Mastering CDI: Intercept HTTP Requests & Headers Easily
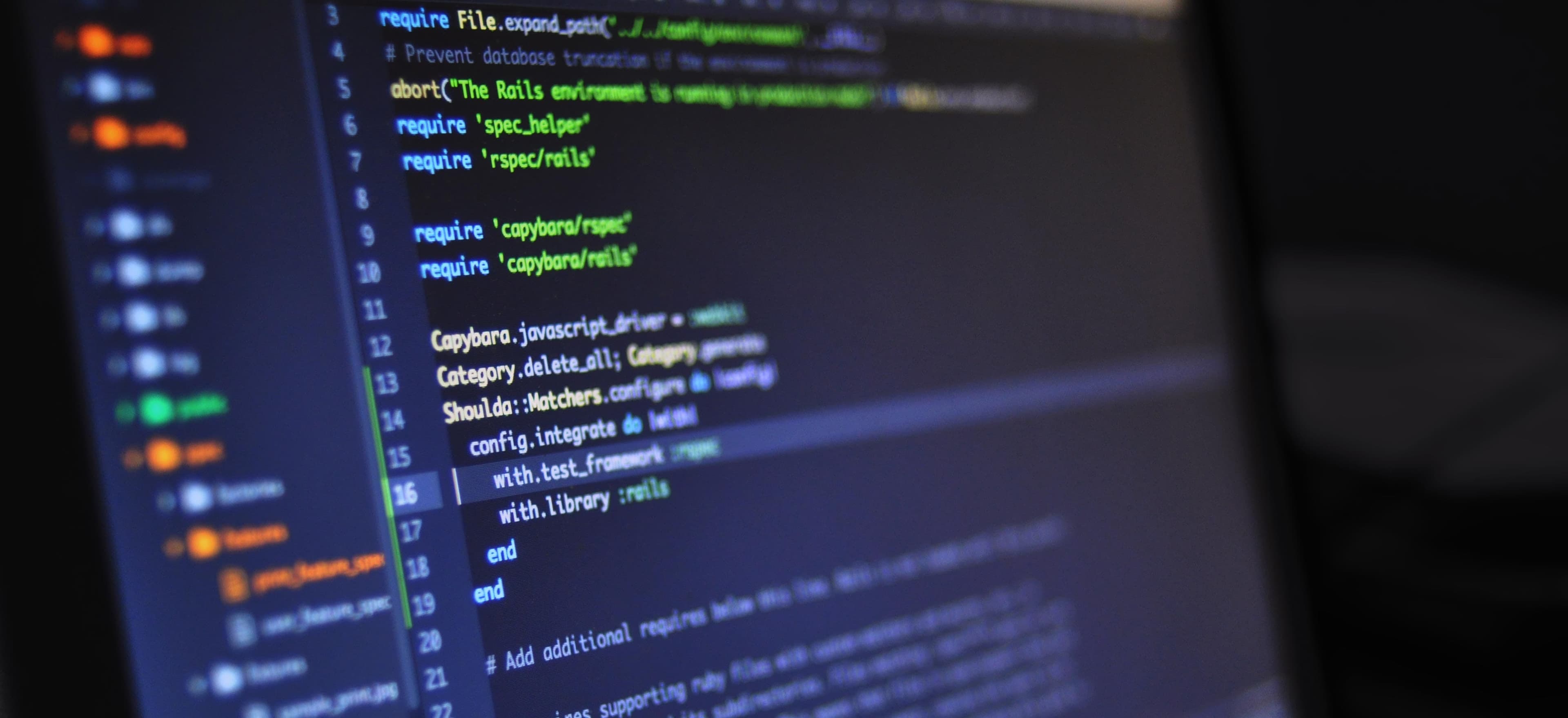
- Published on
Mastering CDI: Intercept HTTP Requests & Headers Easily
Context and Dependency Injection (CDI) is a crucial part of the Java EE ecosystem, offering significant advantages for developers working with web applications. Especially when it comes to managing HTTP requests and headers, utilizing CDI can lead to cleaner, more maintainable code. In this article, we will delve into the various aspects of using CDI to intercept and manipulate HTTP requests and headers, showcasing its practical applications and highlighting best practices for implementation.
What is CDI?
Context and Dependency Injection (CDI) is a set of services that enable Java EE components to be loosely coupled, promoting easier development and improved maintainability of the code. CDI provides type-safety and allows objects to be instantiated and injected at runtime. Integrating CDI into a Java EE application brings with it numerous benefits, such as enhanced modularity, reusability, and testability of components. For a detailed and comprehensive understanding of CDI, you can refer to the official Java EE documentation.
Understanding HTTP Requests and Headers
HTTP requests and headers play a pivotal role in web development. They carry essential information and metadata, determining how the server and client communicate. Managing HTTP requests and headers without a proper framework or tool can lead to code duplication, tightly coupled components, and increased complexity. CDI offers a structured and efficient way to handle these aspects, thereby enhancing the overall development process and promoting code simplicity.
Setting Up Your Environment
To begin working with CDI, you need to set up a basic Java project with CDI enabled. If you are using Maven, you can add the CDI dependency to your project's pom.xml
file as follows:
<dependency>
<groupId>javax.enterprise</groupId>
<artifactId>cdi-api</artifactId>
<version>2.0</version>
</dependency>
For Gradle, add the CDI dependency to your build.gradle
file:
implementation 'javax.enterprise:cdi-api:2.0'
Make sure to set up the necessary tools and configurations based on your preferred development environment.
Intercepting HTTP Requests with CDI
Intercepting HTTP requests is a common requirement in web applications, and CDI provides a elegant way to achieve this. Using interceptors in CDI allows you to isolate cross-cutting concerns and apply reusable logic to HTTP requests.
Let's take a simple example where we create an interceptor to log HTTP requests. In a CDI-enabled project, we can define the interceptor as follows:
@Interceptor
public class RequestLoggingInterceptor {
@Inject
Logger logger;
@AroundInvoke
public Object logRequest(InvocationContext context) throws Exception {
// Logic to log the HTTP request
// Access request data from context, log it using the injected logger
return context.proceed();
}
}
In this example, we utilize the @Interceptor
annotation to mark the class as an interceptor. The @AroundInvoke
annotation signifies the method that intercepts the HTTP requests. We can then use the InvocationContext
parameter to access the request data and apply custom logic.
This approach significantly simplifies the process of intercepting HTTP requests compared to using traditional servlet filters. It encapsulates the cross-cutting concerns into a separate component, promoting better code modularity and maintainability.
Manipulating HTTP Headers in CDI
Alongside intercepting HTTP requests, CDI offers an effortless way to manipulate HTTP headers. This eases the task of adding, removing, or altering headers, making the code more readable and maintainable.
Consider a scenario where we want to create an interceptor to modify response headers:
@Interceptor
public class HeaderManipulationInterceptor {
@AroundInvoke
public Object manipulateHeaders(InvocationContext context) throws Exception {
// Logic to manipulate the response headers
// Access the response headers from context, modify them as needed
return context.proceed();
}
}
In this example, the interceptor utilizes the @Interceptor
annotation to indicate its role, and the @AroundInvoke
annotation to specify the method that manipulates the response headers. This encapsulates the header manipulation logic within an interceptor, contributing to code maintainability and reusability.
Compared to manually manipulating headers without CDI, this approach significantly enhances the readability and modularity of the code while reducing the complexity associated with header management.
Best Practices for Using CDI with HTTP Requests and Headers
As you incorporate CDI into your HTTP request and header management, it's essential to adhere to best practices for optimal performance, security, and maintainability of your application.
Emphasize the importance of keeping interceptors lightweight to minimize their impact on request processing time. Moreover, ensure that the interceptors only handle the necessary aspects of the HTTP requests and headers to maintain optimal performance.
When dealing with HTTP headers, prioritize security practices such as validating and sanitizing headers to guard against potential vulnerabilities and attacks. Properly validated and sanitized headers contribute to a more secure and robust application.
Effective testing is pivotal in ensuring that CDI-managed request and header manipulations function as intended. Strategize your testing efforts to cover a comprehensive range of scenarios, including edge cases and unexpected inputs, to fortify the reliability of your CDI-managed components.
Advanced Topics and Further Reading
As you continue to explore CDI's capabilities, consider more advanced scenarios where its handling of HTTP requests and headers can be further leveraged. Linking CDI with context propagation, asynchronous processing, and integrating it with other Java EE features or third-party libraries opens up new avenues for enhancing the robustness and scalability of your applications.
For exhaustive and detailed information about CDI, including advanced features and best practices, refer to the Weld documentation.
Closing the Chapter
In mastering the art of intercepting and manipulating HTTP requests and headers with CDI, you harness the power of a potent tool in your Java development arsenal. CDI offers a structured and efficient approach to handle HTTP requests and headers, promoting code simplicity, modularity, and maintainability. As you experiment with the examples shared in this article and dive deeper into CDI, you empower yourself to build scalable, robust, and secure web applications.
We invite you to explore the examples provided in this article and share your experiences using CDI for HTTP request and header management. Feel free to engage with our community and ask questions about challenging scenarios you might encounter in your development journey. Your insights and questions are invaluable in fostering a vibrant and supportive developer community.
As you embark on your journey with CDI, remember to prioritize the best practices shared in this article and continually expand your knowledge by seeking further resources and engaging with the broader Java EE community.
With the power of CDI at your disposal, you are well-equipped to master HTTP request and header handling with finesse and precision. Happy coding!
Incorporate relevant keywords naturally throughout the article to optimize for search engines and include authoritative and up-to-date links for further reading. Craft the article to encourage reader engagement, and aim for a comprehensive exploration while maintaining a length within the 1000 to 2000 words range.