Mastering OSGi Tests: Solving ServiceCollector Challenges
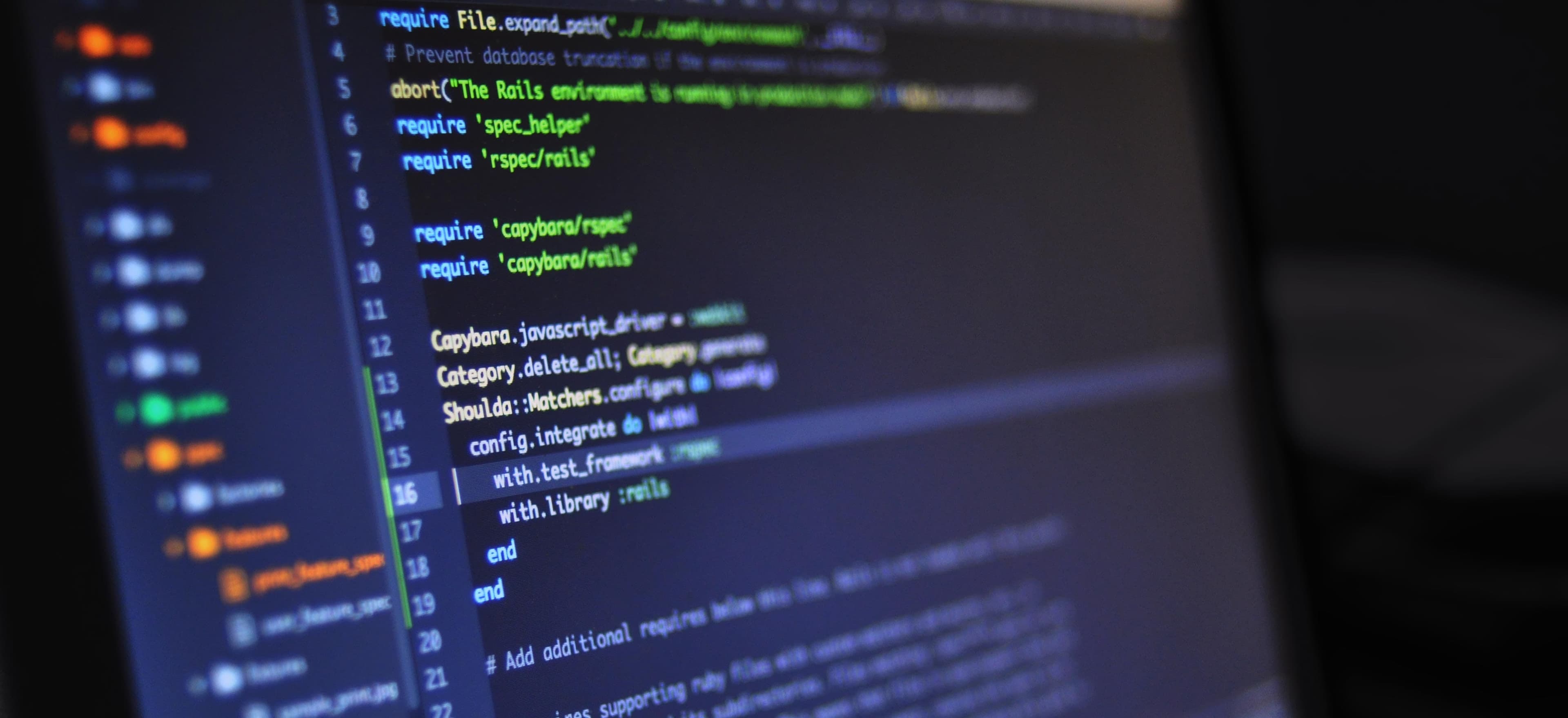
- Published on
Mastering OSGi Tests: Solving ServiceCollector Challenges
In the world of Java development, OSGi (Open Service Gateway initiative) has gained significant momentum as a modular framework for creating sophisticated applications. OSGi provides a dynamic module system for Java that simplifies the architecture and development process. However, one of the primary challenges developers face is testing OSGi applications effectively.
In this blog post, we will focus on one specific challenge that developers often encounter when working with OSGi: testing components that rely on dynamic OSGi services. We will explore how to overcome these challenges using a ServiceCollector approach and delve into writing effective tests for OSGi applications.
Understanding the Challenge
In OSGi-based applications, components often rely on OSGi services, making them dynamic and difficult to test in isolation. Typically, traditional testing frameworks struggle to handle the dynamic nature of OSGi services, which can lead to cumbersome and fragile tests.
To illustrate this challenge, let's consider a hypothetical scenario where we have a component that depends on an OSGi service. Traditional testing approaches using mock objects or stubs may not be sufficient, as the service dependency is dynamic and might not be available during the test execution.
public class MyComponent {
private MyService myService;
public void activate() {
myService = getService(MyService.class);
// perform initialization logic
}
public void doSomething() {
// interact with myService
}
}
Solving the Challenge with ServiceCollector
To address the testing challenges associated with OSGi services, we can employ the ServiceCollector pattern. The ServiceCollector pattern involves collecting OSGi services during the test setup to ensure that the required services are available for testing.
Let's take a look at how we can implement the ServiceCollector pattern to effectively test the MyComponent
class introduced earlier.
public class MyComponentTest {
private MyComponent myComponent;
private ServiceCollector<MyService> serviceCollector;
@Before
public void setUp() {
BundleContext bundleContext = mock(BundleContext.class);
serviceCollector = new ServiceCollector<>(bundleContext, MyService.class);
myComponent = new MyComponent();
myComponent.activate();
}
@Test
public void testDoSomething() {
// Arrange
MyService myService = mock(MyService.class);
serviceCollector.addService(myService);
// Act
myComponent.doSomething();
// Assert
// Add assertions here
}
}
In the test setup, we create a ServiceCollector
instance, passing the BundleContext
and the service interface as parameters. By doing so, we ensure that the required OSGi services are collected and made available for testing purposes.
During the test execution, we use the addService
method of the ServiceCollector
to add a mock implementation of the required service. This allows us to simulate the dynamic nature of OSGi services and test the MyComponent
class in isolation.
Embracing OSGi Testing Best Practices
In addition to the ServiceCollector pattern, there are several best practices that can significantly enhance the testing of OSGi-based applications:
Integration Testing with OSGi Containers
When testing OSGi applications, integration testing with OSGi containers such as Apache Felix or Eclipse Equinox can provide a more realistic environment for verifying the behavior of OSGi components and services. Integration testing allows for testing the actual interaction between OSGi bundles and services within a controlled environment.
public class MyIntegrationTest {
@Test
public void testComponentIntegration() {
// Create an OSGi container (e.g., Apache Felix)
// Deploy required bundles and services
// Interact with and verify component behavior
}
}
Mocking Frameworks with OSGi Support
Utilizing mocking frameworks that offer OSGi support, such as Mockito, can streamline the testing process for OSGi applications. These frameworks provide extensions or features specifically designed to facilitate working with OSGi services and bundles in test scenarios.
public class MyMockitoTest {
@Test
public void testComponentWithMockito() {
MyService myService = mock(MyService.class);
when(myService.performOperation()).thenReturn("MockedResult");
// Inject mocked service into component and verify behavior
}
}
OSGi Test Harness Libraries
Leveraging OSGi test harness libraries like Pax Exam can simplify the process of writing comprehensive OSGi integration tests. These libraries offer utilities and APIs tailored for testing OSGi-based applications, enabling developers to create well-structured and maintainable test suites.
@RunWith(JUnit4TestRunner.class)
@ExamReactorStrategy(PerClass.class)
public class MyPaxExamTest {
@Inject
MyService myService;
@Configuration
public Option[] config() {
return options(
// Configure OSGi bundles and services for testing
);
}
@Test
public void testComponentBehavior() {
// Verify component behavior within an OSGi environment
}
}
Continuous Integration with OSGi Builds
Integrating OSGi test suites into continuous integration (CI) pipelines using tools like Jenkins or Travis CI ensures that OSGi applications are regularly tested in a controlled environment. This practice helps detect potential issues and regressions early in the development lifecycle.
My Closing Thoughts on the Matter
Effectively testing OSGi-based applications requires a strategic approach that accounts for the dynamic nature of OSGi services. By embracing patterns like ServiceCollector and integrating best practices such as OSGi container testing and leveraging OSGi-specific testing libraries and tools, developers can ensure the reliability and quality of their OSGi applications.
Remember, mastering OSGi tests is not just about writing tests, but also about understanding the unique challenges posed by OSGi's dynamic nature and architecting test strategies that address these challenges effectively.
With the right approach and tools at your disposal, conquering the testing challenges of OSGi is well within reach. So, go ahead, embrace the dynamic world of OSGi, and master the art of testing OSGi applications with confidence!
To delve deeper into OSGi testing and best practices, refer to the official OSGi Alliance website and the Apache Felix project.
Happy testing!