Solving Complex Route Handling in Express.js
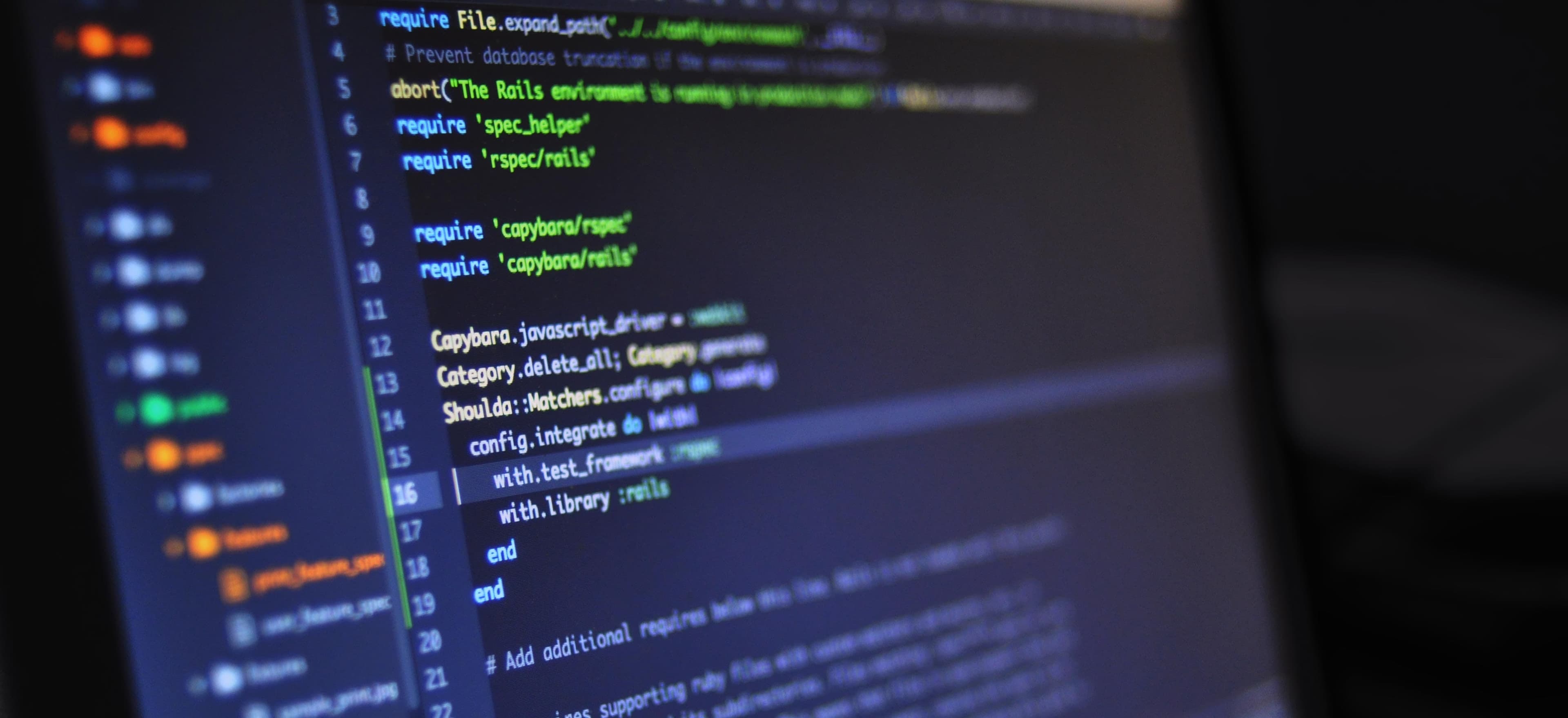
- Published on
Unraveling Complex Route Handling in Express.js to Simplify Your Web Development Journey
Express.js stands as a beacon for developers steering through the tempestuous seas of web application development. With its unopposed dexterity in building web applications and RESTful APIs, Express.js remains a preferred choice for developers. However, as one ventures deeper into the realm of developing complex applications, the initial simplicity can give way to the intricate web of route handling. Fear not, for this guide aims to demystify complex route handling in Express.js, ensuring your web development journey is both efficient and enjoyable.
The Basics: Express.js Route Handling
Express.js, a minimalist web framework for Node.js, introduces a straightforward way of handling routing. Routing refers to determining how an application responds to a client request to a specific endpoint, which is a URI (or path) and a specific HTTP request method (GET, POST, and so forth).
Here's a basic example:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Welcome to the Express.js journey!');
});
app.listen(3000, () => console.log('Server running on port 3000'));
In this example, we're creating a simple server that listens on port 3000 and responds with a welcoming message when the root URL ('/'
) is accessed. Simple, right?
Diving Deeper: Beyond Basic Route Handling
As applications grow, so does the complexity of handling routes. You may find yourself drowning in a sea of routes for handling various CRUD operations across multiple resources. This is where the real challenge begins.
Organizing Routes with express.Router
One of the most powerful features of Express.js for handling complex routing is the express.Router
class. This class allows you to create modular, mountable route handlers. By using routers, you can break down your application into smaller, more manageable pieces without losing sight of the big picture.
Consider the following example where we're handling routes for users:
const express = require('express');
const router = express.Router();
// Get all users
router.get('/users', (req, res) => {
// Logic to fetch all users
});
// Get a single user by ID
router.get('/users/:id', (req, res) => {
const { id } = req.params;
// Logic to fetch a user by ID
});
module.exports = router;
By structuring our routes this way, each part of our application has its own space to define routes related to specific resources, significantly simplifying complex route handling.
Middleware to the Rescue
Middleware functions are pivotal in the world of Express.js, offering a way to execute code between receiving the request and sending the response. Middleware can perform a myriad of operations like executing code, making changes to the request and response objects, ending the request-response cycle, and calling the next middleware in the stack.
For handling complex route conditions, middleware can be a game changer. Let's say you want to authenticate requests:
const express = require('express');
const app = express();
// Middleware for authentication
function authenticate(req, res, next) {
// Authentication logic here
if (authFailed) {
res.status(403).send('Authentication Required');
} else next();
}
app.use('/secure-path', authenticate, (req, res) => {
res.send('Welcome to the secured route!');
});
app.listen(3000);
In this example, any request to /secure-path
will first pass through the authenticate
middleware. If the authentication fails, the request is terminated with a 403 status. Otherwise, it proceeds to the route handler.
Advanced Route Handling: Parameters, Query Strings, and Beyond
Dynamic Routing:
Dynamic routing allows you to capture values specified at a certain segment of the URL as parameters. This feature is immensely useful for creating RESTful interfaces:
app.get('/users/:userId/books/:bookId', (req, res) => {
res.send(req.params);
});
Here, userId
and bookId
are accessible via req.params
, allowing for dynamic responses based on URL parameters.
Query Strings:
Query strings offer another level of flexibility for your routes, enabling you to capture data sent in the URL's query string:
app.get('/search', (req, res) => {
const { query } = req.query;
// Use the query for searching logic
res.send(`Results for ${query}`);
});
Wrapping Up
Complex route handling in Express.js, while initially daunting, can be effectively managed by leveraging the framework's built-in features such as express.Router
and middleware. Organizing your routes and employing dynamic routing and query strings enable scalable and maintainable codebase growth.
As you continue to explore the depths of Express.js, remember that the framework is designed to be robust yet flexible, allowing you to sculpt it to fit your application's needs. For more advanced patterns and practices, the Express.js documentation and resources like Mozilla's Express/Node introduction can provide invaluable guidance.
In the grand scheme of web development, understanding and mastering route handling in Express.js not only enhances your backend capabilities but also sharpens your overall development acumen. Embrace the journey, and may your routes always lead you to successful applications.