Overcoming SMTP StartTLS Issues in Java and Android
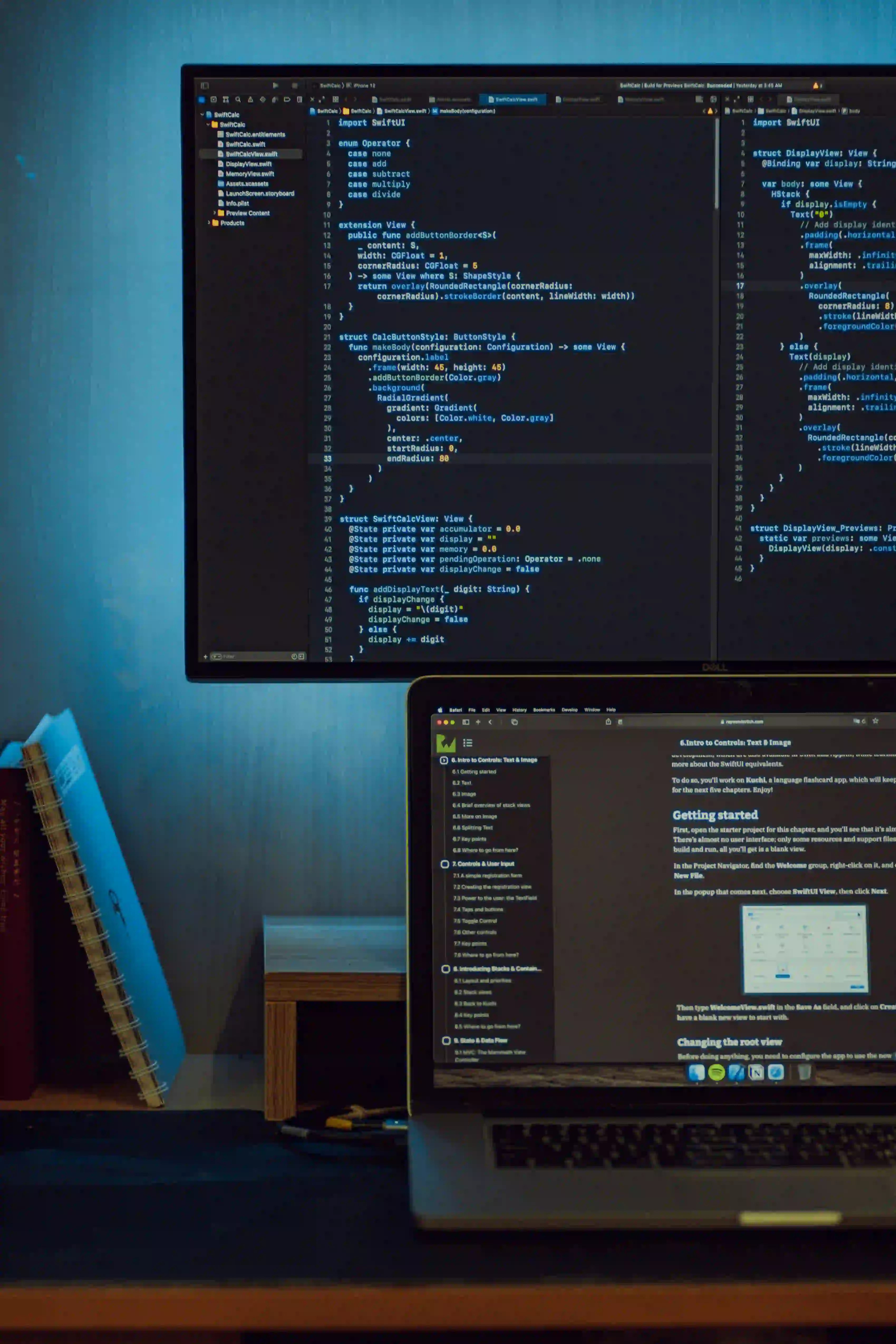
Overcoming SMTP StartTLS Issues in Java and Android
When working with email in Java and Android, you might encounter issues with sending emails over a secure connection using the StartTLS protocol. StartTLS issues can often be frustrating to deal with, but with the right knowledge and approach, they can be overcome. In this blog post, we will explore how to effectively handle SMTP StartTLS issues in Java and Android, providing solutions and best practices for a seamless email sending experience.
Understanding StartTLS
Before diving into troubleshooting StartTLS issues, it's important to have a solid understanding of what StartTLS is and how it is used in the context of email communication.
StartTLS is a protocol extension for upgrading a plain text connection to an encrypted (TLS or SSL) connection. In the case of email, StartTLS is commonly used to secure the communication between email clients or servers and the SMTP (Simple Mail Transfer Protocol) server. This ensures that emails are transmitted securely over an encrypted channel, preventing unauthorized access and tampering.
Common StartTLS Issues
When working with StartTLS in Java and Android, you may encounter several common issues, including:
- Handshake Failures: The handshake process between the client and the server fails, preventing the StartTLS upgrade.
- Unsupported Cipher Suites: The client and server are unable to negotiate compatible cipher suites for establishing a secure connection.
- Truststore and Keystore Configuration: Incorrect configuration of truststore and keystore files can lead to StartTLS failures.
- Hostname Verification: Failure to properly verify the hostname in the server's SSL certificate can result in StartTLS issues.
Handling StartTLS Issues in Java
To effectively handle StartTLS issues in Java, it's essential to leverage the JavaMail API, which provides a powerful and flexible framework for sending and receiving emails. Let's delve into some practical solutions for addressing common StartTLS issues in Java.
Resolving Handshake Failures
One common cause of handshake failures when initiating a StartTLS connection is the use of outdated TLS/SSL protocols or cipher suites. To address this, it's crucial to ensure that your Java application uses modern and secure protocols and cipher suites for SSL/TLS connections.
Here's an example of configuring the supported protocols and cipher suites in the JavaMail session:
Properties props = new Properties();
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.ssl.protocols", "TLSv1.2");
props.put("mail.smtp.ssl.ciphersuites", "TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256");
Session session = Session.getInstance(props, null);
In this example, we explicitly set the supported protocol to TLSv1.2 and the preferred cipher suite to TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256. By doing so, we ensure that our application is using strong encryption protocols and cipher suites compatible with modern security standards.
Managing Truststore and Keystore Configuration
The proper configuration of truststore and keystore files is crucial for establishing secure connections using StartTLS. The truststore contains trusted certificates from Certificate Authorities, while the keystore holds the application's own digital certificates and private keys.
props.put("mail.smtp.ssl.trust", "smtp.server.com"); // Use the server's hostname
props.put("mail.smtp.ssl.keystore", "/path/to/keystore");
props.put("mail.smtp.ssl.keystore.password", "keystore_password");
props.put("mail.smtp.ssl.key", "/path/to/private/key");
props.put("mail.smtp.ssl.key.password", "key_password");
In the code snippet above, we specify the truststore, keystore, and corresponding passwords required for the SSL/TLS connection. By ensuring the correct configuration of these properties, we can mitigate StartTLS issues stemming from truststore and keystore misconfigurations.
Hostname Verification
Proper hostname verification is essential for ensuring the authenticity of the server's SSL certificate during the StartTLS handshake. Failure to perform hostname verification can lead to security vulnerabilities and potential man-in-the-middle attacks.
To enable hostname verification in JavaMail, we can use the following code snippet:
props.put("mail.smtp.ssl.checkserveridentity", "true");
Enabling the mail.smtp.ssl.checkserveridentity
property ensures that the server's hostname in the SSL certificate is verified during the StartTLS negotiation, thereby enhancing the security of the email communication.
Addressing StartTLS Issues in Android
In Android, handling StartTLS issues for email sending involves similar principles as those applied in Java, with additional considerations specific to the Android platform. Let's explore practical strategies for addressing StartTLS issues in the context of Android application development.
SSL/TLS Configuration in AndroidManifest.xml
When working with StartTLS in an Android application, it's important to specify the SSL/TLS configuration in the AndroidManifest.xml
file. This includes defining the minimum and maximum supported TLS versions and the enabled cipher suites.
<application
android:networkSecurityConfig="@xml/network_security_config">
...
</application>
In the network_security_config.xml
file, you can specify the TLS version and cipher suite configurations as follows:
<network-security-config>
<base-config cleartextTrafficPermitted="false">
<trust-anchors>
<certificates src="system" />
<certificates src="user" />
</trust-anchors>
</base-config>
<domain-config>
<domain includeSubdomains="true">smtp.server.com</domain>
<trust-anchors>
<certificates src="user" />
</trust-anchors>
<pin-set>
<pin digest="SHA-256">Base64EncodedPin</pin>
</pin-set>
</domain-config>
</network-security-config>
By defining the network security configuration in the AndroidManifest.xml
and network_security_config.xml
files, you can ensure that the Android application enforces secure network communication, including StartTLS connections.
Performing StartTLS Negotiation
In an Android application, the StartTLS negotiation for email sending can be achieved using the JavaMail API in conjunction with the Android SDK. Properly configuring the SMTP session properties, as discussed earlier for Java, remains crucial for initiating the StartTLS upgrade effectively.
Here's a concise example of initiating StartTLS negotiation in an Android application using JavaMail:
Properties props = new Properties();
props.put("mail.smtp.starttls.enable", "true");
props.put("mail.smtp.ssl.protocols", "TLSv1.2");
props.put("mail.smtp.ssl.ciphersuites", "TLS_ECDHE_RSA_WITH_AES_128_GCM_SHA256");
Session session = Session.getInstance(props, null);
try {
Transport transport = session.getTransport("smtp");
transport.connect("smtp.server.com", 587, "username", "password");
MimeMessage message = new MimeMessage(session);
// Set message content, recipient, etc.
transport.sendMessage(message, message.getAllRecipients());
transport.close();
} catch (MessagingException e) {
e.printStackTrace();
}
In the code snippet above, we configure the SMTP session properties to enable StartTLS, specify the supported protocols and cipher suites, and subsequently initiate the StartTLS negotiation when sending an email from the Android application.
The Bottom Line
In summary, overcoming SMTP StartTLS issues in Java and Android involves a thorough understanding of the underlying protocols, proper SSL/TLS configuration, and effective management of truststore and keystore files. By addressing common StartTLS issues and applying best practices, you can ensure secure and reliable email communication within your Java and Android applications.
By implementing the solutions and strategies outlined in this blog post, you can confidently tackle StartTLS challenges and enhance the security of email sending in your Java and Android projects. Embracing a proactive approach to addressing StartTLS issues will not only bolster the security of your applications but also contribute to a seamless and trustworthy email communication experience for your users.
As you continue to navigate the intricacies of secure email communication in Java and Android, stay informed about the latest developments and best practices in SSL/TLS, network security, and email protocols. Armed with the knowledge and insights gained from this article, you are well-equipped to overcome StartTLS challenges and elevate the security and reliability of email transmission in your Java and Android applications.
Remember, ensuring the secure transmission of emails is paramount in today's digital landscape, and conquering StartTLS issues is a significant step toward fortifying the integrity and confidentiality of email communications in your software projects.