Solving Kotlin Compatibility Issues in Android Development
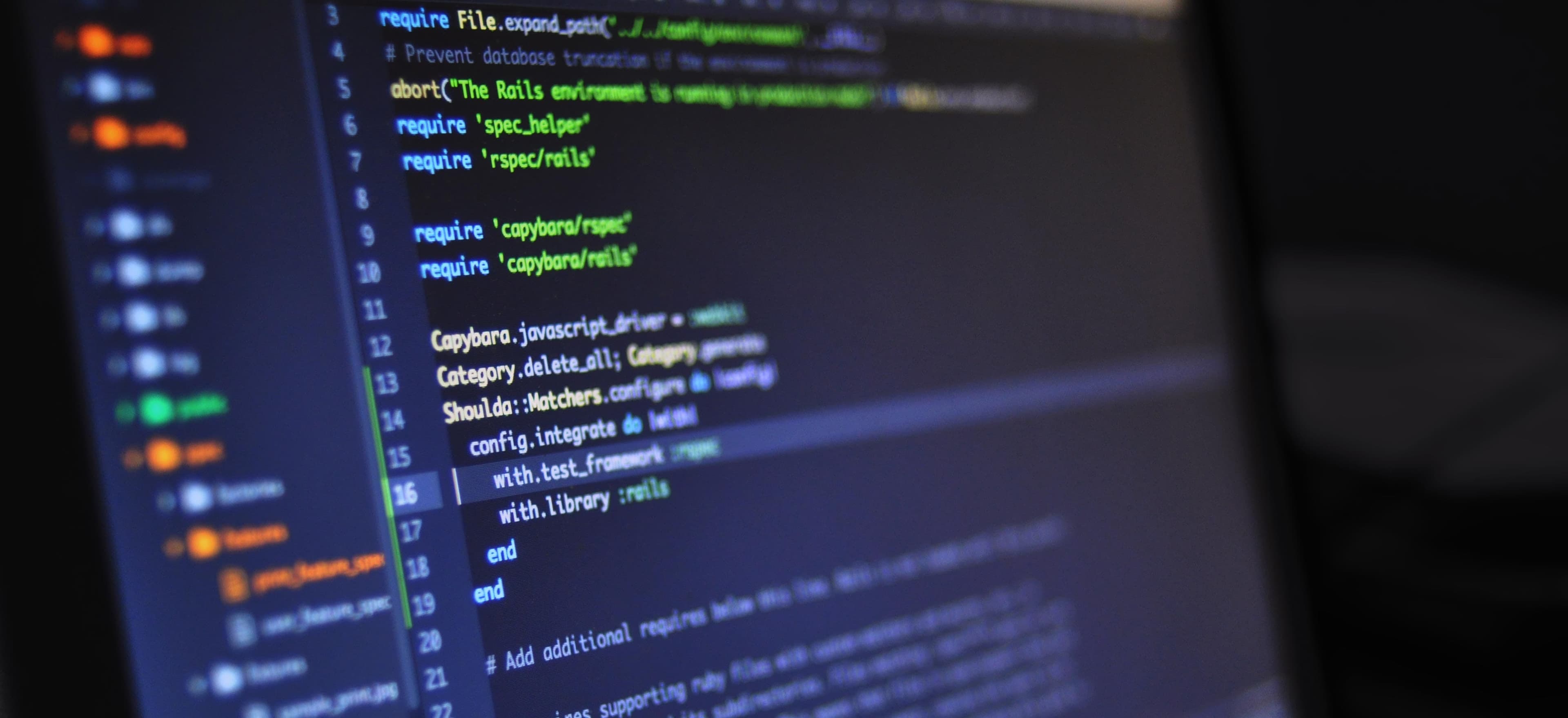
- Published on
Solving Kotlin Compatibility Issues in Android Development
When developing an Android application, choosing Kotlin as the programming language offers numerous advantages, such as improved code conciseness, null safety, and interoperability with Java. However, it's not uncommon for developers to encounter compatibility issues when using Kotlin in their Android projects. In this article, we'll explore some common compatibility issues and demonstrate how to solve them effectively.
1. Kotlin Version Compatibility
Before diving into the code, it's essential to ensure that the Kotlin version used in the project is compatible with the versions of libraries and frameworks being utilized. In Android Studio, this compatibility is typically reflected in the build.gradle
file.
Example:
buildscript {
ext.kotlin_version = '1.5.20'
// Other dependencies...
dependencies {
classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version"
// Other dependencies...
}
}
It's crucial to check the Kotlin version and ensure that it aligns with the versions specified by the libraries and frameworks in the project. Using incompatible versions can lead to unexpected errors and behavior.
2. Interoperability with Java
Kotlin is designed to be fully interoperable with Java, allowing both languages to coexist within the same project. However, there are instances where interoperability issues may arise, especially when working with Java libraries that make use of certain language features not fully supported in Kotlin.
One common issue involves the declaration of checked exceptions in Java, which Kotlin handles differently. When using a Java library that throws checked exceptions, Kotlin code may encounter compilation errors.
To resolve this, Kotlin's @Throws
annotation can be used to specify that a Kotlin function may throw a specific exception, thus addressing the interoperability issue.
Example:
@Throws(IOException::class)
fun readFile() {
// Code that may throw IOException
}
By using @Throws
, it becomes explicit that the function readFile
may throw the IOException
declared in the Java library, ensuring seamless interoperability.
3. Null Safety
Kotlin's emphasis on null safety provides a significant advantage over Java. However, when dealing with Java code that may return null, nullability issues can surface.
Consider a scenario where a Kotlin function calls a Java method that returns a nullable type. This can lead to potential null pointer exceptions if not handled properly.
To address this, Kotlin offers the safe call operator (?.
) and the Elvis operator (?:
) to handle nullable types effectively.
Example:
val result: Int? = javaObject?.nullableMethod() ?: 0
In this example, if javaObject
is not null, nullableMethod()
will be called. If the result is not null, result
will be assigned the returned value. Otherwise, it will be assigned the default value of 0.
4. Kotlin Android Extensions Deprecation
Kotlin Android Extensions, once a popular feature for Android development, has been deprecated in favor of View Binding. Projects that still rely on Kotlin Android Extensions may encounter compatibility issues, especially when migrating to newer versions of Android Gradle Plugin.
To mitigate this issue, migrating to View Binding is recommended. View Binding generates a binding class for each XML layout file present in the module, providing direct references to all views with null safety and no performance overhead.
Migrating to View Binding involves enabling it in the module-level build.gradle file and updating the code to use the generated binding classes.
Example:
android {
viewBinding {
enabled = true
}
}
After enabling View Binding, the generated binding classes can be used to access views directly, ensuring type safety and compatibility with the latest Android Gradle Plugin.
5. Using Kotlin Coroutines with Java
Kotlin Coroutines, a powerful concurrency design pattern, are often favored for asynchronous programming in Kotlin. When working in a codebase that includes both Kotlin and Java, integrating Kotlin Coroutines with existing Java code may present compatibility challenges.
To incorporate Kotlin Coroutines in Java-friendly ways, the @ExperimentalCoroutinesApi
annotation can be used to mark the experimental coroutine-related API elements accessible from Java as @JvmOverloads
or @JvmName
.
Example:
@ExperimentalCoroutinesApi
fun fetchData(callback: Continuation<String>) {
// Coroutines logic
}
By annotating the coroutine-related API elements with @ExperimentalCoroutinesApi
, it becomes possible to use Kotlin Coroutines seamlessly in mixed-language codebases.
In conclusion, when using Kotlin in Android development, compatibility issues may arise, but they can be effectively addressed through version alignment, interoperability techniques, null safety handling, migration to modern features, and strategic use of language-specific features. By resolving these compatibility issues, developers can harness the full potential of Kotlin while maintaining seamless integration with existing Java codebases.
Checkout our other articles