Seamless IoT: Integrating Arduino with Yahoo via Temboo
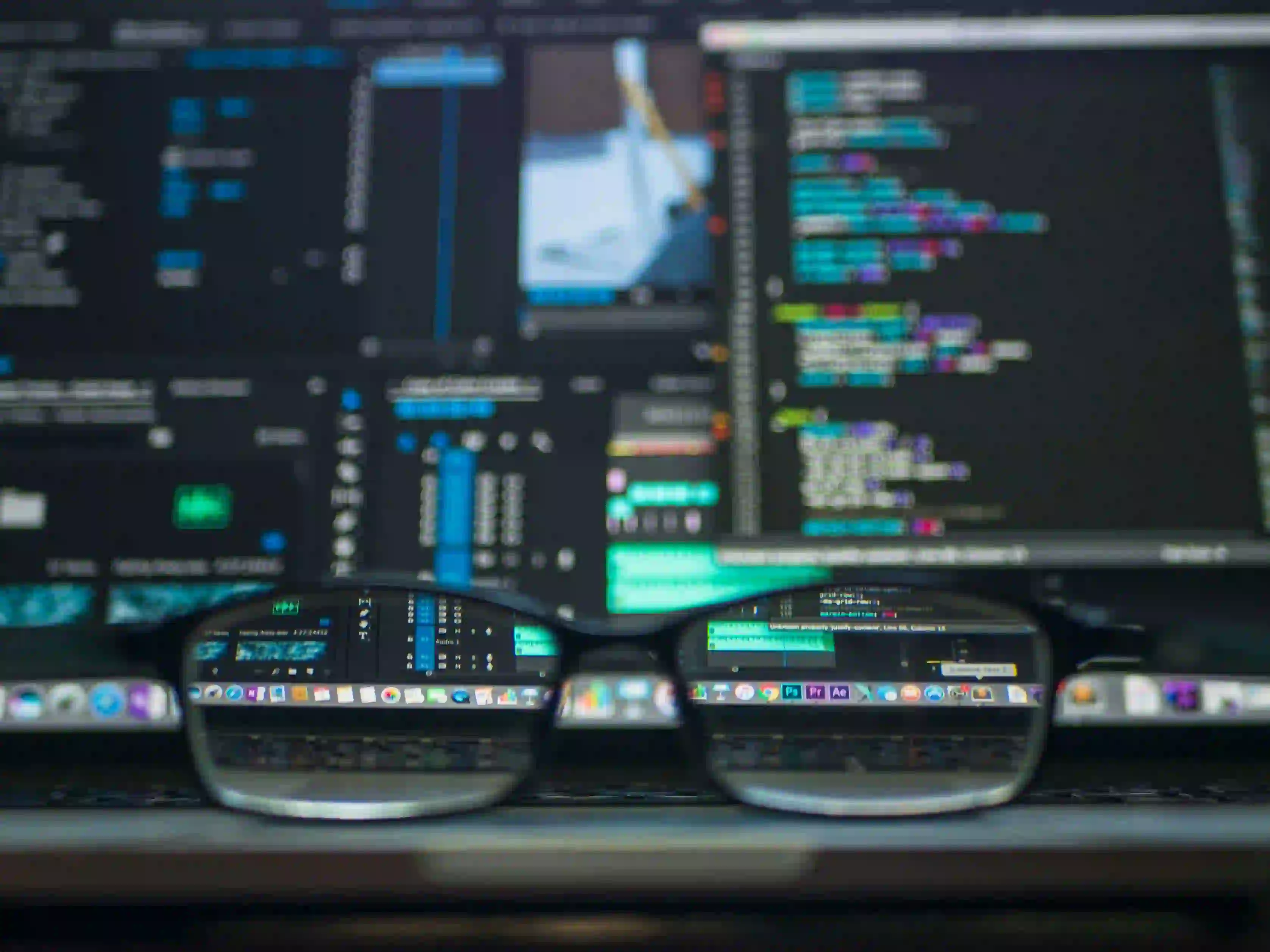
Integrating Arduino with Yahoo via Temboo for Seamless IoT
In the world of Internet of Things (IoT), integrating devices with web services is crucial for creating seamless and efficient solutions. Arduino, a popular open-source electronics platform, is widely used for building IoT projects due to its flexibility and ease of use. Yahoo, a multinational technology company, offers a range of web services that can be utilized to enhance IoT applications. Temboo, an IoT software platform, provides a bridge between Arduino and various web services, including Yahoo, making it easier to connect and interact with them. In this blog post, we will explore how to integrate Arduino with Yahoo via Temboo to create a seamless IoT solution.
Understanding the Integration
Before we delve into the technical details, let's understand the importance of integrating Arduino with Yahoo via Temboo. Yahoo's web services offer a plethora of functionalities, such as weather data, finance information, and more, which can be leveraged to enhance IoT applications. By integrating Arduino with Yahoo using Temboo, we can access and utilize these web services directly from our Arduino-based projects, enabling features like real-time weather updates, financial data retrieval, and more.
Prerequisites
To follow along with this tutorial, you will need the following:
- An Arduino board (such as Arduino Uno or Arduino Mega)
- A computer with the Arduino IDE installed
- A stable internet connection
- A Temboo account (sign up here)
- Basic knowledge of Arduino programming and web services
Getting Started with Temboo
To begin, sign in to your Temboo account and navigate to the "Library" section. Here, you will find a list of available web services, including Yahoo. Select the Yahoo service and explore the different choreos (pre-built processes) it offers, such as fetching weather data, retrieving finance information, and more. For this tutorial, let's focus on fetching weather data from Yahoo.
Accessing Yahoo Weather Data with Arduino and Temboo
Now, let's write a simple Arduino sketch to access Yahoo's weather data using Temboo. Start by creating a new sketch in the Arduino IDE and include the necessary libraries for connecting to the internet and using Temboo. The following code snippet demonstrates how to use Temboo's Yahoo Weather choreo to fetch weather data:
#include <SPI.h> // Include the SPI library for internet connectivity
#include <Ethernet.h> // Include the Ethernet library for connecting to the internet
#include <Temboo.h> // Include the Temboo library for accessing web services
// Replace with your Temboo account credentials
#define TEMBOO_ACCOUNT "your_account_name"
#define TEMBOO_APP_KEY_NAME "your_app_key_name"
#define TEMBOO_APP_KEY "your_app_key"
// Replace with your own network details
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED }; // Replace with your Ethernet shield's MAC address
IPAddress ip(192,168,1,100); // Replace with your network settings
char server[] = "api.temboo.com";
EthernetClient client;
void setup() {
// Initialize the Ethernet connection
Ethernet.begin(mac, ip);
delay(1000);
Temboo.begin(client); // Initialize Temboo
}
void loop() {
// Use the Yahoo Weather choreo to fetch weather data
Yahoo_Weather weatherChoreo; // Create an instance of the Yahoo_Weather choreo
weatherChoreo.setAccountName(TEMBOO_ACCOUNT);
weatherChoreo.setAppKeyName(TEMBOO_APP_KEY_NAME);
weatherChoreo.setAppKey(TEMBOOO_APP_KEY);
// Set inputs
weatherChoreo.setWOEID("2459115"); // Replace with your location's WOEID
// Run the choreo
weatherChoreo.run();
// Print the output to the serial monitor
while(weatherChoreo.available()) {
char c = weatherChoreo.read();
Serial.print(c);
}
}
In the above code, we first include the necessary libraries for internet connectivity, including the SPI
and Ethernet
libraries for Arduino, as well as the Temboo
library for accessing web services. We then initialize the Ethernet connection and Temboo using our account credentials. Inside the loop
function, we create an instance of the Yahoo_Weather
choreo, set our Temboo account credentials and the location's WOEID, and then run the choreo to fetch the weather data. Finally, we print the fetched weather data to the serial monitor.
Why Use Temboo for Integration?
Temboo simplifies the process of integrating Arduino with web services like Yahoo by providing a unified platform for accessing a wide range of choreos. This abstraction allows developers to focus on the functionality they want to implement (e.g., fetching weather data) without worrying about the underlying intricacies of interacting with web APIs. Additionally, Temboo's extensive documentation and pre-built code snippets for various platforms, including Arduino, make it easier to get started and accelerate the development process.
Wrapping Up
In this tutorial, we explored how to integrate Arduino with Yahoo via Temboo to access and utilize Yahoo's web services in IoT applications. By leveraging Temboo's choreos, we can seamlessly interact with Yahoo's functionalities, such as fetching weather data and retrieving finance information, directly from Arduino-based projects. This integration opens up a myriad of possibilities for creating innovative IoT solutions that leverage the power of web services. With the knowledge gained from this tutorial, you can now explore and integrate other web services with Arduino using Temboo, thereby expanding the capabilities of your IoT projects.
Integrating Arduino with Yahoo via Temboo provides a powerful combination for building sophisticated IoT applications, and with the right approach, developers can truly unlock the potential of seamless and efficient IoT solutions.