Boosting Microservices with ZooKeeper: The Secret to Efficient Discovery
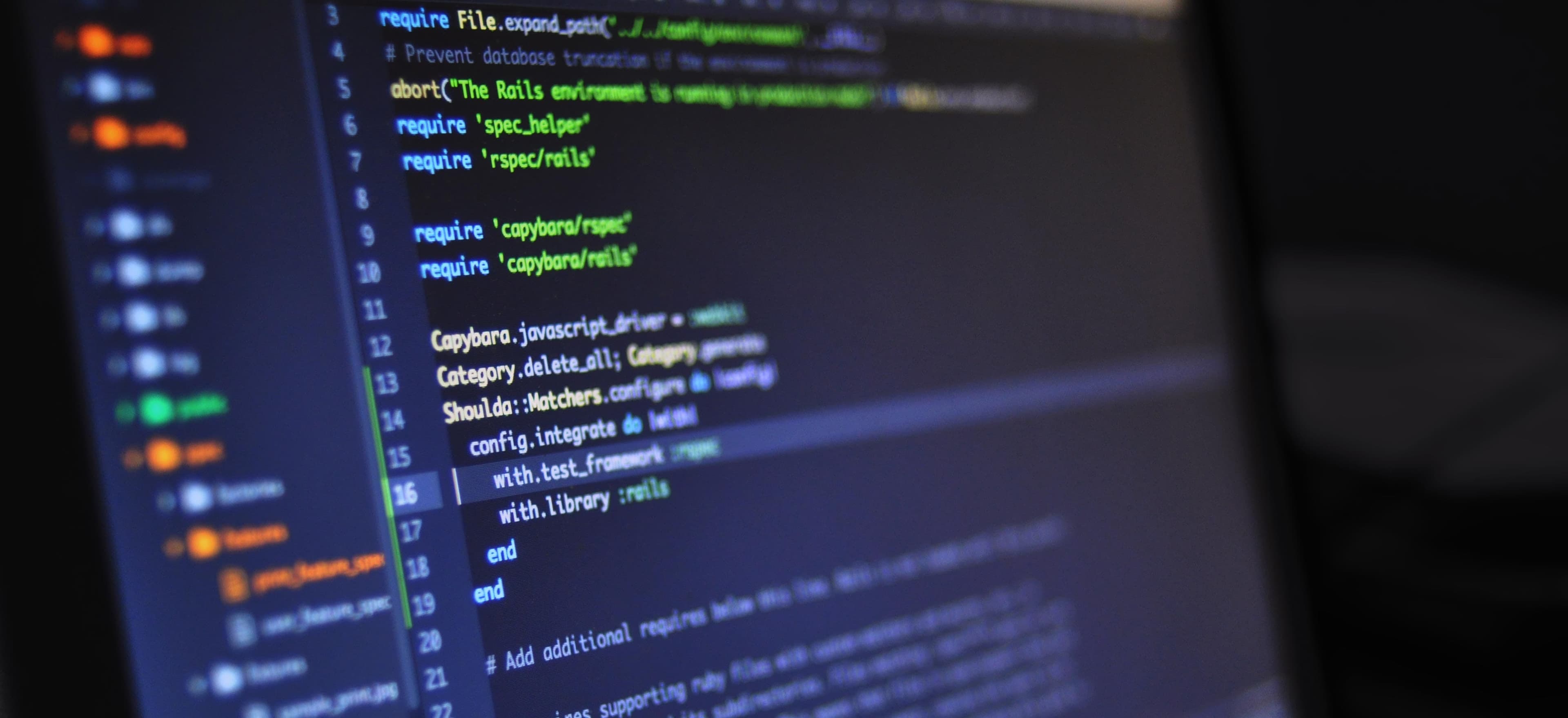
- Published on
Boosting Microservices with ZooKeeper: The Secret to Efficient Discovery
In the realm of microservices architecture, the efficient discovery of services is crucial for seamless communication and collaboration between various components. This is where Apache ZooKeeper shines as a robust and reliable coordination service, facilitating service discovery and synchronization in distributed systems.
What is ZooKeeper?
Apache ZooKeeper is a centralized service for maintaining configuration information, providing distributed synchronization, and naming. It's designed to be highly available, scalable, and fault-tolerant, making it an ideal choice for managing coordination tasks across large, distributed systems.
Why ZooKeeper for Microservices?
When it comes to the complexities of microservices, having a centralized system that stores configuration details, coordinates distributed services, and manages group membership is a game-changer. ZooKeeper acts as the backbone for service discovery, enabling seamless communication and coordination between microservices.
Setting Up ZooKeeper
To get started with ZooKeeper, you first need to set up a ZooKeeper ensemble. This involves creating a cluster of ZooKeeper servers that work together to provide a reliable and fault-tolerant service. Once the ensemble is in place, you can begin leveraging its features for microservices discovery and coordination.
Integrating ZooKeeper for Service Discovery
One of the fundamental use cases of ZooKeeper in microservices is service discovery. By registering microservices with ZooKeeper and watching for changes, other services can dynamically discover and communicate with them. Let's dive into a simple example of how ZooKeeper can be integrated into a Java-based microservice for efficient service discovery.
Example: Java-Based Microservice Integration with ZooKeeper
import org.apache.zookeeper.*;
public class ServiceRegistry {
private final ZooKeeper zooKeeper;
private static final String ZOOKEEPER_ADDRESS = "your_zookeeper_address";
public ServiceRegistry() {
this.zooKeeper = new ZooKeeper(ZOOKEEPER_ADDRESS, 5000, event -> {});
}
public void registerService(String serviceName, String serviceAddress) {
String servicePath = "/services/" + serviceName;
try {
if (zooKeeper.exists(servicePath, false) == null) {
zooKeeper.create(servicePath, new byte[]{}, ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.PERSISTENT);
}
String addressPath = servicePath + "/" + serviceAddress;
zooKeeper.create(addressPath, new byte[]{}, ZooDefs.Ids.OPEN_ACL_UNSAFE, CreateMode.EPHEMERAL);
} catch (KeeperException | InterruptedException e) {
e.printStackTrace();
}
}
}
In this example, we create a ServiceRegistry
class responsible for registering a microservice with ZooKeeper. When a microservice starts up, it can use this class to register itself so that other services can discover and communicate with it.
Why This Works
-
ZooKeeper Connection: The
ZooKeeper
class from the Apache ZooKeeper library is used to establish a connection to the ZooKeeper ensemble. -
Service Registration: The
registerService
method creates a znode for the microservice under the/services
path in ZooKeeper and registers its address as an ephemeral node. This allows the microservice to dynamically register and deregister itself based on its availability. -
Fault Tolerance: ZooKeeper's fault-tolerant nature ensures that the service registry remains available even in the face of network partitions or server failures.
By integrating ZooKeeper for service discovery, microservices can dynamically register and discover one another, fostering a highly adaptable and robust architecture.
My Closing Thoughts on the Matter
In the world of microservices, efficient service discovery is paramount for seamless interaction between distributed components. Apache ZooKeeper offers a powerful solution for managing service registration, synchronization, and discovery in a fault-tolerant and scalable manner. By integrating ZooKeeper into Java-based microservices, developers can elevate the reliability and dynamism of their architecture, laying the foundation for a robust and scalable system.
Discover the power of Apache ZooKeeper and unlock the full potential of your microservices architecture today!
To delve deeper into the world of microservices and ZooKeeper integration, check out ZooKeeper's official documentation and Apache Curator, a high-level ZooKeeper client library that simplifies the interaction with ZooKeeper for microservices and other distributed systems.
Checkout our other articles