Boosting Java 8 Lambdas with Apache Commons Functor
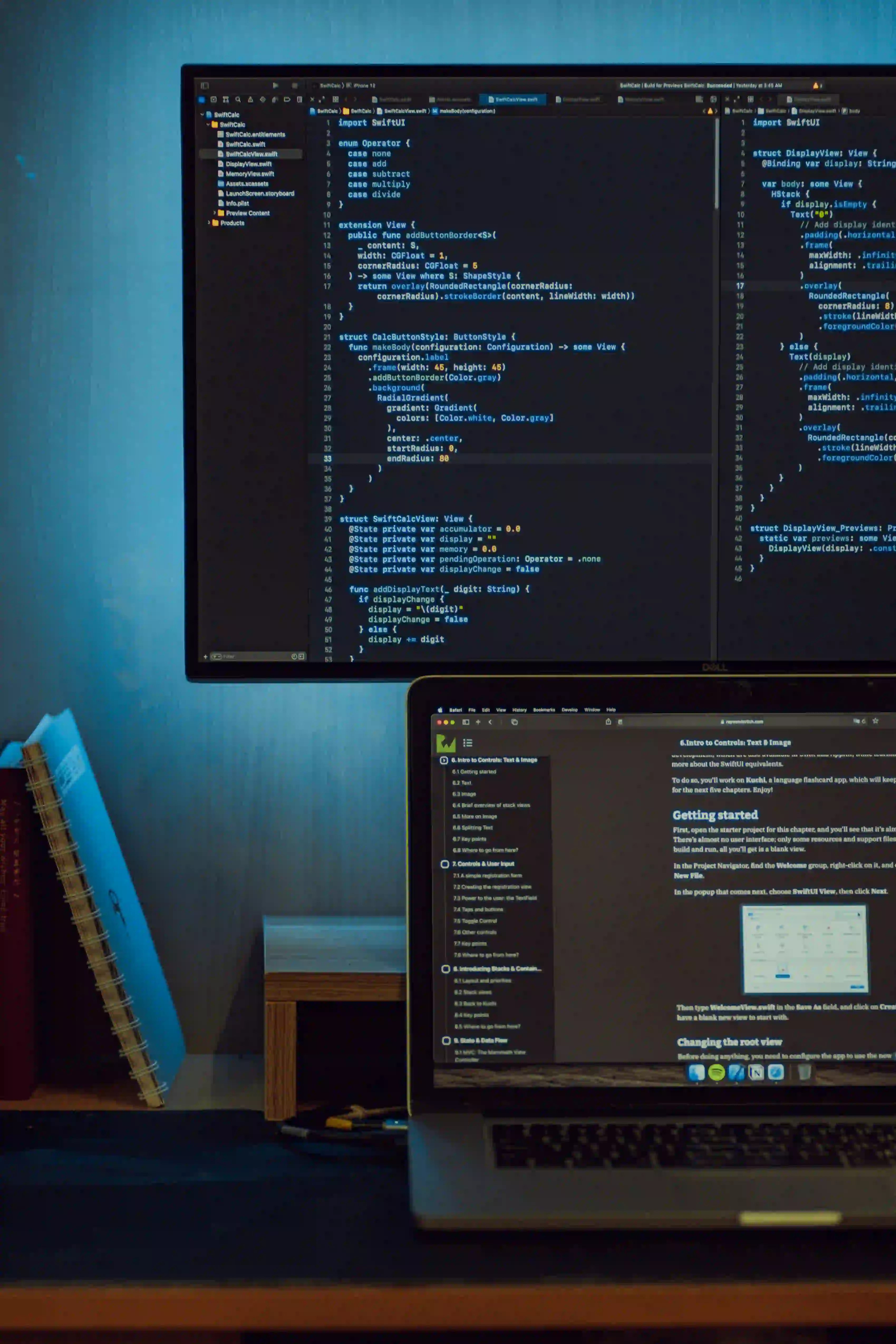
Boosting Java 8 Lambdas with Apache Commons Functor
Java 8 introduced the concept of lambdas, providing a concise way to express behavior using a functional-style syntax. However, the capabilities of lambdas in Java are sometimes limited, especially when dealing with complex use cases. This is where Apache Commons Functor comes into play. In this article, we will explore how Apache Commons Functor can complement Java 8 lambdas to boost their power and flexibility.
Understanding Java 8 Lambdas
Java 8 lambdas are essentially anonymous functions. They allow you to pass behavior as a method argument, leading to more concise and expressive code, especially when working with collections and the Stream API.
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println("Hello, " + name));
In the example above, the lambda name -> System.out.println("Hello, " + name)
is passed to the forEach
method, allowing concise iteration over the names
list.
Limitations of Java 8 Lambdas
While Java 8 lambdas are powerful, they have some limitations. For example, lambdas can only capture final or effectively final variables from the enclosing scope. This can be restrictive when dealing with stateful computations. Additionally, lambdas are limited in terms of composition and reuse.
Introducing Apache Commons Functor
Apache Commons Functor is a library that provides a rich set of abstractions for defining and manipulating functions and predicates in Java. It offers a wide range of functional interfaces and utilities, allowing for more expressive and composable functional programming in Java.
Complementing Java 8 Lambdas with Apache Commons Functor
Let's take a look at some examples to understand how Apache Commons Functor can complement and enhance the capabilities of Java 8 lambdas.
Function Composition
In Java 8, composing functions can be cumbersome, often resulting in verbose and less readable code. Apache Commons Functor provides a Function
interface with built-in support for function composition, making it easy to combine multiple functions into a single function.
import org.apache.commons.functor.Function;
Function<Integer, Integer> increment = input -> input + 1;
Function<Integer, Integer> doubleIt = input -> input * 2;
Function<Integer, Integer> incrementAndDouble = increment.andThen(doubleIt);
System.out.println(incrementAndDouble.evaluate(3)); // Output: 8
In this example, we create two simple functions increment
and doubleIt
, and then use andThen
to compose them into a new function incrementAndDouble
.
Stateful Computations
Java 8 lambdas are limited in their ability to capture and modify mutable state. Apache Commons Functor provides Procedure
and ProcedureFunction
interfaces that allow for the encapsulation of stateful computations.
import org.apache.commons.functor.Procedure;
import org.apache.commons.functor.ProcedureFunction;
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
AtomicInteger sum = new AtomicInteger(0);
Procedure<Integer> addToSum = input -> sum.addAndGet(input);
numbers.forEach(addToSum);
System.out.println(sum.get()); // Output: 15
In this example, we use a stateful computation to calculate the sum of the numbers in the list, leveraging the Procedure
interface to encapsulate the state modification logic.
Predicate Composition
Similar to function composition, Apache Commons Functor provides a Predicate
interface with support for predicate composition, making it easier to combine multiple predicates into a single predicate.
import org.apache.commons.functor.Predicate;
Predicate<Integer> isEven = input -> input % 2 == 0;
Predicate<Integer> isGreaterThanTen = input -> input > 10;
Predicate<Integer> isEvenAndGreaterThanTen = isEven.and(isGreaterThanTen);
System.out.println(isEvenAndGreaterThanTen.test(12)); // Output: true
In this example, we compose two predicates isEven
and isGreaterThanTen
into a new predicate isEvenAndGreaterThanTen
using the and
method.
My Closing Thoughts on the Matter
Java 8 lambdas have significantly improved the way we write code in Java, particularly when it comes to functional-style programming. However, Apache Commons Functor complements Java 8 lambdas by providing additional abstractions and utilities, addressing some of the limitations of lambdas and enhancing their power and flexibility.
In this article, we've only scratched the surface of what Apache Commons Functor has to offer. If you're interested in exploring further, the Apache Commons Functor documentation provides comprehensive information on its features and capabilities.
By leveraging Apache Commons Functor alongside Java 8 lambdas, you can take your functional programming in Java to the next level, enabling more expressive, composable, and flexible code.
Start incorporating Apache Commons Functor in your Java projects today and unlock the full potential of functional programming in Java!