Solving Maven Dependency Hells: A Step-by-Step Guide
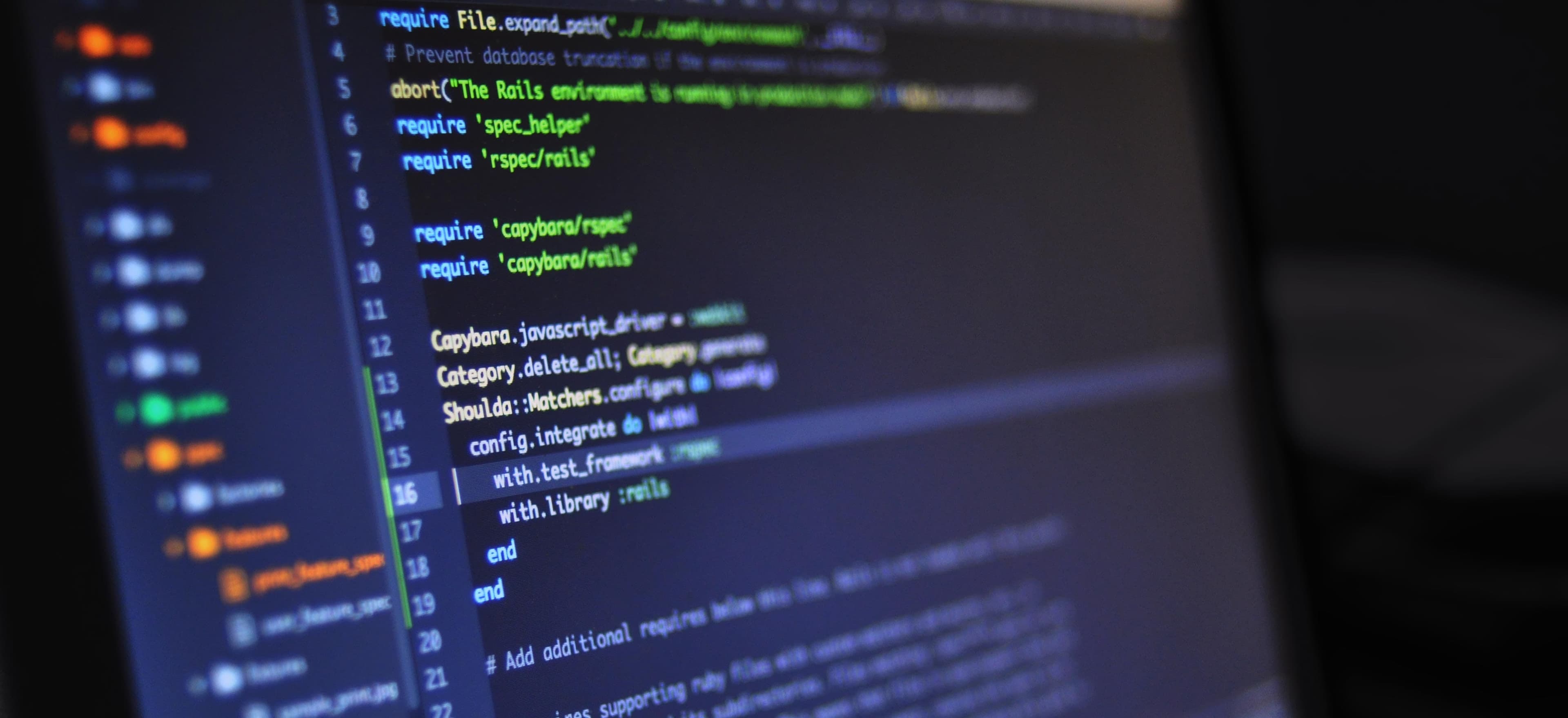
- Published on
Solving Maven Dependency Hells: A Step-by-Step Guide
Maven is a powerful build automation tool predominantly used for Java projects. One of its most useful features is its ability to manage project dependencies through its Project Object Model (POM) file. However, managing dependencies can become challenging when dealing with transitive dependencies, conflicting versions, and dependency hells. In this guide, we'll explore some common Maven dependency issues and provide step-by-step solutions.
Understanding Maven Dependencies
In a Maven project, dependencies are managed through the POM file. When a project relies on external libraries or other projects, Maven automatically downloads the necessary JAR files and adds them to the classpath. Maven also resolves transitive dependencies, which are dependencies of dependencies.
Identifying Dependency Issues
Conflicting Versions
One common issue is conflicting versions of the same dependency. This can arise when two different dependencies require different versions of the same library.
Missing Dependencies
Sometimes, a project may have missing dependencies that are not explicitly declared in the POM file. This can lead to runtime errors or unexpected behavior.
Overlapping Versions
Another issue is when different dependencies rely on the same transitive dependency but require different versions. Maven needs to resolve which version to use, which can lead to unexpected behavior.
Steps to Solve Maven Dependency Hells
Analyze Dependency Tree
Maven provides a helpful command to visualize the project's dependency tree. Use the following command to generate a graphical representation of the dependencies:
mvn dependency:tree
The output will display the complete dependency tree, including transitive dependencies. Analyzing this tree can help identify conflicting versions or missing dependencies.
Exclude Transitive Dependencies
In some cases, it's necessary to exclude certain transitive dependencies to resolve conflicts. Use the <exclusions>
tag within the dependency declaration to exclude specific transitive dependencies. For example:
<dependency>
<groupId>com.example</groupId>
<artifactId>example-library</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>org.unwanted</groupId>
<artifactId>unwanted-library</artifactId>
</exclusion>
</exclusions>
</dependency>
By excluding unwanted libraries, you can ensure that only the required dependencies are included in the project.
Dependency Management
Maven allows for centralized management of dependencies through the <dependencyManagement>
section in the POM file. This section can be used to define versions of dependencies and then reference them in individual dependencies.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>common-library</artifactId>
<version>2.0.0</version>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>specific-library</artifactId>
<version>1.0.0</version>
</dependency>
<!-- Other dependencies -->
</dependencies>
By centralizing version management, it is easier to ensure consistency across the project.
Use Dependency Scope Wisely
Maven provides various dependency scopes such as compile, runtime, provided, and test. These scopes control when dependencies are available during the build lifecycle. It's important to use the appropriate scope to prevent unnecessary dependencies from being included in the final artifact.
Dependency Exclusion
If a transitive dependency is causing issues, it can be explicitly excluded using the <exclusion>
tag within the dependency declaration. This allows for granular control over which transitive dependencies are included in the project.
Update Dependency Versions
Frequently, new versions of dependencies are released to address issues and introduce new features. It's essential to regularly check for updates to dependencies and incorporate them into your project to benefit from bug fixes and performance improvements.
Use Bill of Materials (BOM)
In cases where many dependencies are used from the same vendor, a BOM can be leveraged to manage versions of all those dependencies in one place. Using a BOM can significantly simplify the management of versions and prevent version mismatch issues.
The Last Word
Managing dependencies in a Maven project can be a complex task, especially when dealing with transitive dependencies and conflicting versions. By following the step-by-step solutions outlined in this guide, you can effectively solve Maven dependency hells and ensure a smooth and stable project build. As always, it's essential to stay informed about best practices and keep dependencies up to date to maintain a healthy and efficient project.
Dealing with Maven dependency hells? Check out this step-by-step guide to solve your issues! #Java #Maven #DependencyManagement [Link to the blog post]
By following these steps, you can effectively navigate the complexities of Maven dependency management and ensure a stable and efficient project build. If you're looking to delve deeper into Maven best practices, the expansive guide provided by Maven Official Documentation is an invaluable resource.