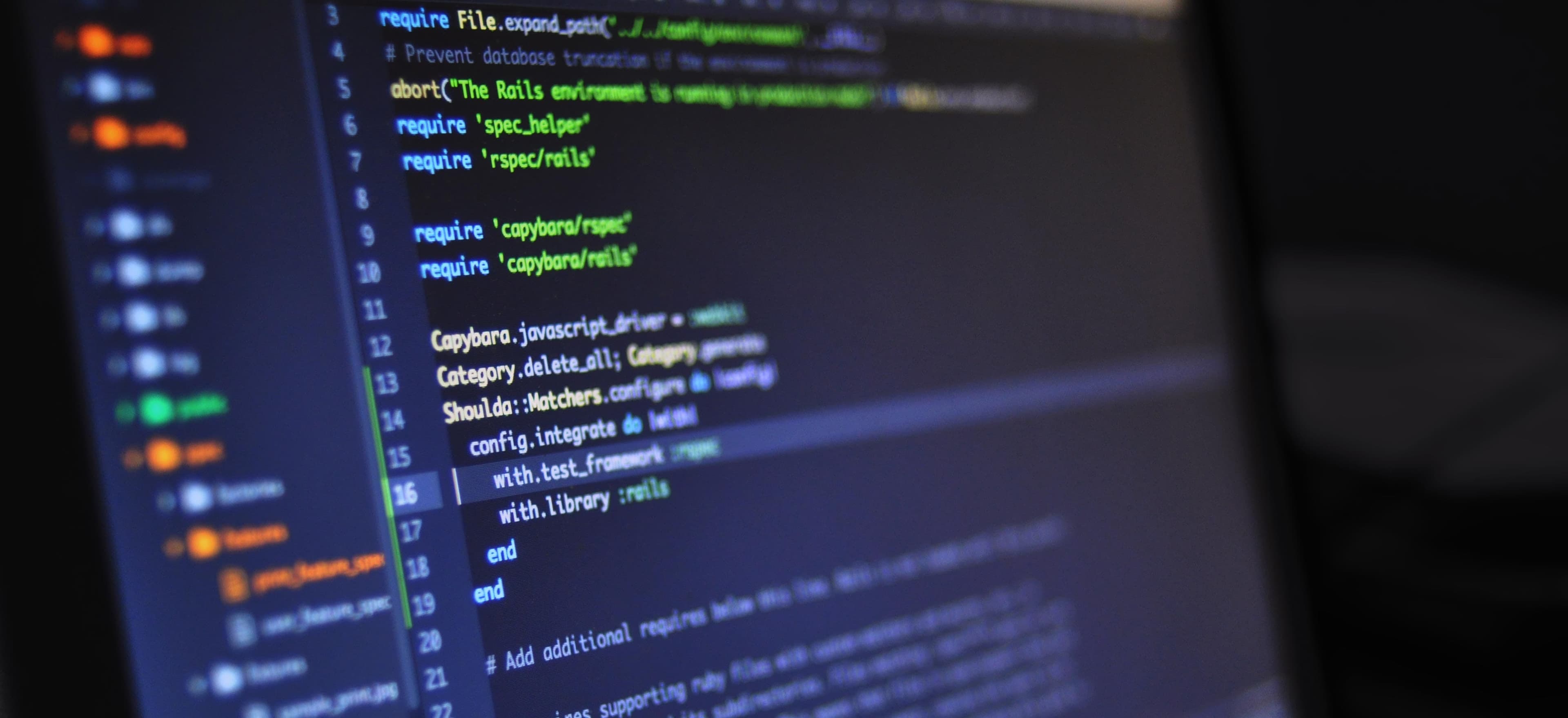
- Published on
Overcoming Common Pitfalls in JOOQ for Type-Safe Queries
When it comes to database access in Java, JOOQ stands out as a powerful library that provides a type-safe way to build SQL queries. However, mastering JOOQ requires overcoming some common pitfalls. In this post, we will explore these pitfalls and learn how to overcome them to harness the full potential of JOOQ for type-safe queries.
Pitfall 1: Manual Mapping of Result Records
When working with JOOQ, one of the common pitfalls you might encounter is the manual mapping of result records to Java objects. This can lead to boilerplate code and increased maintenance overhead.
Solution: Leveraging Record Mapper
JOOQ provides a powerful feature called RecordMapper
which allows for automatic mapping of result records to Java objects. By utilizing this feature, you can eliminate the need for manual mapping and reduce boilerplate code.
// Using RecordMapper to map result records to a custom Pojo
List<MyPojo> pojos = DSL.using(configuration)
.select()
.from(MY_TABLE)
.fetch()
.map(new RecordMapper<Record, MyPojo>() {
@Override
public MyPojo map(Record record) {
return new MyPojo(
record.get(MY_TABLE.MY_FIELD_1),
record.get(MY_TABLE.MY_FIELD_2)
// Additional fields mapping here...
);
}
});
By employing the RecordMapper
, you can streamline the mapping process and make your code more maintainable.
Pitfall 2: Unchecked SQL Queries
Another common pitfall with JOOQ is the potential for unchecked SQL queries, leaving your code vulnerable to SQL injection attacks and schema changes.
Solution: Using Parametrized Queries
To overcome this pitfall, it's crucial to utilize parametrized queries. JOOQ provides the DSL.param()
method which allows you to create parameterized queries, ensuring that user input is properly escaped and preventing SQL injection attacks.
// Using parametrized queries to prevent SQL injection
Result<MyTableRecord> result = DSL.using(configuration)
.selectFrom(MY_TABLE)
.where(MY_TABLE.MY_FIELD.eq(DSL.param("paramValue")))
.fetch();
By using parametrized queries, you can enhance the security of your application and protect it from SQL injection vulnerabilities.
Pitfall 3: Accidental Cartesian Products
Accidental Cartesian products can be a significant issue when working with JOOQ, especially when joining multiple tables without proper conditions, leading to unexpected and potentially detrimental results.
Solution: Adding Join Conditions Explicitly
To mitigate the risk of accidental Cartesian products, it's essential to explicitly define join conditions when working with multiple tables. By doing so, you can prevent unintended combinations and ensure the expected result set.
// Adding explicit join conditions to prevent accidental Cartesian products
DSL.using(configuration)
.select()
.from(TABLE1)
.join(TABLE2).on(TABLE1.ID.eq(TABLE2.TABLE1_ID))
.fetch();
By explicitly specifying the join conditions, you can avoid accidental Cartesian products and maintain the integrity of your query results.
Pitfall 4: Lack of Composition and Reusability
Without proper composition and reusability, JOOQ queries can become repetitive and difficult to maintain, leading to code duplication and increased complexity.
Solution: Utilizing Query Parts and Building Blocks
To address this pitfall, JOOQ offers powerful features such as query parts and building blocks. By leveraging these features, you can compose and reuse query components, reducing redundancy and enhancing maintainability.
// Creating reusable query components using query parts
Condition condition1 = MY_TABLE.MY_FIELD1.eq(value1);
Condition condition2 = MY_TABLE.MY_FIELD2.eq(value2);
DSL.using(configuration)
.select()
.from(MY_TABLE)
.where(condition1.and(condition2))
.fetch();
By utilizing query parts and building blocks, you can encapsulate query logic into reusable components, promoting maintainability and reducing code duplication.
Pitfall 5: Ignoring Generated SQL
One of the pitfalls to avoid when working with JOOQ is ignoring the generated SQL. Failing to understand the underlying SQL statements can lead to inefficient queries and missed optimization opportunities.
Solution: Analyzing Generated SQL and Execution Plans
To overcome this pitfall, it's essential to analyze the generated SQL and understand the execution plans. By doing so, you can identify opportunities for query optimization and ensure efficient database access.
// Logging the generated SQL for analysis
String sql = DSL.using(configuration)
.select()
.from(MY_TABLE)
.where(MY_TABLE.MY_FIELD.eq(value))
.getSQL();
logger.debug("Generated SQL: {}", sql);
By logging and analyzing the generated SQL, you can gain insights into query performance and optimize your database interactions.
Bringing It All Together
As we've explored, JOOQ offers a powerful way to create type-safe queries in Java. By understanding and overcoming these common pitfalls, you can harness the full potential of JOOQ and leverage its features to build robust and maintainable database interactions in your Java applications. With the right approach and best practices, JOOQ can streamline your database access and empower you to write efficient and secure queries.
Incorporating these solutions and best practices will not only enhance your proficiency with JOOQ but also contribute to the overall performance and stability of your Java applications. So, equip yourself with these insights and elevate your JOOQ query-building skills to the next level!
Checkout our other articles