Unraveling Mockito: Mastering Matchers Precedence!
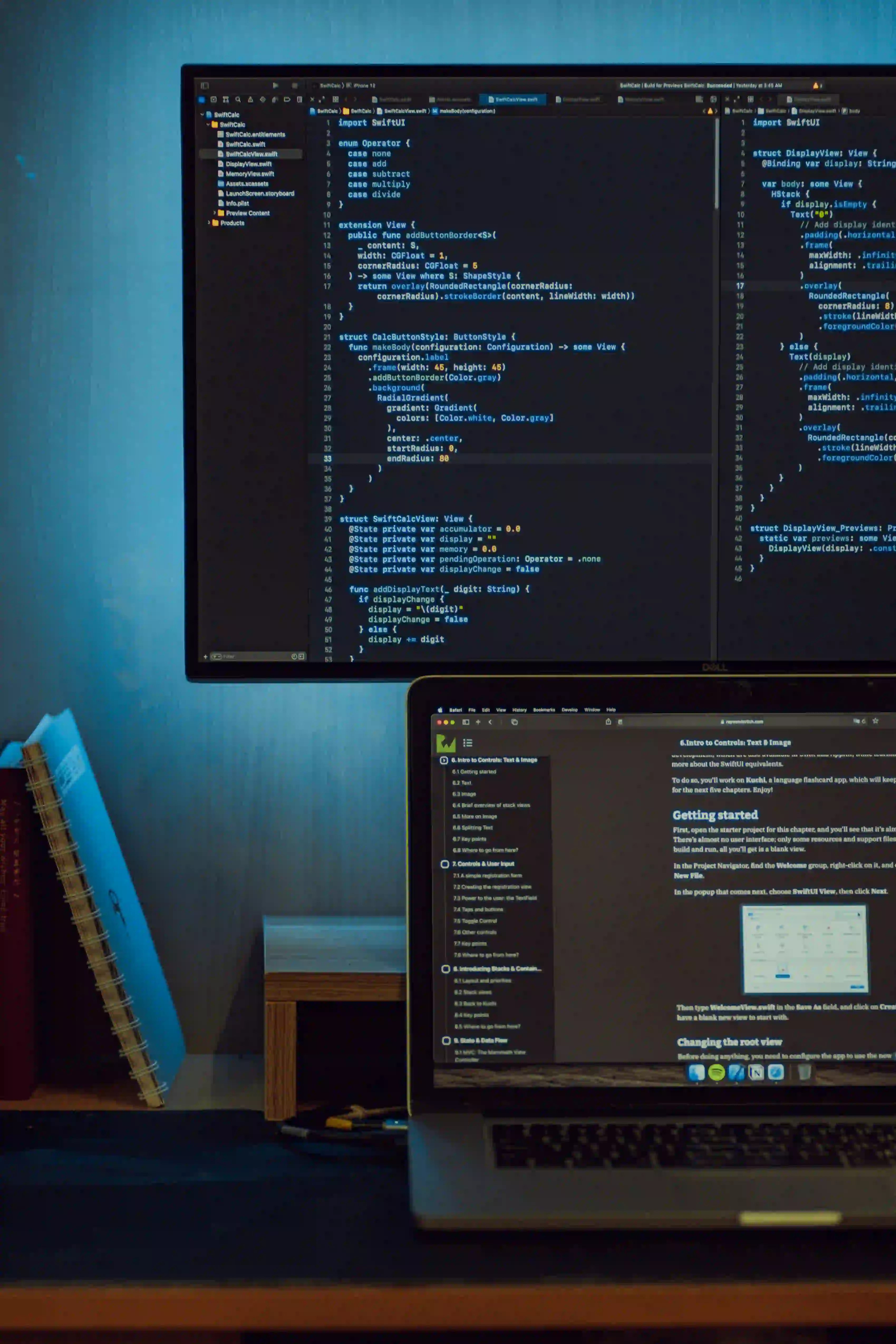
Unraveling Mockito: Mastering Matchers Precedence
Mockito is a popular open-source mocking framework for Java. It allows the creation of mock objects in automated unit tests for the purpose of test-driven development. One of the key aspects of Mockito is its support for matchers, which enable flexible and expressive verification of method calls on mock objects.
In this post, we will delve into the world of Mockito matchers, focusing specifically on understanding and mastering matchers precedence. By the end, you will have a firm grasp of how to effectively use matchers in your Mockito tests.
Understanding Matchers in Mockito
Matchers in Mockito are used to specify the arguments for method calls on mock objects. They allow you to define flexible matching rules for method invocations. This flexibility is essential for verifying behavior in unit tests, especially when dealing with complex interactions between objects.
Let's take a quick look at some common built-in matchers provided by Mockito:
anyInt()
,anyLong()
,anyDouble()
: Matches any integer, long, or double value, respectively.eq(value)
: Matches the specific value provided as an argument.any(Class<T> type)
: Matches any object of the specified type.isNull()
,notNull()
: Matches null or non-null values, respectively.
Matchers Precedence in Mockito
One of the important concepts to understand when working with matchers in Mockito is matcher precedence. Matcher precedence determines the order in which matchers are evaluated when verifying method invocations on mock objects.
The Problem
Consider the following scenario where we have a Calculator
interface with a divide
method that takes two arguments:
public interface Calculator {
double divide(int dividend, int divisor);
}
Now, let's create a mock Calculator
object and verify its divide
method using Mockito matchers:
Calculator calculator = mock(Calculator.class);
verify(calculator).divide(anyInt(), anyInt());
In this scenario, the anyInt()
matcher is used for both arguments. However, if we want to specify a specific value for one of the arguments, we encounter a problem with matcher precedence.
Matcher Precedence Rules
Matcher precedence follows the order in which the matchers are defined in the argument list. The rule of thumb is that more specific matchers should be defined first, followed by more general matchers.
Let's illustrate this with an example. Consider the following code:
verify(calculator).divide(eq(10), anyInt());
In this case, eq(10)
is a specific matcher, while anyInt()
is a more general matcher. According to the precedence rules, the eq(10)
matcher takes precedence over anyInt()
. This is because Mockito evaluates the matchers from left to right, and the first specific matcher it encounters takes precedence.
Solving the Precedence Issue
To solve the precedence issue, we need to define the matchers in the correct order. In the previous example, we can swap the order of the matchers to ensure that eq(10)
takes precedence:
verify(calculator).divide(anyInt(), eq(10));
By swapping the order, we ensure that the specific matcher eq(10)
is evaluated first, followed by the more general matcher anyInt()
.
The Last Word
Mastering matchers precedence in Mockito is crucial for writing effective and reliable unit tests. Understanding the order in which matchers are evaluated allows you to express complex verification logic and ensure the accuracy of your tests.
By following the rules of matcher precedence and arranging matchers in the correct order, you can wield the full power of Mockito matchers to create expressive and precise verifications in your unit tests.
To further enhance your understanding of Mockito matchers and their precedence, consider exploring the official Mockito documentation: Mockito Matchers.
In conclusion, the mastery of matchers precedence in Mockito is an essential skill for any Java developer looking to write robust and reliable unit tests. With this knowledge in your toolbox, you can elevate your unit testing practices and ensure the integrity of your codebase.
Now, armed with the knowledge of matchers precedence in Mockito, go forth and write impeccable unit tests with confidence!