Cracking Redis: Dynamic APIs with Java Command Interfaces
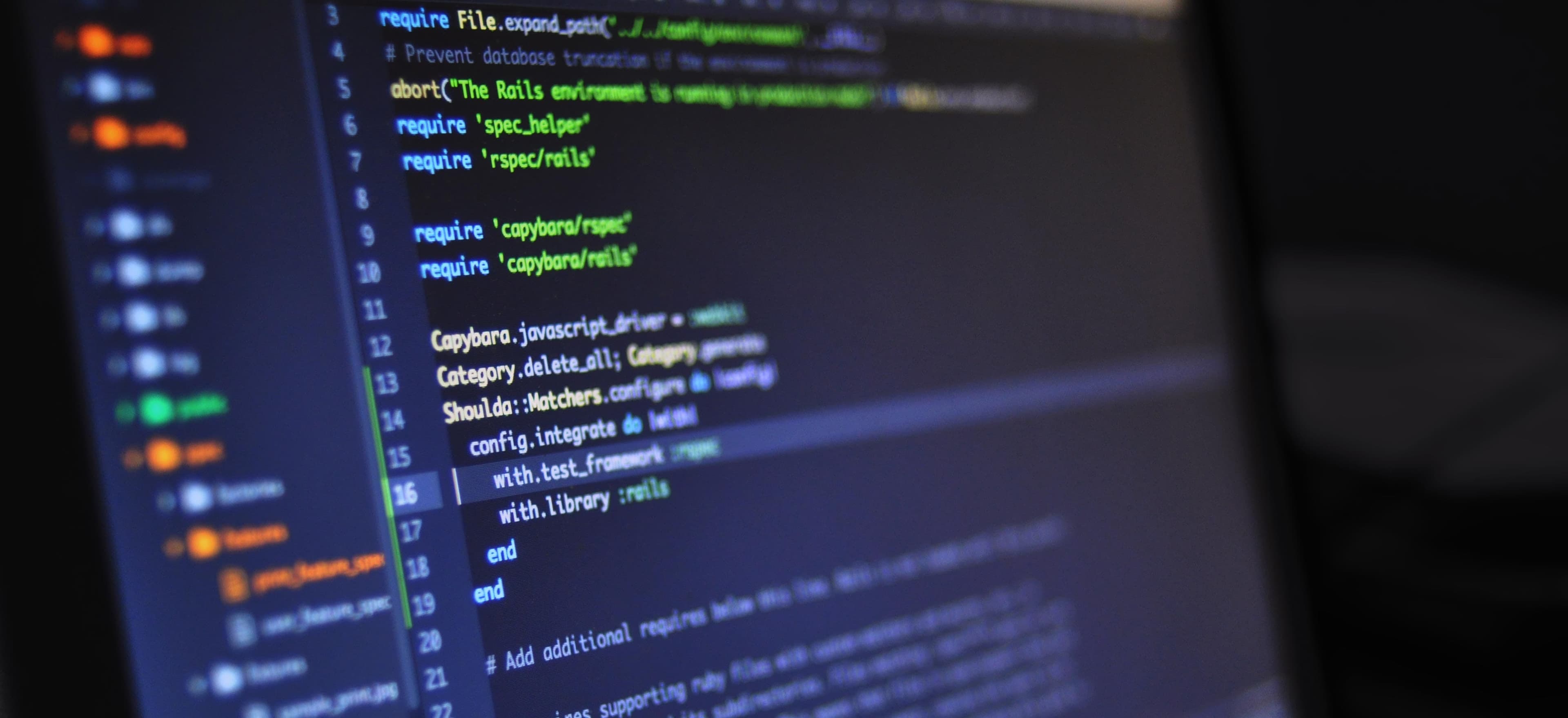
- Published on
Cracking Redis: Dynamic APIs with Java Command Interfaces
In the world of web development, building dynamic APIs is crucial for creating responsive and interactive applications. Redis, a popular in-memory data store, provides a powerful platform for implementing dynamic APIs. When combined with Java, Redis can be used to build robust command interfaces for seamless data manipulation. In this article, we'll dive into the exciting realm of building dynamic APIs with Java command interfaces powered by Redis.
Understanding Redis
Redis is an open-source, in-memory data structure store that can be used as a database, cache, and message broker. Its high performance, support for various data structures, and versatility make it an ideal choice for building dynamic APIs. Redis supports key-value, hash, list, set, and other data structures, which can be manipulated using a rich set of commands.
Java and Redis Integration
To implement dynamic APIs with Redis in Java, we can use the Jedis library, a Redis Java client that provides a high-level API for interacting with Redis. Jedis allows Java developers to perform operations such as setting and getting values, working with hashes, lists, sets, and more, making it a powerful tool for building command interfaces.
Setting Up the Environment
Before diving into the code, let's ensure that the environment is set up properly. We need to have Redis installed and running on our system. You can follow the official guide for downloading and installing Redis. Additionally, we need to include the Jedis library in our Java project. We can do this by adding the following Maven dependency:
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>3.7.0</version>
</dependency>
Connecting to Redis
The first step in building dynamic APIs with Redis in Java is establishing a connection to the Redis server. Let's see how we can achieve this using Jedis:
import redis.clients.jedis.Jedis;
public class RedisConnection {
public static void main(String[] args) {
// Connect to Redis server running on localhost
Jedis jedis = new Jedis("localhost");
System.out.println("Connected to Redis");
// Check if the server is running
System.out.println("Server is running: " + jedis.ping());
}
}
In the above code, we create a Jedis
instance and connect to the Redis server running on localhost
. We then verify the connection by sending a PING
command to the server. If the server is running, it will respond with "PONG."
Working with Key-Value Pairs
One of the fundamental operations in Redis is working with key-value pairs. Let's look at how we can set and get values using Jedis:
Setting a Value
// Setting a key-value pair
jedis.set("name", "John Doe");
Getting a Value
// Getting the value for a key
String name = jedis.get("name");
System.out.println("Name: " + name);
In the above code snippets, we set a key "name" with the value "John Doe" and then retrieve the value for the key "name." This demonstrates how easy it is to perform basic key-value operations in Redis using Jedis.
Working with Data Structures
Redis supports a variety of data structures such as lists, sets, and hashes. Let's explore how we can work with these data structures using Jedis.
Working with Lists
// Adding items to a list
jedis.lpush("tasks", "Task 3");
jedis.lpush("tasks", "Task 2");
jedis.lpush("tasks", "Task 1");
// Fetching items from the list
List<String> tasks = jedis.lrange("tasks", 0, -1);
for (String task : tasks) {
System.out.println("Task: " + task);
}
In the above code, we add three tasks to a list using the LPUSH
command and then retrieve all the tasks using the LRANGE
command.
Working with Sets
// Adding members to a set
jedis.sadd("tags", "Redis");
jedis.sadd("tags", "Java");
jedis.sadd("tags", "APIs");
// Fetching all members of the set
Set<String> tags = jedis.smembers("tags");
for (String tag : tags) {
System.out.println("Tag: " + tag);
}
Here, we add tags to a set and then retrieve all the tags using the SMEMBERS
command.
Working with Hashes
// Setting fields and values in a hash
jedis.hset("user:1000", "name", "Alice");
jedis.hset("user:1000", "email", "alice@example.com");
// Getting values from a hash
String userName = jedis.hget("user:1000", "name");
String userEmail = jedis.hget("user:1000", "email");
System.out.println("User Name: " + userName);
System.out.println("User Email: " + userEmail);
In the above example, we use hashes to store user information, with each user having a unique identifier. We set the name and email fields for a user with the ID "1000" and then retrieve the values using the HGET
command.
Building Command Interfaces
Now that we have seen how to interact with Redis using Jedis, let's harness this knowledge to build a dynamic API with a command interface. We'll create a simple Java application that allows users to add, retrieve, and delete tasks from a to-do list stored in Redis.
Adding Tasks
// Method to add a task to the to-do list
public void addTask(String task) {
jedis.lpush("todolist", task);
}
Retrieving Tasks
// Method to retrieve all tasks from the to-do list
public List<String> getTasks() {
return jedis.lrange("todolist", 0, -1);
}
Deleting Tasks
// Method to delete a task from the to-do list
public void deleteTask(String task) {
jedis.lrem("todolist", 0, task);
}
By encapsulating these operations in methods, we have created a simple command interface for manipulating the to-do list in Redis. This showcases how Java and Redis can be combined to build powerful and dynamic APIs.
My Closing Thoughts on the Matter
In this article, we delved into the world of building dynamic APIs with Java command interfaces powered by Redis. We explored the integration of Java and Redis using the Jedis library, and we learned how to perform basic operations such as connecting to Redis, working with key-value pairs, and manipulating various data structures. Finally, we applied this knowledge to build a simple command interface for a to-do list, showcasing the potential of using Java and Redis for creating dynamic APIs.
Redis, with its blazing speed and support for various data structures, coupled with the versatility of Java, opens up endless possibilities for building robust and responsive dynamic APIs.
In conclusion, the combination of Java and Redis presents a compelling proposition for developers looking to create powerful, scalable, and dynamic APIs. With the right tools and knowledge, the potential for innovation and creativity in this space is limitless.
So, roll up your sleeves, dive into the world of Java and Redis, and unleash the full potential of dynamic APIs!
Remember, the power of dynamic data manipulation is now in your hands, thanks to Java and Redis.
Happy coding!
Disclaimer: This document is for educational purposes only and does not guarantee performance improvements in every scenario. Use the provided information with discretion, and always conduct thorough testing before implementing any changes in a production environment.