Boost Your Data Insights: Mastering Bootstrap Testing
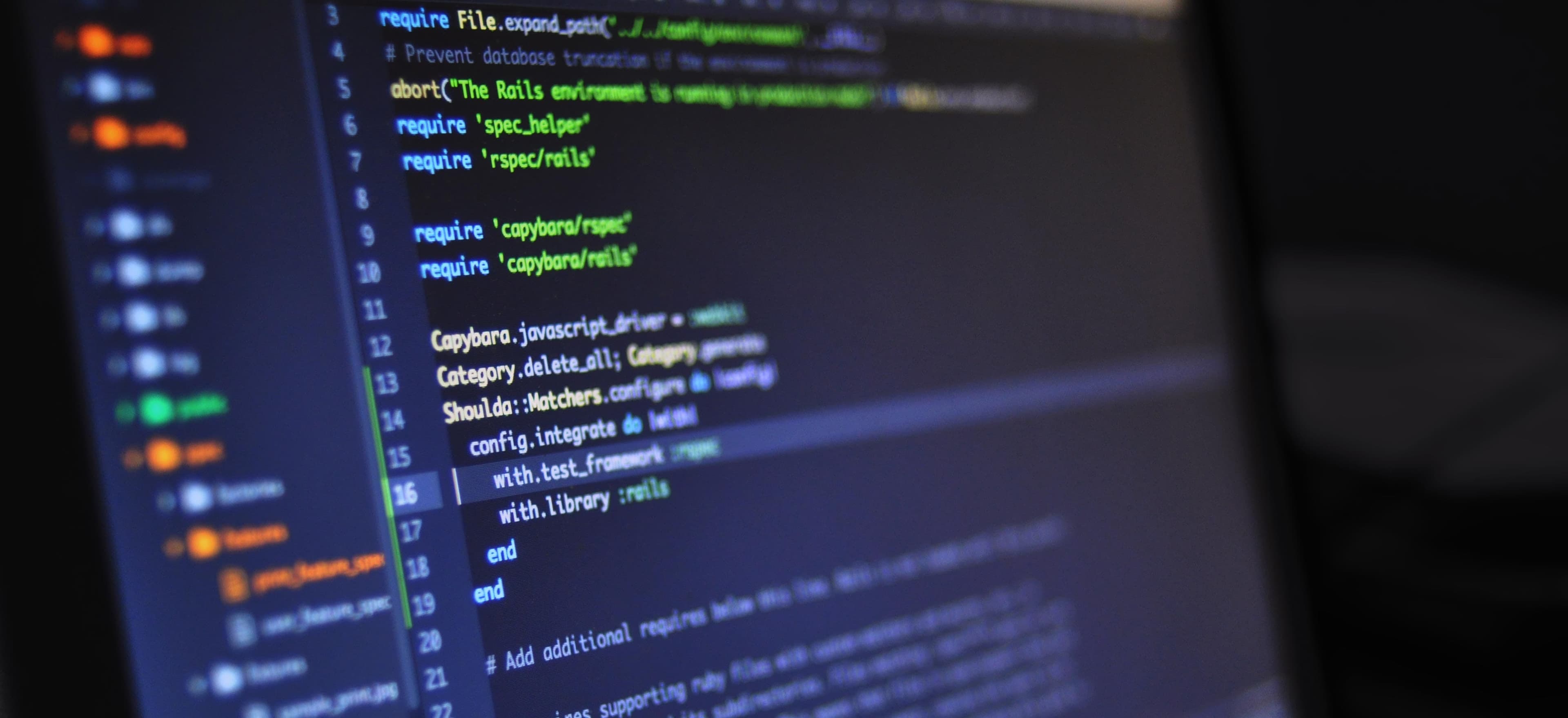
- Published on
Understanding Bootstrap Testing in Java
When it comes to data analysis, making informed decisions relies heavily on the validity and accuracy of statistical inferences. This is where Bootstrap Testing comes into play. In the realm of Java programming, understanding and implementing Bootstrap Testing can significantly enhance the reliability of data insights. In this blog post, we will delve into the concept of Bootstrap Testing, its significance, and how Java can be leveraged to harness its power.
What is Bootstrap Testing?
Bootstrap Testing is a resampling method used to estimate the sampling distribution of a statistic by repeatedly resampling with replacement from the original data. This technique is particularly useful when the underlying distribution of the data is unknown or when the sample size is limited. By creating numerous resampled datasets, Bootstrap Testing empowers analysts to derive estimates, confidence intervals, and assess the significance of statistical measures.
The Significance of Bootstrap Testing
Traditional statistical methods often assume specific distributional forms or large sample sizes. However, real-world data may not conform to these assumptions, leading to biased or inaccurate results. Bootstrap Testing, on the other hand, does not rely on stringent assumptions about the data's distribution, making it robust and versatile. It provides a powerful tool for estimating the sampling distribution of a statistic and enhancing the precision of inferential statistics.
Implementing Bootstrap Testing in Java
Now, let's shift our focus to implementing Bootstrap Testing in Java. Leveraging Java's rich ecosystem of libraries such as Apache Commons Math and JBLAS, we can readily utilize Bootstrap Testing to derive valuable insights from data.
Below is a simplified example of implementing Bootstrap Testing for estimating the mean of a sample in Java:
import org.apache.commons.math3.stat.descriptive.DescriptiveStatistics;
import org.apache.commons.math3.random.RandomGeneratorFactory;
import org.apache.commons.math3.random.RandomGenerator;
import org.apache.commons.math3.distribution.RealDistribution;
import org.apache.commons.math3.distribution.NormalDistribution;
public class BootstrapTesting {
public static void main(String[] args) {
// Sample data
double[] data = {25.3, 30.2, 28.5, 32.7, 26.8, 29.1, 27.6, 31.4, 30.5, 28.9};
// Number of bootstrap samples
int numSamples = 1000;
// Perform Bootstrap Testing
DescriptiveStatistics descriptiveStatistics = new DescriptiveStatistics();
RandomGenerator randomGenerator = RandomGeneratorFactory.createRandomGenerator();
RealDistribution realDistribution = new NormalDistribution();
for (int i = 0; i < numSamples; i++) {
double[] resampledData = new double[data.length];
for (int j = 0; j < data.length; j++) {
int index = randomGenerator.nextInt(data.length);
resampledData[j] = data[index];
}
descriptiveStatistics.addValue(realDistribution.getMean(resampledData));
}
// Compute bootstrap estimate
double bootstrapMean = descriptiveStatistics.getMean();
System.out.println("Bootstrap estimate of the mean: " + bootstrapMean);
}
}
In this example, we use Apache Commons Math library to perform Bootstrap Testing for estimating the mean of a sample dataset. We create resampled datasets by bootstrapping the original data, compute the mean for each resampled dataset, and then derive the Bootstrap estimate of the mean.
Why Use Bootstrap Testing in Java?
Utilizing Bootstrap Testing in Java offers several compelling advantages. Firstly, Java's strong support for statistical and mathematical operations, coupled with its extensive library ecosystem, provides a solid foundation for implementing Bootstrap Testing with ease. Additionally, Java's platform independence and scalability make it an ideal choice for processing large-scale data and performing computationally intensive tasks, which are often inherent to Bootstrap Testing.
Furthermore, incorporating Bootstrap Testing in Java enables developers and analysts to seamlessly integrate it within existing applications, data pipelines, and analytical workflows. This facilitates the extraction of robust statistical insights and enhances the overall reliability of decision-making processes.
The Bottom Line
In conclusion, mastering Bootstrap Testing in Java equips data analysts and developers with a potent tool for deriving reliable statistical inferences and enhancing the accuracy of data insights. By embracing the flexibility and power of Bootstrap Testing, coupled with Java's robust capabilities, practitioners can bolster their data analysis endeavors and make well-founded decisions based on sound statistical foundations.
Incorporating Bootstrap Testing in Java represents a pivotal step towards unlocking the full potential of data-driven decision-making, ultimately paving the way for more informed and impactful outcomes in diverse domains ranging from finance and healthcare to e-commerce and beyond.
To delve deeper into Bootstrap Testing and its applications in data analysis, consider exploring the resources provided by Statistical Bootstrap Methods and Apache Commons Math.
Master Bootstrap Testing in Java, and empower your data insights like never before!
Checkout our other articles