Unraveling Recursion: Crafting a Java Palindrome Checker
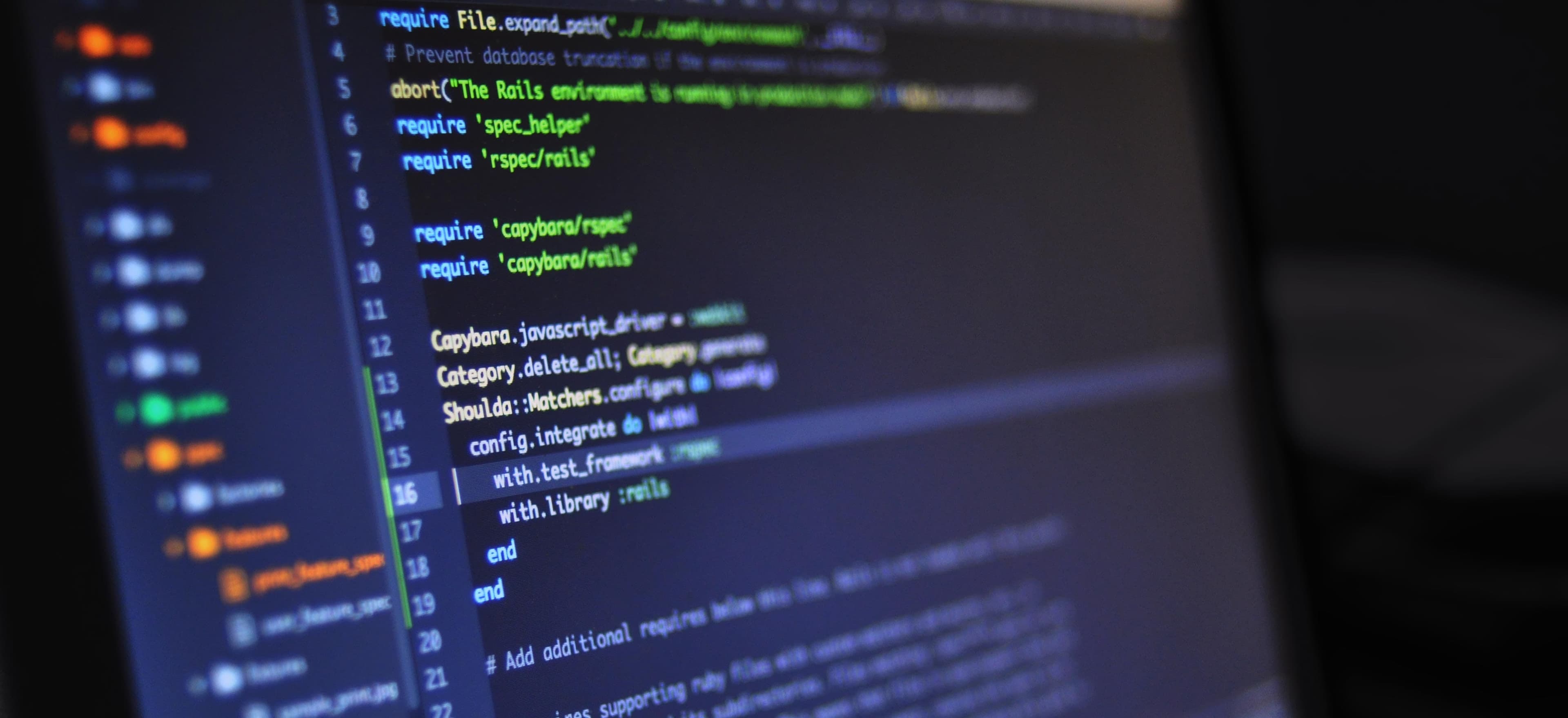
- Published on
Unraveling Recursion: Crafting a Java Palindrome Checker
Recursion is a fascinating concept in programming; it allows a function to call itself and break down complex problems into simpler sub-problems. In this blog post, we will delve deep into the magic of recursion by creating a Java program to check for palindromes. We will explore the intricacies of recursion, learn how to implement it in Java, and witness its power in action as we craft a palindrome checker.
Understanding Palindromes
Before we dive into the world of recursion, let's ensure we have a solid understanding of palindromes. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward (ignoring spaces, punctuation, and capitalization). Examples of palindromes include "radar," "level," "deified," and "12321."
Recursive Approach to Palindrome Checking
The concept of recursion involves breaking a problem down into smaller, more manageable sub-problems. In the context of palindrome checking, a recursive approach allows us to compare the first and last characters of the input string and then recursively check the remaining substring until we reach the middle of the string. This approach highlights the elegance and power of recursion in solving complex problems with minimal code.
Implementing the Palindrome Checker in Java
Let's take a deep dive into crafting a palindrome checker using Java. We will create a class called PalindromeChecker
and implement the recursive approach to determine whether a given string is a palindrome.
public class PalindromeChecker {
public static boolean isPalindrome(String s) {
// Base case: if the string has 0 or 1 characters, it is a palindrome
if (s.length() == 0 || s.length() == 1) {
return true;
}
// Compare the first and last characters, and recursively check the substring
if (s.charAt(0) == s.charAt(s.length() - 1)) {
return isPalindrome(s.substring(1, s.length() - 1));
}
// If the first and last characters do not match, the string is not a palindrome
return false;
}
}
In the isPalindrome
method, we handle the base case where a string with 0 or 1 characters is considered a palindrome. Then, we compare the first and last characters of the string. If they match, we recursively call the isPalindrome
method with the substring excluding the first and last characters. This process continues until the base case is reached, or the characters don't match, at which point we return false
.
Why Recursion?
Recursion provides an elegant and concise solution to the palindrome checking problem. By breaking down the string into smaller parts and leveraging the concept of self-referential functions, we achieve a compact and efficient implementation.
Testing the Palindrome Checker
Now that we have our PalindromeChecker
class, let's put it to the test by creating a simple test program to check various strings for palindromic properties.
public class PalindromeTester {
public static void main(String[] args) {
String[] testStrings = {"radar", "level", "deified", "hello", "12321"};
for (String s : testStrings) {
if (PalindromeChecker.isPalindrome(s)) {
System.out.println(s + " is a palindrome");
} else {
System.out.println(s + " is not a palindrome");
}
}
}
}
In the main
method of PalindromeTester
, we create an array of strings to test our PalindromeChecker
. We then iterate through each string, using the isPalindrome
method to determine whether it is a palindrome and providing the appropriate output.
Final Considerations
In this blog post, we explored the mesmerizing world of recursion by delving into the concept of palindromes and crafting a Java palindrome checker using a recursive approach. By implementing the palindrome checker in Java, we gained deeper insights into the power and elegance of recursion as a problem-solving technique.
Recursion, with its ability to break down complex problems into simpler sub-problems, has the potential to unlock new dimensions of creativity and efficiency in programming. Crafting a palindrome checker served as a compelling showcase of recursion's prowess in action.
As you continue your programming journey, remember to embrace the beauty of recursion and explore its endless possibilities in solving a myriad of computational challenges.
Take your Java skills to the next level by understanding recursion and implementing it in your programs. With a thorough understanding of recursion, you can tackle complex problems with ease and elegance. If you're keen on further enhancing your Java expertise, consider exploring more advanced topics such as data structures and algorithms.