Why Skipping Unit Tests is a Fool's Errand
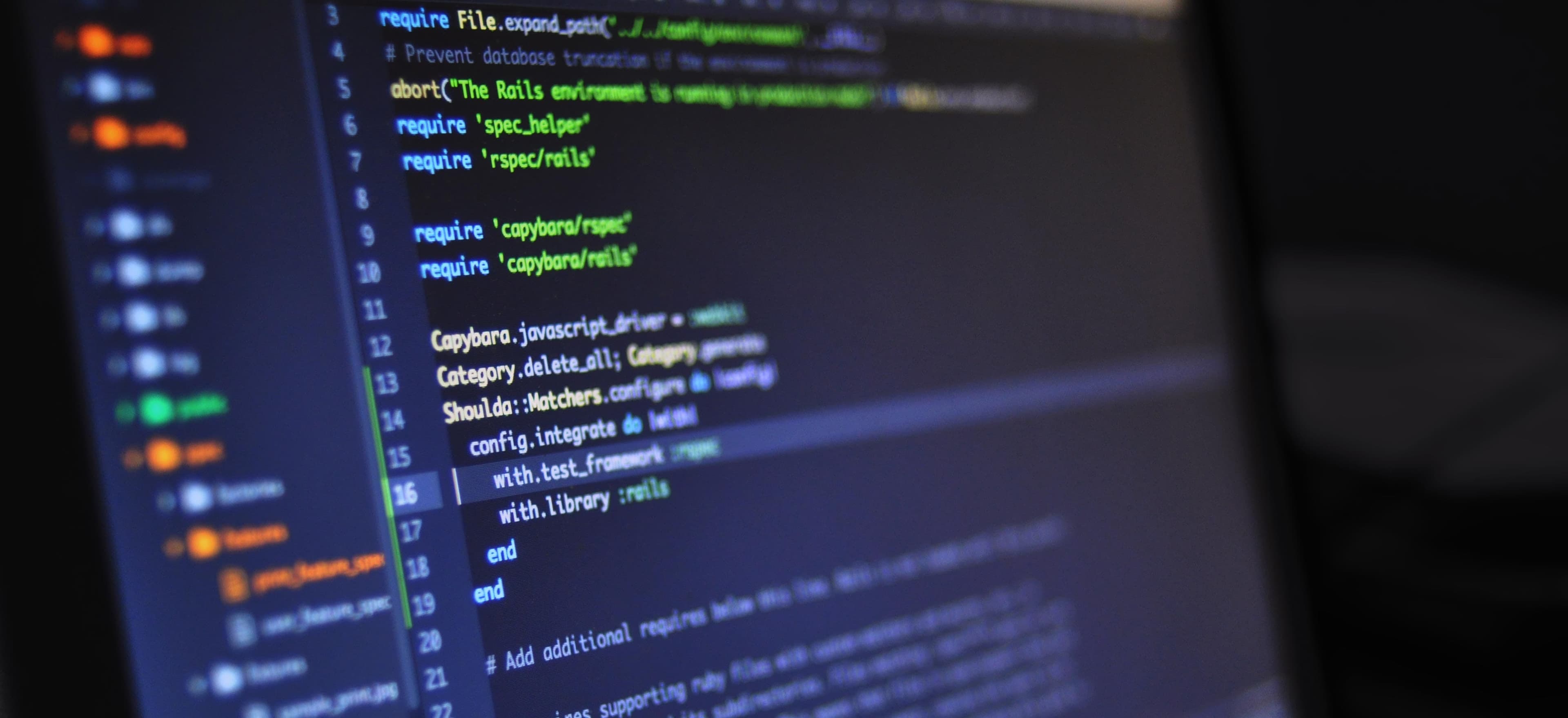
- Published on
The Importance of Unit Testing in Java
In the world of Java development, unit testing is a crucial aspect of writing high-quality, robust, and maintainable code. Unfortunately, some developers consider unit testing to be a time-consuming activity that can be skipped in favor of delivering features more quickly. However, overlooking unit tests is a fool's errand that can lead to a myriad of problems in the long run.
Understanding Unit Testing
Firstly, let's define what unit testing is. Unit testing involves testing individual units or components of a software application in isolation. In Java, a unit is typically a method or function. Unit tests are written to validate that each unit of the software performs as expected. This practice is fundamental to the Test-Driven Development (TDD) approach, where tests are written before the actual code.
The Benefits of Unit Testing
Unit testing offers a plethora of benefits that directly contribute to the overall quality and maintainability of a Java codebase. Some of the key advantages include:
1. Early Bug Detection
Unit tests allow developers to catch bugs and defects at an early stage of the development lifecycle. By uncovering issues at their inception, it becomes easier and more cost-effective to fix them. This practice significantly reduces the likelihood of defects propagating to higher levels of testing or even to production.
2. Improved Code Quality
When writing unit tests, developers are prompted to consider the expected behavior of their code in a more granular manner. This process often leads to the creation of more modular, loosely coupled, and easily understandable code. Consequently, unit testing promotes better overall code quality.
3. Facilitated Refactoring
In the world of software development, code evolves continuously. As new features are added and requirements change, the codebase needs to be refactored. Unit tests act as a safety net during refactoring. They provide the confidence to make changes and streamline the code without the fear of inadvertently introducing defects.
4. Effective Documentation
Well-written unit tests also serve as living documentation for the codebase. By examining the unit tests, developers can gain a comprehensive understanding of how various components of the system are meant to function. This can be particularly useful for newcomers to a project or those debugging unfamiliar code.
The Fallacy of Skipping Unit Tests
Despite the evident advantages, some developers are tempted to omit unit testing from their Java projects. This approach is fundamentally flawed and can lead to a range of issues:
- Increased Technical Debt
When unit tests are neglected, the overall technical debt of a project tends to increase. As changes and bug fixes are made, the absence of unit tests makes it challenging to ensure that the modifications do not inadvertently break existing functionality. Over time, this leads to a less maintainable and more error-prone codebase.
- Compromised Code Stability
Without unit tests, the stability of the codebase is compromised. Unforeseen bugs can easily find their way into the system, leading to unexpected behavior and potentially costly production incidents. As the codebase grows, the lack of unit tests further exacerbates the risk of instability.
- Reduced Productivity in the Long Run
While skipping unit tests may seem to accelerate initial development, it ultimately leads to reduced productivity in the long run. The time saved upfront is eclipsed by the additional effort required to debug, troubleshoot, and stabilize the codebase later on.
Best Practices for Unit Testing in Java
To effectively harness the benefits of unit testing in Java, it is essential to adhere to best practices. Here are some key recommendations to consider:
1. Isolate Dependencies
When writing unit tests for Java code, it's crucial to isolate dependencies such as external services, databases, and other components. By using mocking frameworks like Mockito, developers can simulate the behavior of dependencies, allowing the unit under test to be examined in isolation.
// Example of using Mockito to mock dependencies
@Test
public void testSomeMethod() {
// Create a mock
SomeDependency someDependencyMock = Mockito.mock(SomeDependency.class);
// Set up mock behavior
Mockito.when(someDependencyMock.someMethod()).thenReturn(someValue);
// Inject the mock
ClassUnderTest classUnderTest = new ClassUnderTest(someDependencyMock);
// Invoke the method under test
classUnderTest.methodUnderTest();
// Verify expected interactions
Mockito.verify(someDependencyMock).someMethod();
}
In this example, the SomeDependency
is mocked using Mockito, allowing the ClassUnderTest
to be tested independently of its actual dependencies.
2. Utilize Parameterized Tests
Parameterized tests are valuable for testing a piece of functionality with multiple input values. In Java, libraries like JUnit provide support for parameterized tests, enabling developers to run the same set of tests with different parameters.
// Example of a parameterized test in JUnit
@RunWith(Parameterized.class)
public class ParameterizedTest {
@Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][]{
{1, true},
{2, false},
{3, true}
});
}
private int input;
private boolean expectedResult;
public ParameterizedTest(int input, boolean expectedResult) {
this.input = input;
this.expectedResult = expectedResult;
}
@Test
public void testIsEven() {
assertEquals(expectedResult, SomeClass.isEven(input));
}
}
The ParameterizedTest
class demonstrates a parameterized test using JUnit. The same test logic is applied to multiple input-output pairs, ensuring comprehensive test coverage.
3. Strive for Complete Code Coverage
While achieving 100% code coverage may not always be practical or necessary, striving for comprehensive code coverage is essential. Leveraging tools like JaCoCo can aid in identifying areas of code that lack test coverage, empowering developers to enhance the overall quality of their unit tests.
// Example of using JaCoCo to measure code coverage
mvn clean test
By running the Maven command mvn clean test
with JaCoCo configured in the project, developers can generate a code coverage report, thus gaining insights into the percentage of code covered by unit tests.
Embracing a Culture of Unit Testing
Unit testing in Java is not merely an optional practice; it is a cornerstone of sound software development. By embracing unit testing, developers foster a culture of quality, stability, and maintainability within their projects. Moreover, with the use of effective testing frameworks and best practices, the process of writing and maintaining unit tests becomes more seamless and integrated into the development workflow.
In conclusion, the decision to skip unit tests in Java is a perilous one that can lead to a host of long-term challenges. By recognizing the value of unit testing and adopting industry best practices, developers can steer clear of the pitfalls associated with bypassing this critical aspect of software development. Ultimately, the investment in unit testing pays dividends in the form of robust, reliable, and evolvable Java codebases.
Checkout our other articles