Solving Docker REST API Issues for Java Developers
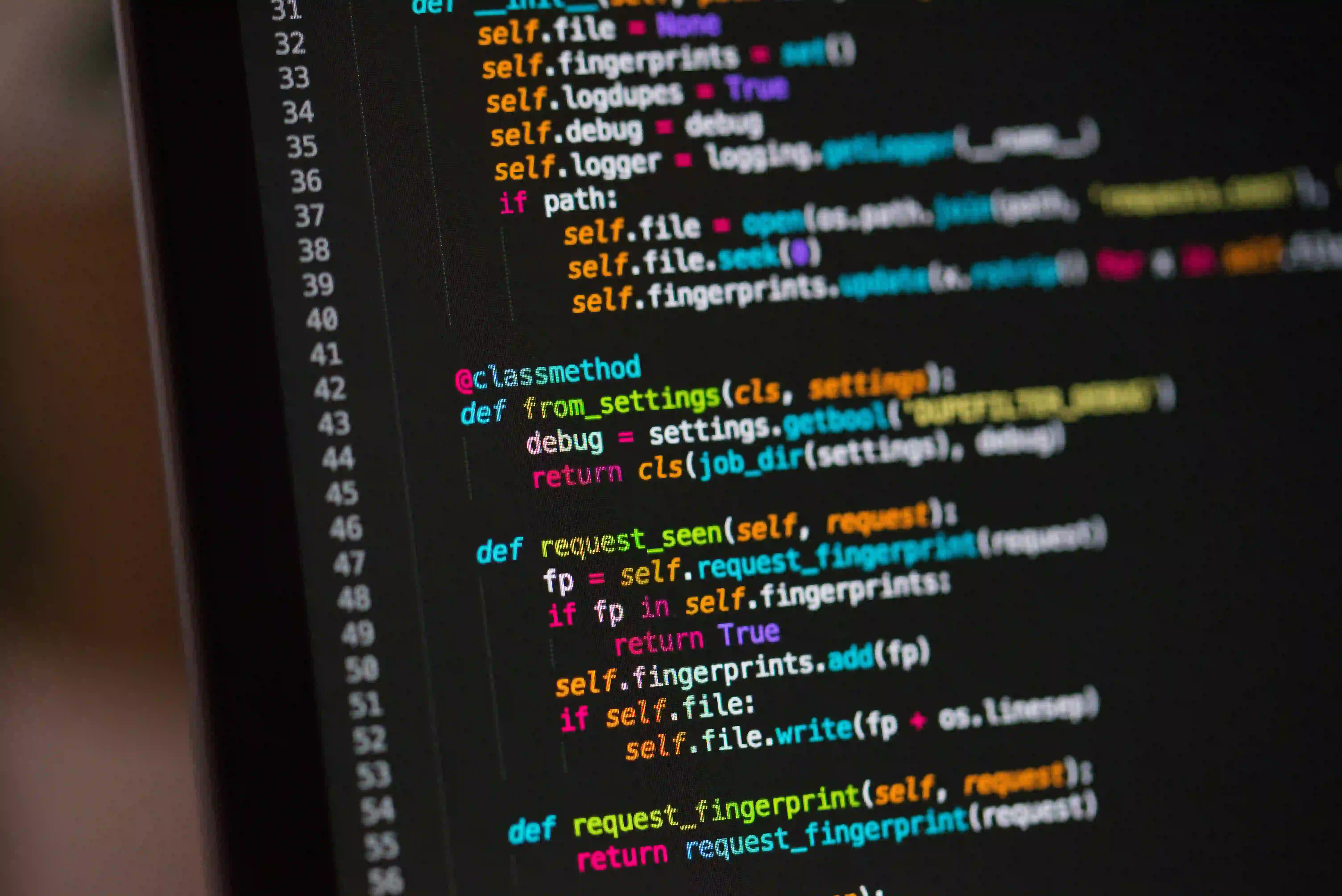
Troubleshooting Docker REST API Issues for Java Developers
As a Java developer, working with Docker provides countless benefits, such as containerization, efficient resource utilization, and simplified application deployment. However, when integrating Docker with Java applications through the REST API, you may encounter challenges. This blog post will guide you through troubleshooting common Docker REST API issues and provide solutions for seamless integration with your Java projects.
Issue 1: Connection Refused
One of the most common issues when interacting with the Docker REST API from a Java application is encountering "connection refused" errors. This error typically occurs when the Java application fails to establish a connection with the Docker daemon.
Solution:
To resolve this issue, ensure that the Docker daemon is running and accessible. Additionally, check if the Java application is using the correct URL to communicate with the Docker daemon.
Here's an example of establishing a connection to the Docker REST API using the docker-java
library:
DockerClientConfig config = DefaultDockerClientConfig.createDefaultConfigBuilder().build();
DockerHttpClient client = new ApacheDockerHttpClient.Builder()
.dockerHost(config.getDockerHost())
.sslConfig(config.getSSLConfig())
.build();
DockerClient dockerClient = DockerClientImpl.getInstance(config, client);
In this code snippet, we use the docker-java
library to create a connection to the Docker daemon. It's essential to ensure that the dockerHost
configuration points to the correct URL where the Docker daemon is running.
Issue 2: Unauthorized Access
Another common issue is encountering "unauthorized" or "permission denied" responses when making requests to the Docker REST API from a Java application. This error indicates that the Java application lacks the necessary permissions to perform the requested operation.
Solution:
To address unauthorized access issues, verify that the Java application has the required permissions to communicate with the Docker daemon. This may involve granting the application specific privileges or ensuring that it runs with appropriate credentials.
When using the docker-java
library, you can provide authentication credentials to authenticate with the Docker daemon:
DockerClientConfig config = DefaultDockerClientConfig.createDefaultConfigBuilder()
.withDockerHost("tcp://docker.example.com:2376")
.withDockerConfig("src/main/resources/.docker/")
.withDockerTlsVerify("1")
.withDockerCertPath("/Users/username/.docker/certs")
.withRegistryUsername("username")
.withRegistryPassword("password")
.build();
DockerHttpClient client = new ApacheDockerHttpClient.Builder()
.dockerHost(config.getDockerHost())
.sslConfig(config.getSSLConfig())
.build();
DockerClient dockerClient = DockerClientImpl.getInstance(config, client);
In this example, the withRegistryUsername
and withRegistryPassword
methods are used to provide authentication credentials when establishing a connection to the Docker daemon. Ensure that the credentials are valid and have the necessary permissions to access Docker resources.
Issue 3: Handling SSL/TLS Certificates
When communicating with the Docker REST API over HTTPS, SSL/TLS certificates play a crucial role. Failure to handle certificates appropriately can result in SSL handshake errors or security-related exceptions in your Java application.
Solution:
To effectively handle SSL/TLS certificates when interacting with the Docker REST API, ensure that the Java application is configured to trust the Docker daemon's certificate. This involves providing the necessary certificate files and configuring the Java application to use them for secure communication.
The docker-java
library allows you to configure SSL/TLS properties for secure communication with the Docker daemon. Here's an example of setting up SSL/TLS configuration:
DockerClientConfig config = DefaultDockerClientConfig.createDefaultConfigBuilder()
.withDockerHost("https://docker.example.com:2376")
.withDockerConfig("src/main/resources/.docker/")
.withDockerTlsVerify("1")
.withDockerCertPath("/Users/username/.docker/certs")
.build();
DockerHttpClient client = new ApacheDockerHttpClient.Builder()
.dockerHost(config.getDockerHost())
.sslConfig(config.getSSLConfig())
.build();
DockerClient dockerClient = DockerClientImpl.getInstance(config, client);
In this code snippet, the withDockerHost
, withDockerTlsVerify
, and withDockerCertPath
methods are used to specify the Docker daemon's HTTPS endpoint and the path to the SSL/TLS certificate files. By providing the correct certificate files and configuration, the Java application can establish a secure connection with the Docker daemon.
The Bottom Line
Integrating Docker with Java applications through the REST API can significantly enhance the efficiency and scalability of your software projects. By addressing common issues such as connection errors, unauthorized access, and SSL/TLS certificate handling, Java developers can ensure smooth communication with Docker containers.
In summary, when working with the Docker REST API from a Java application:
- Verify the Docker daemon's availability and accessibility.
- Authenticate with the Docker daemon using appropriate credentials.
- Configure SSL/TLS properties for secure communication.
By following these best practices and leveraging the docker-java
library, Java developers can troubleshoot Docker REST API issues effectively and unlock the full potential of containerized environments for their applications.
For further exploration of Docker REST API integration in Java, consider exploring the official Docker Java client library and the Docker API documentation. These resources provide valuable insights and additional support for seamless Docker integration in Java applications.
Happy coding!
By adhering to best practices and leveraging the docker-java
library, Java developers can effectively troubleshoot Docker REST API issues and ensure seamless integration with their applications. For further exploration, consider referring to the official Docker Java client library and the Docker API documentation.