread-safe set.
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashSet<E> {
private final ConcurrentHashMap<E, Boolean> map;
public ConcurrentHashSet() {
this.map = new ConcurrentHashMap<>();
}
public boolean add(E element) {
return map.putIfAbsent(element, Boolean.TRUE) == null;
}
public boolean remove(E element) {
return map.remove(element) != null;
}
public boolean contains(E element) {
return map.containsKey(element);
}
public Set<E> getConcurrentHashSet() {
return map.keySet();
}
}
In the above implementation, we use a ConcurrentHashMap
with a Boolean value to simulate a set, where the elements are keys in the map and the Boolean value is insignificant.
The add
, remove
, and contains
operations are simply delegated to the corresponding methods of the underlying ConcurrentHashMap
, which provides thread-safe implementations for these operations.
The 'Why' Behind the Implementation
Leveraging putIfAbsent for Atomicity
The putIfAbsent
method of ConcurrentHashMap
adds the specified key-value pair if it is not already present. This method is atomic - meaning it ensures that only one thread can successfully add the element at a time, preventing race conditions and ensuring thread safety.
Utilizing ConcurrentHashMap's Thread-Safe Operations
The remove
and containsKey
operations of ConcurrentHashMap
are inherently thread-safe. By delegating these operations, we inherit thread safety from the underlying map.
Exposing the Set with getConcurrentHashSet
The getConcurrentHashSet
method allows safe access to the underlying set by returning a thread-safe view of the keys present in the ConcurrentHashMap
. This prevents direct mutation of the set and ensures overall thread safety.
Using the ConcurrentHashSet in Practice
Let's see how we can use the ConcurrentHashSet
in a multi-threaded environment:
ConcurrentHashSet<Integer> concurrentHashSet = new ConcurrentHashSet<>();
ExecutorService executorService = Executors.newFixedThreadPool(2);
executorService.submit(() -> {
concurrentHashSet.add(1);
concurrentHashSet.add(2);
});
executorService.submit(() -> {
concurrentHashSet.remove(2);
System.out.println(concurrentHashSet.contains(1)); // true
System.out.println(concurrentHashSet.contains(2)); // false
});
executorService.shutdown();
In the example above, we create a ConcurrentHashSet
and use two threads to concurrently add and remove elements from it. The ConcurrentHashSet
safely handles the concurrent operations without any risk of data corruption or inconsistency.
Final Thoughts
In this guide, we've delved into the need for a thread-safe set in Java and crafted a ConcurrentHashSet
using the powerful ConcurrentHashMap
. By delegating operations to the underlying ConcurrentHashMap
, we've ensured thread safety for adding, removing, and checking existence of elements in our set.
By wielding the ConcurrentHashSet
, we can confidently manipulate sets in multi-threaded scenarios, without fearing data inconsistencies or race conditions.
In your Java projects, considering the thread safety of your data structures is crucial, and the ConcurrentHashSet
provides a robust solution for concurrent set manipulation.
With Java's rich concurrency utilities, we can craft thread-safe data structures that elegantly handle multi-threaded operations, ensuring the integrity of our data in concurrent environments.
So, next time you find yourself in need of a thread-safe set in Java, consider crafting your own ConcurrentHashSet
and pave the way for safe and seamless multi-threaded set operations.
Happy coding!
To deepen your understanding of Java concurrency, consider exploring the Java documentation about ConcurrentHashMap and the Concurrency Utilities.
This guide was brought to you by JavaGenius, your go-to resource for all things Java.