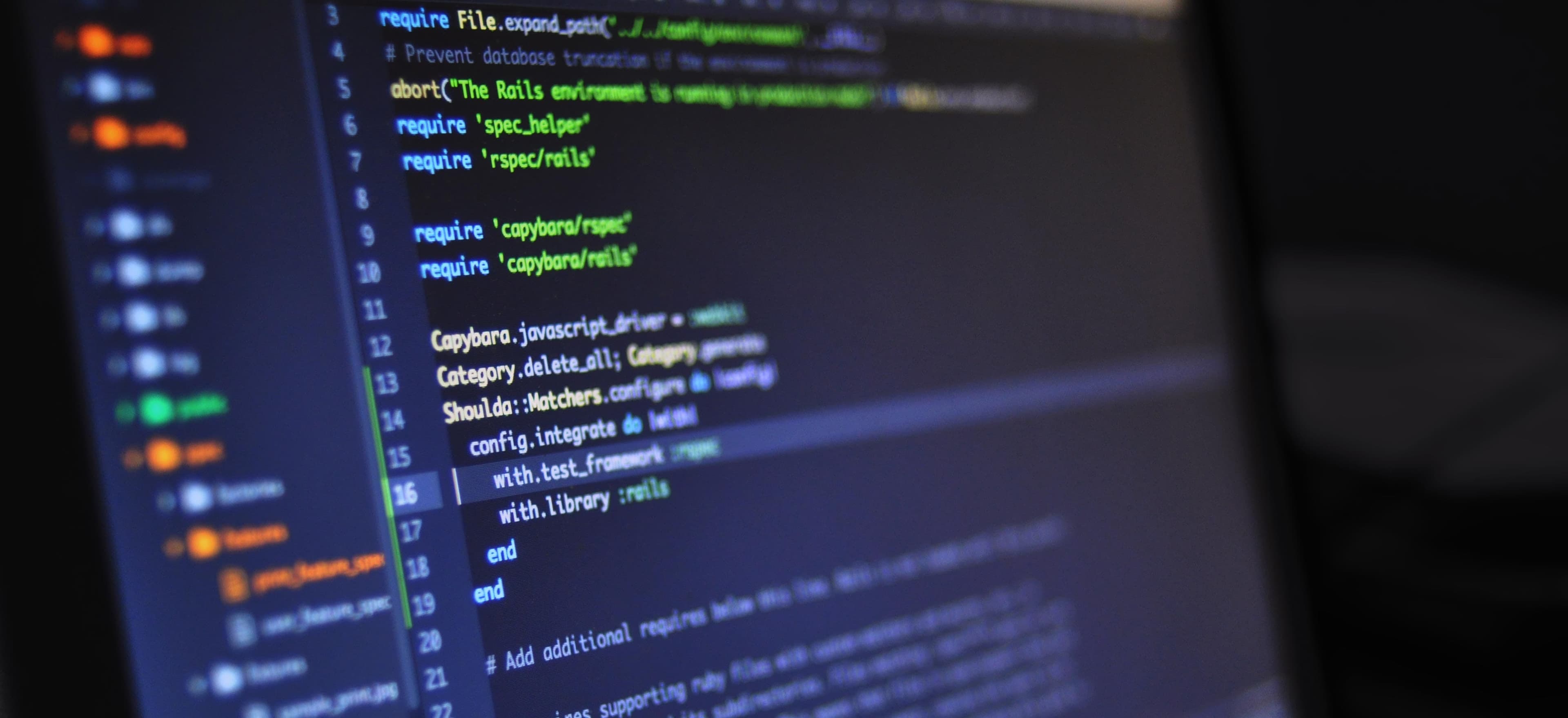
- Published on
Mastering User Authentication in Jakarta EE Applications
User authentication is a critical component of any web application. Jakarta EE provides a robust set of tools and APIs for implementing secure user authentication. In this blog post, we will explore the best practices for implementing user authentication in Jakarta EE applications.
Understanding User Authentication
User authentication is the process of verifying that someone is who they claim to be. In web applications, this typically involves prompting the user to provide some form of credentials, such as a username and password, and then validating those credentials against a known identity store, such as a database or LDAP directory.
Using JAX-RS for User Authentication
Jakarta EE applications commonly use JAX-RS for building RESTful APIs. When it comes to user authentication, JAX-RS provides a convenient way to secure endpoints using annotations such as @RolesAllowed
and @DenyAll
.
Here's an example of a JAX-RS endpoint secured with user roles:
@Path("/secured")
@RolesAllowed("ADMIN")
@GET
public Response getSecureData() {
// Implementation
}
In this example, the @RolesAllowed
annotation ensures that only users with the "ADMIN" role can access the getSecureData
endpoint.
Leveraging Jakarta Security API
Jakarta EE provides a powerful Security API that can be used to implement custom authentication and authorization logic. The javax.security.enterprise
package includes classes and interfaces for building custom authentication mechanisms, such as HttpAuthenticationMechanism
and IdentityStore
.
Let's take a look at a basic custom HttpAuthenticationMechanism
:
@ApplicationScoped
public class CustomAuthenticationMechanism implements HttpAuthenticationMechanism {
@Inject
private IdentityStore identityStore;
@Override
public AuthenticationStatus validateRequest(HttpServletRequest request, HttpServletResponse response, HttpMessageContext httpMessageContext) throws AuthenticationException {
// Custom authentication logic
}
}
In this example, we inject an IdentityStore
to validate the user's credentials. The validateRequest
method is where custom authentication logic can be implemented.
Implementing Custom Identity Store
The IdentityStore
interface is used to interact with an identity store, such as a database or an LDAP directory. Implementing a custom IdentityStore
allows for seamless integration with existing user management systems.
@ApplicationScoped
public class CustomIdentityStore implements IdentityStore {
@Override
public CredentialValidationResult validate(UsernamePasswordCredential usernamePasswordCredential) {
// Custom validation logic
}
@Override
public Set<ValidationType> validationTypes() {
return Collections.singleton(ValidationType.VALIDATE);
}
}
In this example, the validate
method is where custom validation logic for user credentials is implemented.
Utilizing JSON Web Tokens (JWT) for Stateless Authentication
JSON Web Tokens are a popular choice for implementing stateless authentication in web applications. Jakarta EE provides support for JWT through libraries like smallrye-jwt
for generating and validating tokens.
Here's an example of generating a JWT token using the smallrye-jwt
library:
import io.smallrye.jwt.build.Jwt;
import org.eclipse.microprofile.jwt.Claims;
String token = Jwt.issuer("https://myapp.com") // Issuer
.subject("jdoe") // Subject
.upn("jdoe@example.com") // UPN
.claim(Claims.birthdate.name(), "2001-07-13") // Custom claim
.expiresAt(Instant.now().plusSeconds(300)) // Expiration
.sign();
In this example, we create a JWT token with issuer, subject, user principal name, custom claim, and expiration.
The Bottom Line
User authentication is a critical aspect of building secure Jakarta EE applications. By leveraging the tools and APIs provided by Jakarta EE, developers can implement robust and secure user authentication mechanisms. From using JAX-RS annotations for role-based access control to implementing custom authentication mechanisms and leveraging JSON Web Tokens for stateless authentication, Jakarta EE offers a comprehensive set of features for mastering user authentication in web applications.
In summary, mastering user authentication in Jakarta EE applications involves understanding the various APIs and tools available, implementing custom authentication and identity store logic, and leveraging state-of-the-art technologies like JSON Web Tokens for secure and efficient authentication.
By following best practices and staying updated on the latest security features in Jakarta EE, developers can ensure the highest level of security for their applications while providing a seamless and user-friendly authentication experience.
For further reading, refer to the official Jakarta EE documentation and the MicroProfile JWT specification.
Remember, user authentication is not a one-time implementation, but an ongoing process that requires continuous improvement and adaptation to ensure the security of your Jakarta EE applications.