Solving Slow Test Speeds with Quarkus & Testcontainers
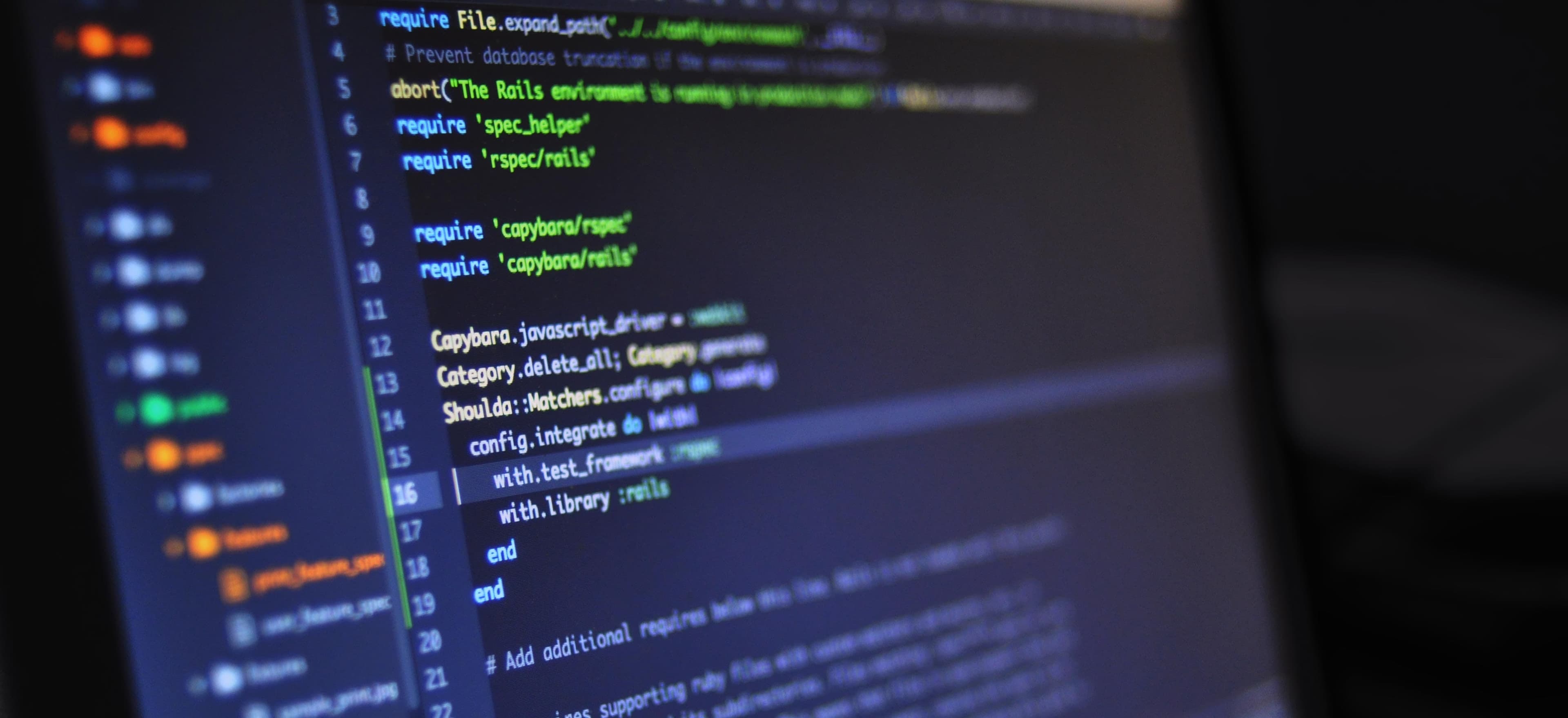
- Published on
Improving Test Speed with Quarkus & Testcontainers
Slow test speeds can be a major hindrance to the development process. Waiting for tests to complete can significantly decrease productivity and impede iterative development. Fortunately, combining Quarkus, a modern Java framework, with Testcontainers, a popular Java testing library, can effectively address this issue. In this blog post, we will explore how to leverage Quarkus and Testcontainers to enhance test speed and efficiency for Java applications.
Understanding the Challenge
Before delving into the solution, it is important to understand the factors contributing to slow test speeds. In many cases, long test execution times result from the overhead of starting and stopping external dependencies such as databases, message brokers, and other services. These dependencies are crucial for testing application behavior, but they can significantly slow down the testing process when started and stopped for each test case.
Introducing Quarkus
Quarkus is a Kubernetes-native Java stack tailored for GraalVM and HotSpot, crafted from the best-of-breed Java libraries and standards. Its ability to produce small and fast bootable JARs makes it an ideal candidate for addressing challenges related to slow test speeds. Quarkus accomplishes this through its blazingly fast startup and low memory consumption, enabling quick test execution without compromising the application's essential functionality.
Leveraging Testcontainers
Testcontainers is a Java library that provides lightweight, throwaway instances of common databases, Selenium web browsers, or anything else that can run in a Docker container for use in JUnit tests. By incorporating Testcontainers into the testing process, developers can seamlessly spin up these dependencies as needed, effectively minimizing the time overhead typically associated with starting and stopping external services for each test.
Integrating Quarkus and Testcontainers
To demonstrate the synergy between Quarkus and Testcontainers, let's consider a scenario where a Quarkus application interacts with a PostgreSQL database. We will build a simple test scenario to showcase how the combination of Quarkus and Testcontainers can significantly enhance test speed.
Setting up the Quarkus Application
First, we need to set up a Quarkus application that interacts with a PostgreSQL database. We can achieve this by defining the necessary dependencies and configurations within the pom.xml
file.
<!-- pom.xml -->
<dependencies>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-agroal</artifactId>
</dependency>
<dependency>
<groupId>io.quarkus</groupId>
<artifactId>quarkus-jdbc-postgresql</artifactId>
</dependency>
<!-- Other dependencies -->
</dependencies>
By including the quarkus-agroal
and quarkus-jdbc-postgresql
dependencies, we enable the Quarkus application to interact with a PostgreSQL database.
Writing the Test Using Testcontainers
With the Quarkus application set up, we can now write a test that utilizes Testcontainers to spin up a PostgreSQL container for the duration of the test. Below is an example of how this can be achieved using Testcontainers' JUnit 5 support.
import org.junit.jupiter.api.Test;
import org.testcontainers.containers.PostgreSQLContainer;
import org.testcontainers.junit.jupiter.Container;
import org.testcontainers.junit.jupiter.Testcontainers;
@Testcontainers
public class QuarkusPostgresIntegrationTest {
@Container
public static PostgreSQLContainer<?> postgresql = new PostgreSQLContainer<>("postgres:latest");
@Test
public void testQuarkusWithPostgres() {
String jdbcUrl = postgresql.getJdbcUrl();
// Use the jdbcUrl to configure the Quarkus application for the test
// Perform assertions and verifications
}
}
In this test class, we utilize Testcontainers' @Container
annotation to declare a PostgreSQL container as a static field, ensuring that it is shared across all test methods in the class. The @Testcontainers
annotation activates the Testcontainers extension for JUnit 5, enabling seamless integration with the testing framework.
By using Testcontainers' PostgreSQLContainer, we can dynamically obtain the JDBC URL for the running PostgreSQL container and configure the Quarkus application to interact with it solely for the duration of the test.
Benefits and Considerations
The integration of Quarkus and Testcontainers offers several advantages:
- Faster Test Execution: By leveraging Testcontainers to spin up necessary dependencies only when required, the overall test execution time is significantly reduced, leading to improved development efficiency.
- Isolation and Consistency: Testcontainers ensures that each test runs against a clean, isolated instance of the required dependency, preventing interference and ensuring consistent test outcomes.
However, it is essential to consider the potential overhead of spinning up containers during testing, particularly with a large number of test cases. Careful consideration and profiling should be undertaken to balance the benefits against the overhead introduced by using Testcontainers.
Key Takeaways
Incorporating Quarkus and Testcontainers into the testing workflow presents a powerful solution for addressing slow test speeds in Java applications. The seamless integration of Quarkus with Testcontainers enables rapid and efficient testing of application functionality that relies on external dependencies. By minimizing the overhead of starting and stopping these dependencies for each test, developers can experience a significant improvement in test speed without sacrificing the integrity of the testing process.
In summary, the combination of Quarkus and Testcontainers offers a compelling approach to enhancing test speed and efficiency, ultimately contributing to a more productive and agile development cycle.
By optimizing your tests in Java, you can boost development speed and efficiency. Try out Quarkus and Testcontainers to enjoy the benefits of faster testing today.
Checkout our other articles