Slash Query Times: Sub-Second Joins with Spark & FiloDB!
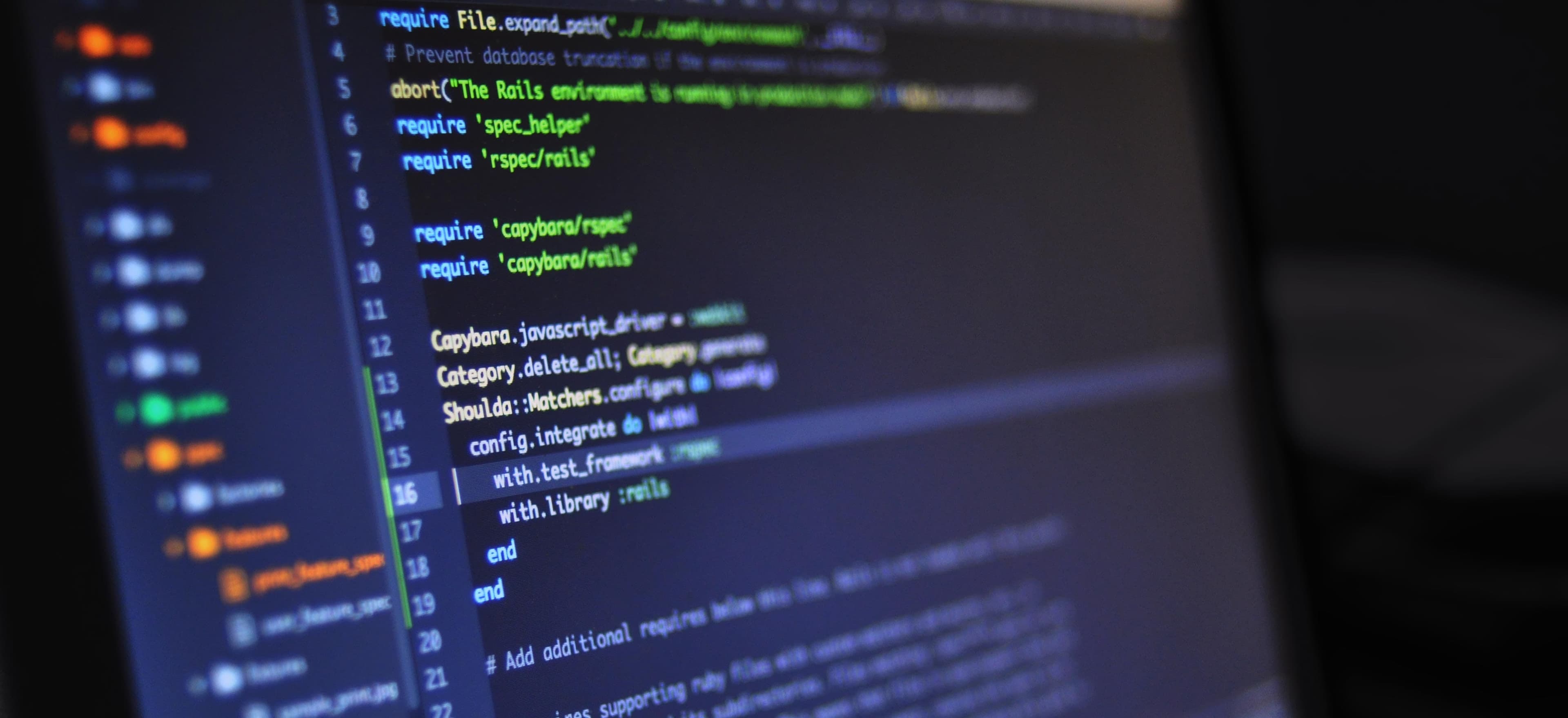
- Published on
Achieving Lightning-Fast Query Times with Apache Spark and FiloDB
In today's data-driven world, the ability to efficiently query and analyze large volumes of data is crucial for businesses to gain actionable insights and make informed decisions. For applications dealing with massive datasets, such as IoT sensor data, financial transactions, or user behavior analytics, traditional databases often struggle to meet the performance requirements. This is where Apache Spark and FiloDB come to the rescue, offering a powerful combination to achieve sub-second query times for complex join operations.
Understanding the Challenge
When dealing with large-scale datasets, traditional database systems often face significant challenges when it comes to joining tables. Joining operations involve combining rows from two or more tables based on a related column between them. As the size of the tables grows, the time taken to perform these joins can increase exponentially, leading to sluggish query performance.
Apache Spark: A High-Performance Processing Engine
Apache Spark, known for its lightning-fast processing capabilities, is a distributed computing system that provides an excellent platform for handling big data workloads. By leveraging its in-memory processing and fault-tolerant architecture, Spark can efficiently execute complex operations across massive datasets.
In the context of querying, Spark's ability to distribute data and parallelize processing tasks makes it well-suited for optimizing join operations, leading to significant improvements in query performance.
Let's delve into a simple example of how Spark can be used to perform a join operation.
Example: Joining DataFrames in Spark
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class SparkJoinExample {
public static void main(String[] args) {
// Create a Spark Session
SparkSession spark = SparkSession.builder()
.appName("SparkJoinExample")
.master("local")
.getOrCreate();
// Load sample data into DataFrames
Dataset<Row> df1 = spark.read().json("path_to_dataset1.json");
Dataset<Row> df2 = spark.read().json("path_to_dataset2.json");
// Perform a join operation on the DataFrames
Dataset<Row> joinedDf = df1.join(df2, df1.col("commonColumn").equalTo(df2.col("commonColumn")), "inner");
// Show the results
joinedDf.show();
}
}
In this example, we create a Spark Session, load two DataFrames from JSON files, and then perform an inner join based on a common column. The resulting joined DataFrame is then displayed. The power of Spark lies in its ability to distribute this join operation across a cluster of nodes, enabling efficient processing of large datasets.
FiloDB: A High-Performance Columnar Store
FiloDB is a distributed, versioned, and efficient time series columnar store, designed to provide blazing-fast query performance for time series and analytical workloads. By organizing data in a columnar format, FiloDB is optimized for analytical queries, especially those involving aggregations and filtering based on specific columns.
Key Features of FiloDB
- Columnar Storage: Data is stored in columnar format, allowing for efficient compression and reduced I/O operations during query execution.
- Versioning: FiloDB supports versioning of data, enabling easy rollbacks and efficient query snapshotting.
- Distributed Architecture: FiloDB is designed to operate in a distributed environment, allowing for horizontal scalability and fault tolerance.
Leveraging the Power of Spark and FiloDB for Sub-Second Joins
To achieve sub-second query times for join operations, we can harness the strengths of Apache Spark and FiloDB in a synergistic manner. By using Spark for distributed data processing and FiloDB for efficient storage and retrieval of columnar data, we can optimize the join performance and deliver rapid query results.
Example: Using Spark and FiloDB for Sub-Second Joins
import com.typesafe.config.Config;
import com.typesafe.config.ConfigFactory;
import filodb.spark.FiloDriver;
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import org.apache.spark.sql.SparkSession;
public class SparkFiloDBJoinExample {
public static void main(String[] args) {
// Create a Spark Session
SparkSession spark = SparkSession.builder()
.appName("SparkFiloDBJoinExample")
.master("local")
.getOrCreate();
// Load data from FiloDB into a DataFrame
Config filoConfig = ConfigFactory.load("filodb-configuration");
FiloDriver.init(filoConfig);
Dataset<Row> filoDF = spark.read().format("filodb.spark").option("database", "sampleDB").option("dataset", "sampleDataset").load();
// Perform join operation with another DataFrame using Spark
Dataset<Row> joinedDf = ...; // Perform join with another DataFrame using Spark
// Show the results
joinedDf.show();
}
}
In this example, we initialize FiloDB configuration, load data into a DataFrame using the FiloDB Spark connector, and then perform a join operation with another DataFrame using Spark's distributed processing capabilities. The combined prowess of Spark and FiloDB culminates in efficient and lightning-fast join operations, enabling sub-second query times even for large-scale datasets.
The Last Word
In the world of big data analytics, achieving rapid query performance is a paramount objective. By harnessing the capabilities of Apache Spark and FiloDB, it becomes possible to accomplish sub-second join times, even when dealing with immense volumes of data. This powerful combination of a high-performance processing engine and an efficient columnar store opens up new possibilities for real-time analytics and insights, empowering businesses to extract value from their data with unprecedented speed and efficiency.
By adopting a strategy that integrates Spark and FiloDB, organizations can elevate their data processing capabilities to new heights, gaining a competitive edge in the dynamic landscape of data-driven decision-making.
In conclusion, the marriage of Apache Spark and FiloDB paves the way for lightning-fast query times and unlocks the full potential of big data analytics. Embrace this powerful duo and propel your data processing performance to the next level.