Handling Checked Exceptions in Java: A Cool Dev’s Guide
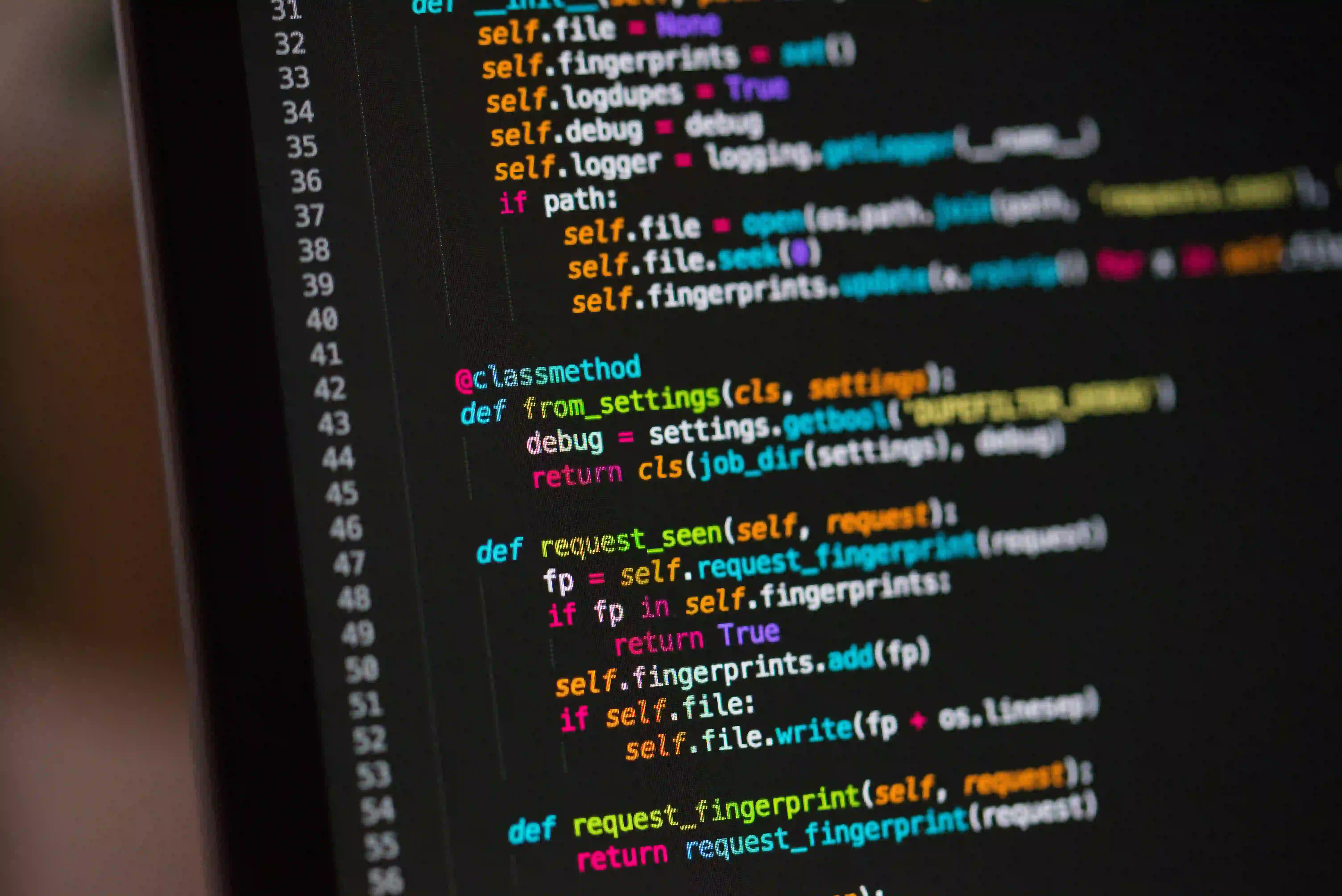
Handling Checked Exceptions in Java: A Cool Dev’s Guide
As a Java developer, you’re probably familiar with the pain of dealing with checked exceptions. They require you to handle or declare them, which can often lead to clunky and verbose code. But fear not! In this guide, we’ll explore some cool techniques and best practices for handling checked exceptions in Java, making your code more elegant and maintainable.
Understanding Checked Exceptions
Firstly, let’s recap what checked exceptions are. In Java, checked exceptions are exceptions that a method must either handle (catch and deal with) or declare using the throws
clause. This is in contrast to unchecked exceptions, which are subclasses of RuntimeException
and do not require mandatory handling.
Checked exceptions are typically used for error conditions that a method should be able to anticipate and handle. For example, I/O operations often throw checked exceptions such as IOException
, forcing calling code to deal with potential issues related to file handling.
The Traditional Approach
The traditional approach to handling checked exceptions is using try-catch
blocks. While this approach is effective, it often leads to repetitive and boilerplate code, especially when dealing with the same exceptions in multiple methods. Let’s take a look at an example:
public void readFile(String filePath) {
try {
FileReader fileReader = new FileReader(filePath);
// Read file
} catch (FileNotFoundException e) {
// Handle file not found exception
}
}
In the above code, the readFile
method handles the FileNotFoundException
by catching it within the method. While this works, it can make the code harder to read and maintain, especially if similar exception handling logic is spread across multiple methods.
The Cool Dev’s Approach: Exception Wrapping
One cool technique for handling checked exceptions more elegantly is to use exception wrapping. Instead of catching the exception directly within a method, you can wrap it in a custom unchecked exception and throw that instead. This approach can help reduce the verbosity of your code and centralize exception handling logic.
Let’s see how exception wrapping works in practice:
public void readFile(String filePath) {
try {
FileReader fileReader = new FileReader(filePath);
// Read file
} catch (FileNotFoundException e) {
throw new UncheckedIOException("Error reading file", e);
}
}
In this example, rather than handling the FileNotFoundException
directly, we create a new UncheckedIOException
and wrap the original exception within it. This allows us to propagate the exception up the call stack without being forced to declare it or handle it in every method along the way.
The Benefits of Exception Wrapping
Exception wrapping offers several benefits, making your code cleaner and more maintainable:
-
Reduced Boilerplate: By wrapping checked exceptions, you can avoid cluttering your code with repetitive
try-catch
blocks, leading to a more concise and readable codebase. -
Centralized Handling: Exception wrapping allows you to centralize the handling of checked exceptions, making it easier to manage and update error-handling logic.
-
Enables Functional Programming: In modern Java development, functional programming techniques such as streams and lambdas are widely used. Exception wrapping plays well with these paradigms by allowing unchecked exceptions to propagate through streams without the need for explicit handling at each step.
Leveraging Java 8’s Optional
With the release of Java 8, the Optional
class was introduced as a way to represent an optional value that may or may not be present. Interestingly, Optional
can also be used to handle exceptions in a more functional and expressive way.
Let's consider a scenario where a method performs a potentially failing operation and returns a result. Instead of explicitly throwing a checked exception, we can use Optional
to gracefully handle the exceptional case:
public Optional<String> readFromFile(String filePath) {
try {
// File reading logic
return Optional.of("File content");
} catch (IOException e) {
return Optional.empty();
}
}
In this example, the readFromFile
method returns an Optional
that either contains the file content or is empty in case of an exception. This approach enables the calling code to handle the exceptional case in a more functional and expressive manner.
A Final Look
In conclusion, while checked exceptions are an integral part of Java’s error-handling mechanism, they can sometimes lead to verbose and repetitive code. By leveraging techniques such as exception wrapping and Optional
, developers can make their code cleaner, more expressive, and easier to maintain.
Remember, a cool dev doesn’t just write code that works, but code that is elegant and delightful to read. So go ahead, embrace these techniques, and level up your exception handling game!
Now, armed with these strategies, you're ready to handle checked exceptions in Java like a pro.
Happy coding!
[Learn more about Java exceptions and best practices at [Java Exception Handling](https://www.baeldung.com/java-exceptions)]
[Explore the power of Java 8’s Optional at [Java Optional Guide](https://www.oracle.com/technical-resources/articles/java/java8-optional.html)]