Unlocking High Performance: Overcoming OpenHFT Java Lang Challenges
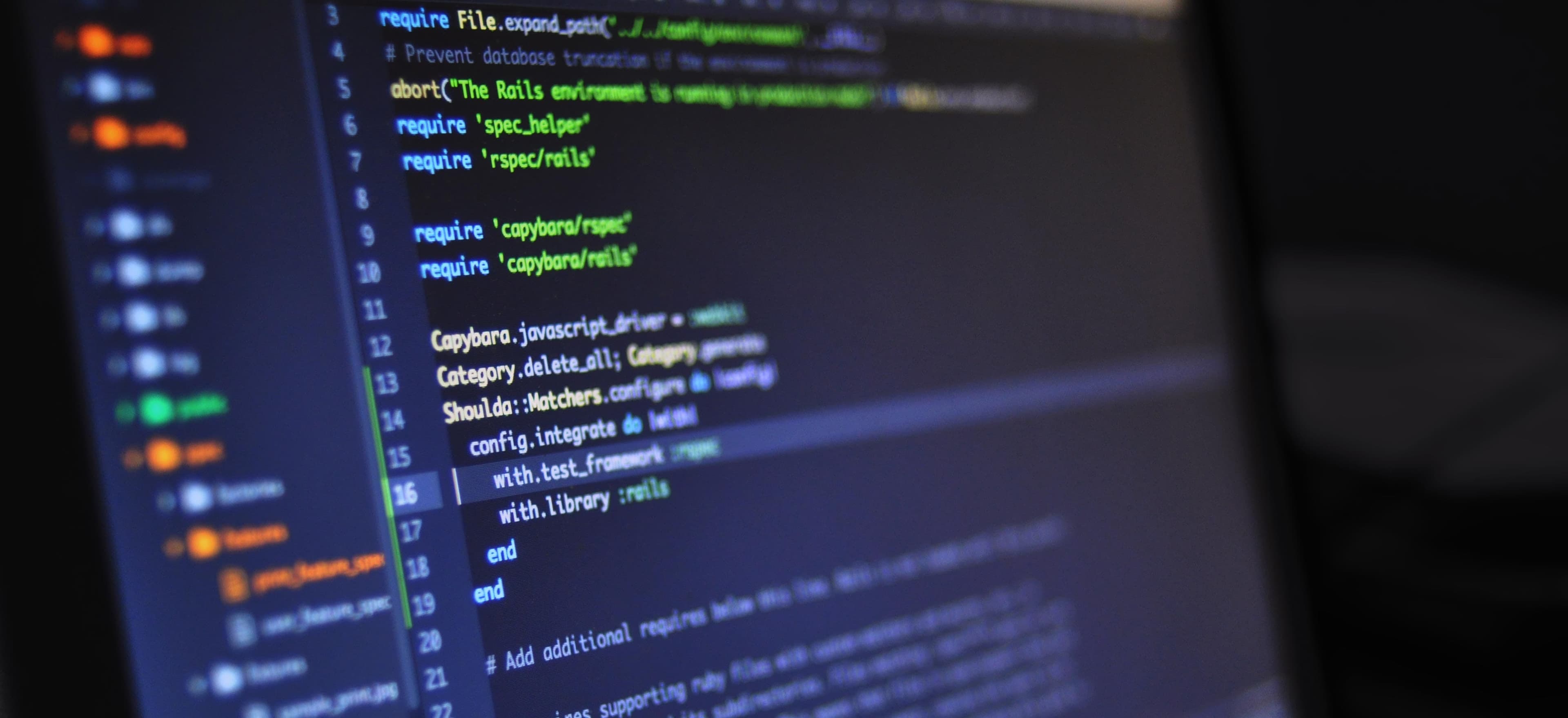
- Published on
Unlocking High Performance: Overcoming OpenHFT Java Lang Challenges
In today's rapidly evolving technology landscape, the demand for high-performance computing is ever-increasing. Java, a stalwart in the programming world, has been the go-to language for building robust and scalable applications. However, when it comes to ultra-low latency and high-frequency trading (HFT) systems, Java faces unique challenges that demand a different approach. This blog post will delve into OpenHFT, its associated challenges, and the strategies to overcome them using Java.
The OpenHFT Framework
OpenHFT is a high-performance, off-heap, and low-latency library for Java. It provides solutions for shared memory, IPC, off-heap memory allocation, persistence, and high-frequency messaging. The library is renowned for its exceptional speed and efficiency, making it an ideal choice for ultra-low latency applications.
Challenges with OpenHFT in Java
While OpenHFT offers unprecedented performance benefits, it also presents certain challenges, particularly in the context of Java programming. Some of the key challenges developers encounter include:
-
Complex Memory Management: OpenHFT's off-heap data structures and memory management necessitate a deep understanding of memory allocation and deallocation, which can be intricate in the Java ecosystem.
-
Concurrency Control: Java's native concurrency control mechanisms, while robust, may not be fully aligned with the requirements of high-frequency trading systems. OpenHFT introduces its own set of concurrency complexities that need to be carefully addressed.
-
Optimized Data Access: To harness the full potential of OpenHFT, developers need to assimilate efficient strategies for data access, leveraging the library's mechanisms for best performance results.
Overcoming OpenHFT Java Lang Challenges
1. Utilizing Off-Heap Memory
One of the key strengths of OpenHFT is its ability to work with off-heap memory. This feature facilitates faster access and reduces the impact of garbage collection pauses. Let's consider an example of how to leverage off-heap memory using OpenHFT's Bytes
class:
// Allocate off-heap memory for 1MB
Bytes bytes = NativeBytes.nativeBytes(1_000_000);
// Write data to off-heap memory
bytes.writeLong(0, 1234567890);
In this code snippet, we allocate 1MB of off-heap memory using NativeBytes.nativeBytes()
. Subsequently, we write a long value to the allocated memory. By utilizing off-heap memory in this manner, we sidestep the limitations of on-heap memory management in Java, thereby enhancing performance.
2. Leveraging OpenHFT's Locking Strategies
Concurrency control is a critical aspect of high-performance systems, and OpenHFT offers specialized locking strategies to address this requirement. The library provides advanced locking mechanisms such as ReadWriteLock
and OneToOneConcurrentOffHeapMap
. These mechanisms enable fine-grained control over concurrent access to shared off-heap data structures, ensuring optimal performance in multi-threaded environments.
// Example of using ReadWriteLock from OpenHFT
Lock lock = new ReentrantReadWriteLock().writeLock();
lock.lock();
try {
// Critical section
} finally {
lock.unlock();
}
By using OpenHFT's locking strategies, developers can mitigate concurrency issues prevalent in high-frequency trading systems, paving the way for smoother and more efficient execution paths.
3. Optimizing Data Access Patterns
Efficient data access patterns are pivotal for achieving high performance with OpenHFT. The library offers a multitude of data structures optimized for rapid data retrieval, such as OffHeapMap
and OffHeapQueue
. When working with these data structures, adhering to best practices for data access is crucial. For instance, utilizing direct memory access can significantly expedite read and write operations.
// Example of efficient data access using OffHeapMap
OffHeapMap<Long, String> offHeapMap = OffHeapMap.create(1000, LongSerializer.LONG, ObjectSerializer.UTF16);
offHeapMap.put(1L, "Hello, OpenHFT!");
String value = offHeapMap.get(1L);
In this example, we create an OffHeapMap
and demonstrate efficient data access by storing and retrieving a key-value pair. The employed data access pattern ensures optimal utilization of OpenHFT's capabilities, ultimately contributing to superior performance.
The Bottom Line
OpenHFT is a powerful library offering exceptional performance for high-frequency trading and low-latency applications. While Java presents certain challenges in leveraging OpenHFT to its full potential, adept developers can overcome these obstacles by harnessing the library's features in a judicious manner. By embracing off-heap memory, employing effective locking strategies, and optimizing data access patterns, Java developers can unlock the true performance potential of OpenHFT in their applications, thereby meeting the demands of ultra-low latency and high-frequency trading systems.
By understanding the intricacies of OpenHFT and its compatibility with Java, developers can pave the way for high-performance solutions that meet the rigors of modern, real-time computing environments.
For additional insights on high-performance Java programming and OpenHFT, consider exploring resources such as the official OpenHFT documentation and the Java Memory Model for a deeper understanding of memory management in Java.
Unlocking the potential of OpenHFT in Java not only empowers developers to build high-performance systems but also propels the realm of real-time computing towards unprecedented efficiency and speed.