Breaking Singleton: Common Pitfalls & How to Avoid Them
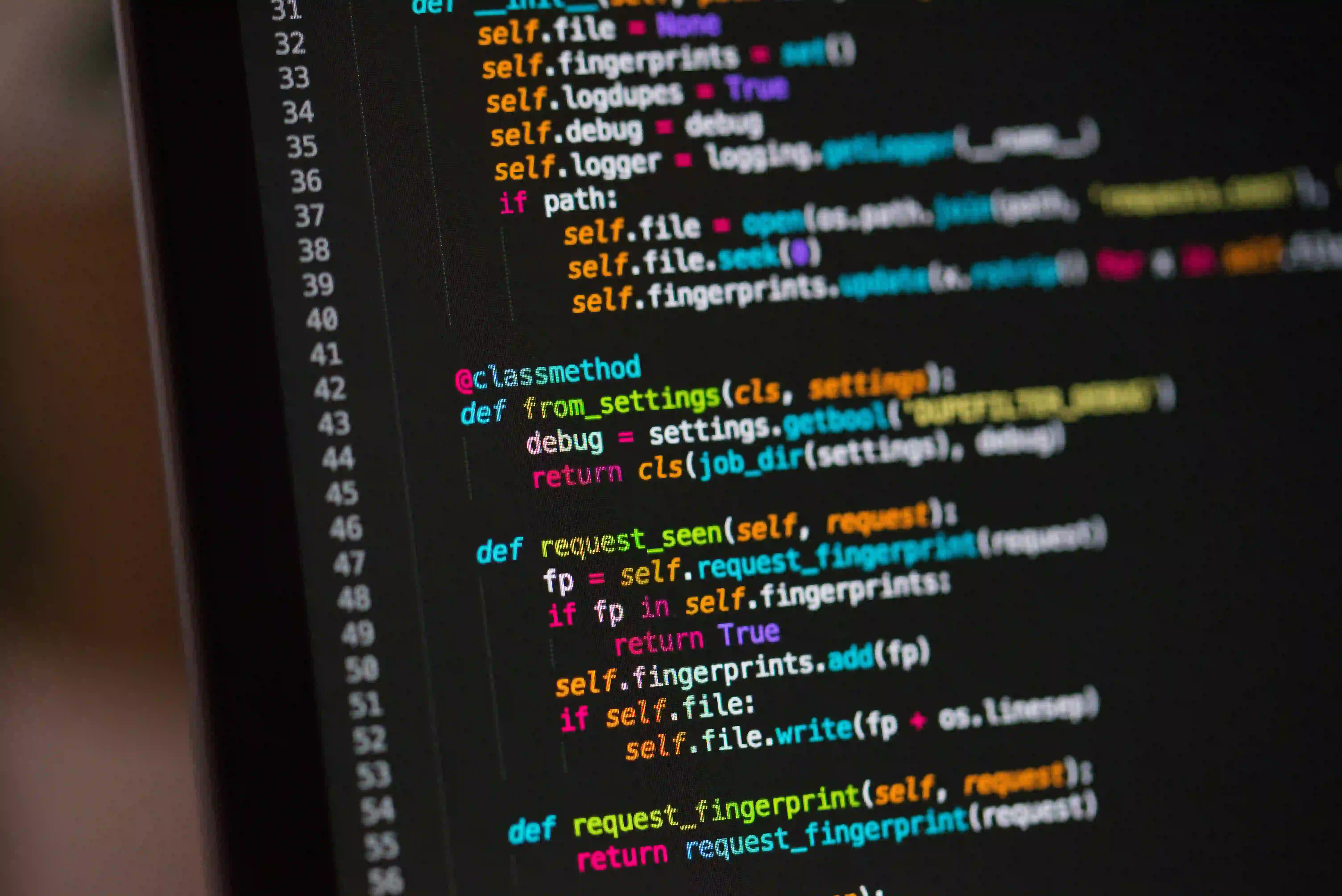
Breaking Singleton: Common Pitfalls & How to Avoid Them
Singleton pattern, a popular creational design pattern, ensures that a class has only one instance and provides a global point of access to that instance. However, due to its widespread use, there are common pitfalls that developers might encounter, potentially breaking the singleton pattern. In this article, we'll explore these pitfalls and discuss best practices to avoid them.
Pitfall 1: Multiple Instantiations
One of the most common pitfalls is allowing multiple instantiations of a singleton class. This defeats the purpose of the singleton pattern and can lead to unexpected behavior in the application.
Let's take a look at an example:
public class Singleton {
private static Singleton instance;
private Singleton() {
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
In the above code, the getInstance
method does not handle multi-threading, which can lead to multiple instantiations in a multi-threaded environment. To avoid this, we can use the double-checked locking mechanism or initialize the instance during class loading.
public class Singleton {
private static Singleton instance = new Singleton();
private Singleton() {
}
public static Singleton getInstance() {
return instance;
}
}
By initializing the instance during class loading, we ensure that only one instance is created, regardless of the multi-threading environment.
Pitfall 2: Serialization
Another common pitfall is when a serialized singleton class is deserialized, it creates a new instance, again violating the singleton pattern.
To address this, we can implement the readResolve
method to enforce the singleton behavior during deserialization.
public class Singleton implements Serializable {
private static final long serialVersionUID = 1L;
private static Singleton instance;
private Singleton() {
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
protected Object readResolve() {
return getInstance();
}
}
The readResolve
method ensures that the deserialized object is discarded in favor of the singleton instance obtained via the getInstance
method.
Pitfall 3: Reflection
Using reflection, it's possible to bypass the singleton pattern and create multiple instances of the singleton class.
public class Main {
public static void main(String[] args) {
Singleton instance1 = Singleton.getInstance();
try {
Constructor<Singleton> constructor = Singleton.class.getDeclaredConstructor();
constructor.setAccessible(true);
Singleton instance2 = constructor.newInstance();
} catch (Exception e) {
e.printStackTrace();
}
}
}
To prevent this, we can modify the constructor to throw an exception if an instance already exists, thus ensuring the singleton pattern is not violated.
public class Singleton {
private static Singleton instance;
private Singleton() {
if (instance != null) {
throw new RuntimeException("Use getInstance() method to get the single instance of this class.");
}
}
public static Singleton getInstance() {
if (instance == null) {
instance = new Singleton();
}
return instance;
}
}
The Closing Argument
In conclusion, while the singleton pattern is a powerful tool, it's crucial to be aware of these common pitfalls and take necessary precautions to avoid them. By addressing multi-threading, serialization, and reflection, we can ensure the integrity of the singleton pattern in our Java applications.
Remember, a well-implemented singleton pattern can help manage resources efficiently and provide a single point of access across the application.
To dive deeper into design patterns and Java best practices, check out the Complete Java Masterclass and Effective Java, both of which offer valuable insights into writing robust and efficient Java code.