Overcoming JCLAP Parsing Issues in Java CLI Apps
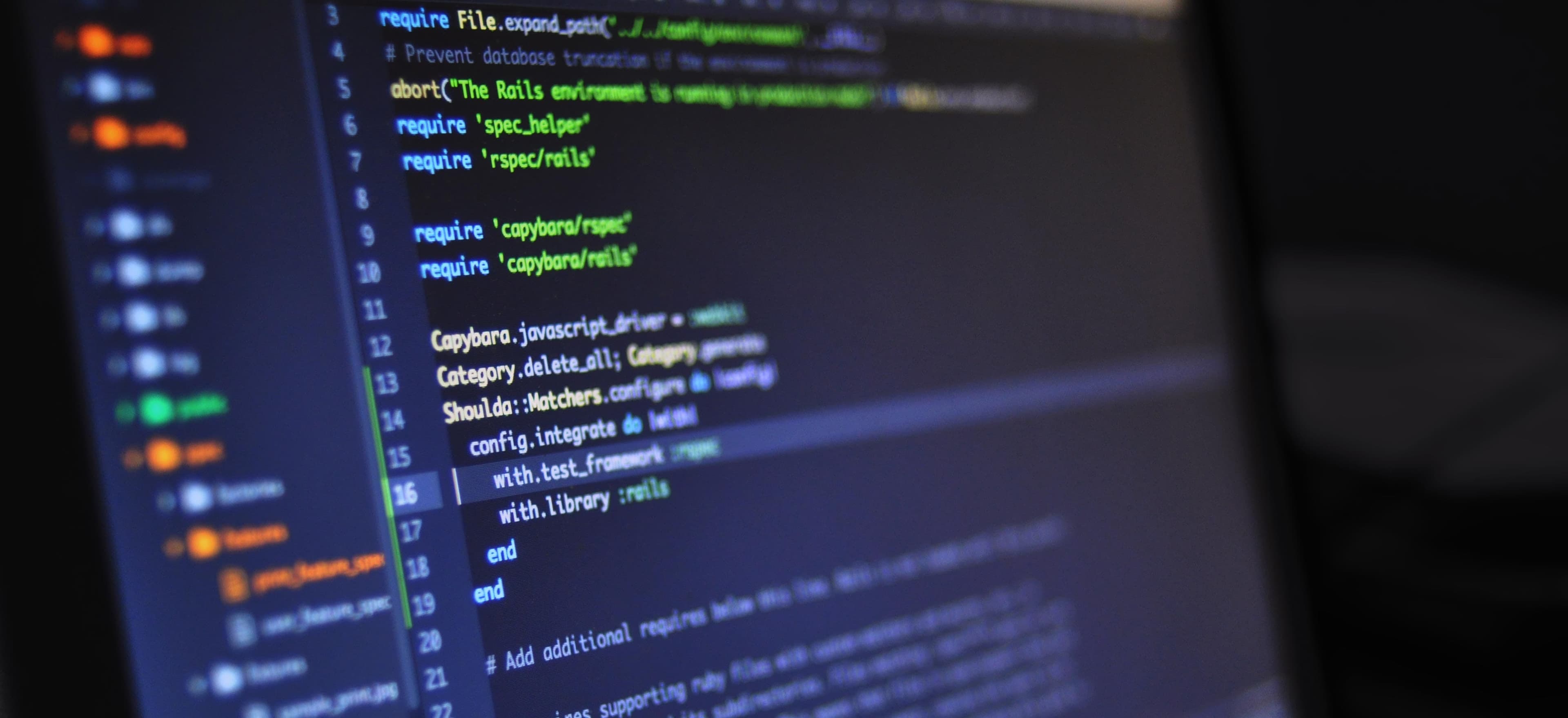
- Published on
Understanding JCLAP and Parsing Issues in Java CLI Apps
In the world of Java application development, Command Line Interface (CLI) apps play an indispensable role. They allow users to interact with software directly through the terminal, providing a seamless and efficient way to execute commands and perform tasks. However, building CLI apps in Java comes with its own set of challenges, particularly when it comes to parsing command-line arguments effectively.
One popular library for handling command-line parsing in Java is JCLAP (Java Command-Line Argument Parser). While JCLAP simplifies the process of defining and parsing command-line arguments, developers often encounter issues related to parsing and handling various types of inputs. In this article, we will explore common parsing issues in Java CLI apps using JCLAP and discuss strategies to overcome them.
Understanding JCLAP
Before diving into parsing issues, let's take a moment to understand the basics of JCLAP. JCLAP is a lightweight and easy-to-use library for parsing command-line arguments in Java. It allows developers to define command-line options, arguments, and flags using a simple and intuitive API.
Here's a quick example of how JCLAP can be used to define command-line options:
import org.clap.*;
public class MyApp {
public static void main(String[] args) {
CliParser parser = new CliParser("MyApp");
parser.addOption(new BooleanOption("verbose", "Produce verbose output"));
parser.addOption(new StringOption("input", "Path to input file", true));
try {
parser.parse(args);
boolean verbose = parser.getBooleanValue("verbose");
String inputFilePath = parser.getStringValue("input");
// Use the parsed values to perform application logic
} catch (CliParseException e) {
System.err.println("Error parsing command-line arguments: " + e.getMessage());
// Handle parsing errors
}
}
}
In this example, we define two command-line options: verbose
and input
. We then parse the command-line arguments using JCLAP and retrieve the values of the parsed options.
Common Parsing Issues
Despite its simplicity, developers often encounter parsing issues when using JCLAP in their Java CLI apps. Some of the common issues include:
1. Handling Input Types
JCLAP provides basic support for common data types such as String
, int
, boolean
, etc. However, parsing custom or complex data types (e.g., date formats, custom objects) can become challenging.
2. Validation and Constraints
Validating and applying constraints to parsed values is crucial for ensuring the correctness of inputs. JCLAP offers limited support for defining validation rules and constraints on command-line options.
3. Error Handling and User Feedback
Providing informative error messages and user feedback in case of parsing errors is essential for a smooth user experience. JCLAP's default error messages may not always be sufficient or user-friendly.
Strategies to Overcome Parsing Issues
Now that we've identified the common parsing issues, let's discuss strategies to overcome these challenges when using JCLAP in Java CLI apps.
1. Custom Type Converters
To handle custom or complex data types, we can implement custom type converters in JCLAP. By extending the Converter
class provided by JCLAP, we can define how to parse and convert custom input types. This allows us to seamlessly integrate complex data types into our CLI apps.
public class CustomDateConverter implements Converter<Date> {
public Date convert(String input) throws ConversionException {
// Implement custom conversion logic
}
}
2. Custom Validation and Constraints
To enforce validation rules and constraints on command-line options, we can create custom validators in JCLAP. By extending the Validator
class, we can define custom validation logic for various types of inputs, empowering us to ensure the correctness of parsed values.
public class CustomRangeValidator implements Validator<Integer> {
public void validate(Integer value) throws ValidationException {
if (value < 0 || value > 100) {
throw new ValidationException("Value must be between 0 and 100");
}
}
}
3. Custom Error Handling and User Feedback
JCLAP allows us to customize error handling and user feedback by providing custom error messages and exception handling. By catching and handling CliParseException
instances, we can provide meaningful error messages and guidance to users when parsing issues occur.
try {
parser.parse(args);
} catch (CliParseException e) {
System.err.println("Error parsing command-line arguments: " + e.getMessage());
System.err.println("Use --help for usage information");
System.exit(1);
}
A Final Look
In conclusion, JCLAP is a powerful tool for parsing command-line arguments in Java CLI apps. By understanding the common parsing issues and employing the strategies discussed in this article, developers can overcome these challenges and enhance the parsing capabilities of their CLI apps. Whether it's handling custom data types, enforcing validation rules, or providing user-friendly error messages, JCLAP can be effectively utilized to build robust and user-friendly Java CLI apps.
By leveraging custom type converters, validators, and error handling mechanisms, developers can elevate the parsing experience in their Java CLI apps, ultimately enhancing the usability and reliability of their command-line interfaces. With these strategies in place, parsing issues should no longer be a hurdle in the development of Java CLI apps using JCLAP.
As you delve into the world of Java CLI app development, remember that mastering the art of parsing command-line arguments is pivotal for creating seamless and efficient user experiences in the terminal.
Happy coding!
By addressing the parsing issues and offering powerful strategies for enhancing parsing capabilities in Java CLI apps using JCLAP, this article provides valuable insights for developers seeking to overcome command-line parsing challenges. Whether it's handling custom data types, enforcing validation rules, or providing user-friendly error messages, the strategies outlined empower developers to build robust and user-friendly Java CLI apps. If you're looking to enhance your CLI app development skills, mastering the art of parsing command-line arguments is pivotal for creating seamless and efficient user experiences in the terminal.
Checkout our other articles