Streamline Your Java: Effortless Joining of Database Tables!
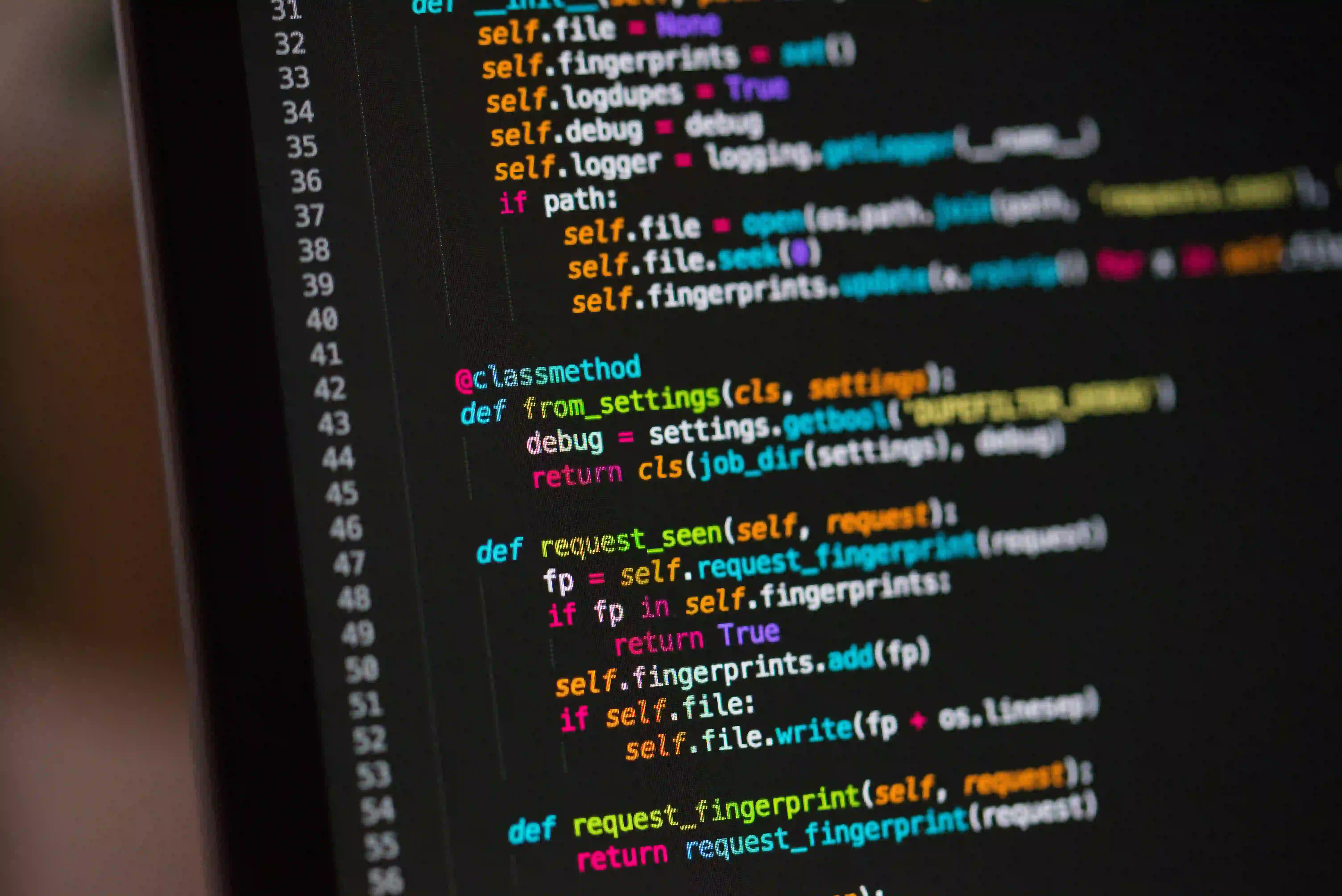
Streamline Your Java: Effortless Joining of Database Tables!
In Java programming, dealing with database tables is a common requirement. Often, there is a need to join tables to fetch the desired data. Handling this in a streamlined manner is crucial for efficient and maintainable code. In this article, we will explore how to effortlessly join database tables in Java, using the power of libraries like Hibernate and JPA.
Understanding the Need for Joining Tables
In a relational database, data is often spread across multiple tables. When fetching information, it is common to require data from multiple tables simultaneously. This is where the concept of joining tables comes into play. Joining allows us to retrieve data from related tables based on a common column or key.
Traditional Query-Based Approach
Before delving into the streamlined solutions, let's consider the traditional approach of joining tables in Java using raw SQL queries. While it certainly gets the job done, it often involves writing lengthy and complex queries. This can lead to an increase in code complexity and maintenance overhead.
Moreover, manual handling of SQL queries exposes the application to risks such as SQL injection attacks. To safeguard against such risks, additional effort needs to be invested in ensuring query parameterization and sanitation.
The Beauty of ORM: Object-Relational Mapping
This is where Object-Relational Mapping (ORM) frameworks come to the rescue. ORM frameworks such as Hibernate and JPA provide a way to map Java objects to database tables, abstracting away the complexities of SQL querying. These frameworks offer a more streamlined and object-oriented approach to database interaction.
Hibernate: Simplifying Table Joins
Let’s see how Hibernate, a widely-used ORM framework for Java, simplifies the process of joining database tables. With Hibernate’s criteria API, performing table joins becomes a breeze, allowing developers to express join conditions in a straightforward and type-safe manner.
Consider a scenario where we have two entities: Employee
and Department
. The Employee
entity has a Many-to-One association with the Department
entity. We need to fetch a list of employees along with their respective departments.
Using Hibernate’s Criteria API for Joining Tables
CriteriaBuilder builder = session.getCriteriaBuilder();
CriteriaQuery<Employee> query = builder.createQuery(Employee.class);
Root<Employee> employeeRoot = query.from(Employee.class);
// Joining the Employee entity with the Department entity
employeeRoot.join("department");
query.select(employeeRoot);
List<Employee> employees = session.createQuery(query).getResultList();
In the above code snippet, by invoking the join
method on the employeeRoot
entity, we explicitly define the join condition between the Employee
and Department
entities. This approach provides a clear and concise way of expressing the join operation.
JPA: Java Persistence API for Seamless Attribute-Based Joins
Java Persistence API (JPA), which is the standard ORM framework for Java EE, also offers a streamlined approach for joining tables. With JPA’s attribute-based approach for specifying joins, the code becomes more readable and maintainable.
Attribute-Based Joins with JPA
@Entity
public class Employee {
@Id
private Long id;
@ManyToOne
@JoinColumn(name = "department_id")
private Department department;
// Other fields and methods
}
@Entity
public class Department {
@Id
private Long id;
// Other fields and methods
}
In the Employee
entity class, the department
attribute is annotated with @ManyToOne
and @JoinColumn
annotations, establishing the relationship between Employee
and Department
entities.
CriteriaBuilder builder = entityManager.getCriteriaBuilder();
CriteriaQuery<Employee> query = builder.createQuery(Employee.class);
Root<Employee> employeeRoot = query.from(Employee.class);
// Attribute-based join between Employee and Department entities
employeeRoot.fetch("department");
query.select(employeeRoot);
List<Employee> employees = entityManager.createQuery(query).getResultList();
By using the fetch
method on the employeeRoot
, we specify the attribute-based join between the Employee
and Department
entities. This approach simplifies the process of expressing the join conditions and enhances the readability of the code.
Key Takeaways
In this article, we have explored how to effortlessly join database tables in Java using ORM frameworks such as Hibernate and JPA. By leveraging the powerful features provided by these frameworks, developers can streamline the process of joining tables, resulting in cleaner, more maintainable code.
By adopting these streamlined approaches, developers can focus on the business logic of their applications, while leaving the intricacies of database interaction to the capable hands of ORM frameworks.
So, the next time you find yourself in need of joining database tables in Java, remember the power of Hibernate and JPA to simplify this process and optimize your code for efficiency and maintainability!
For more in-depth knowledge, check out the Hibernate documentation for Criteria Queries and the Java Persistence API documentation for Attribute-Based Joins.
Happy coding!