Unlocking PDF Secrets: Extract Info Without Hassle
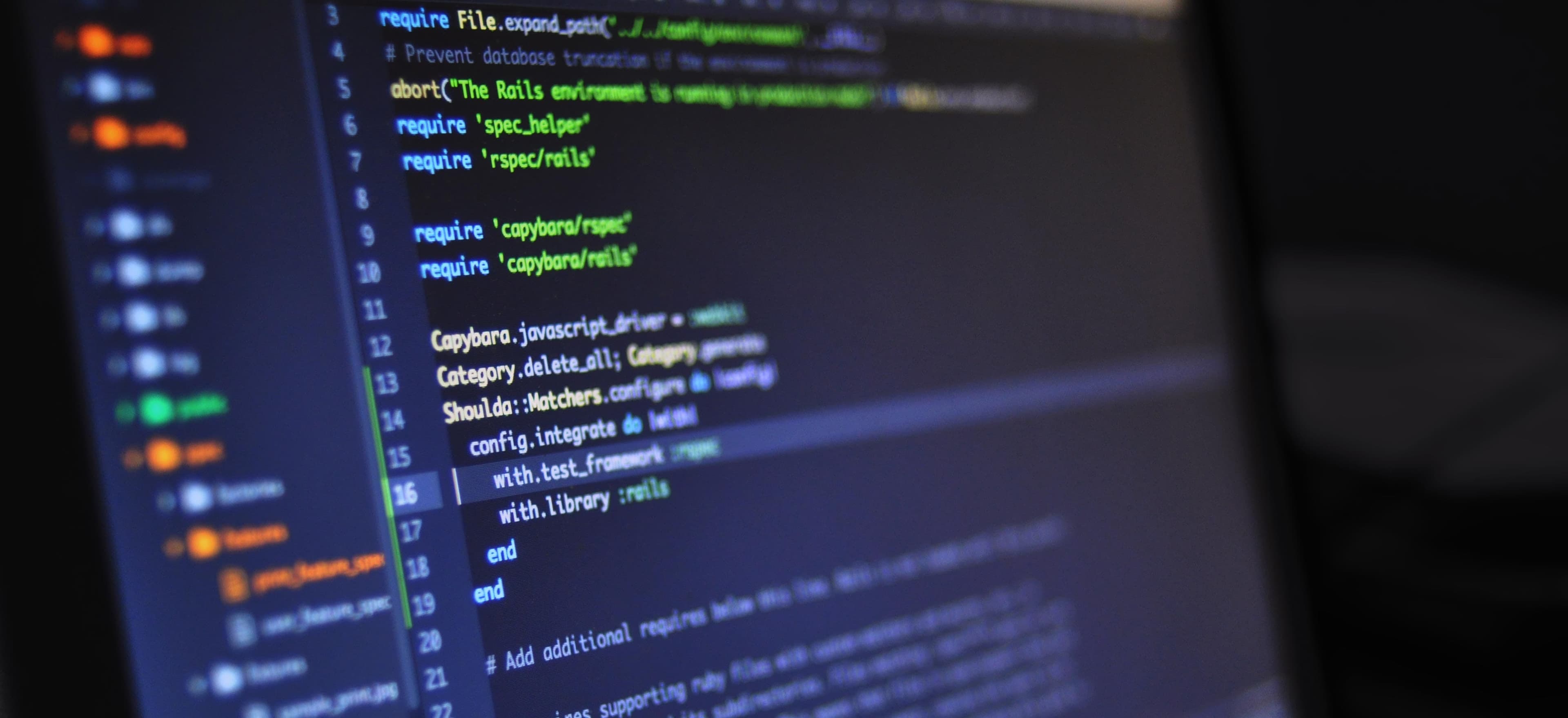
- Published on
Unlocking PDF Secrets: Extract Info Without Hassle
PDF (Portable Document Format) files are a widely used format for exchanging and sharing documents. Thanks to their fixed-layout nature, they are ideal for representing documents in a manner independent of the application software, hardware, and operating systems. Extracting information from PDF files programmatically is often a common requirement for various applications. In this blog post, we will explore how Java can be used to extract useful information from PDF files.
Why Extracting Information from PDFs is Useful
Many organizations and individuals deal with numerous PDF documents on a daily basis. Extracting information from these documents programmatically, such as text, images, or metadata, can be tremendously useful. It enables the automation of tasks like data analysis, content indexing, and metadata extraction. This process can save time and effort by allowing immediate access to the information within the PDFs.
Using Apache PDFBox for PDF Extraction
Apache PDFBox is an open-source Java tool for working with PDF documents. It provides a wide range of capabilities for working with PDFs, including extracting text, images, and metadata. One of the popular use cases of PDFBox is extracting text content from PDFs.
Extracting Text from PDFs using PDFBox
The following code snippet demonstrates how to extract text from a PDF using Apache PDFBox:
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.text.PDFTextStripper;
import java.io.File;
import java.io.IOException;
public class PDFTextExtractor {
public static void main(String[] args) {
try (PDDocument document = PDDocument.load(new File("sample.pdf"))) {
PDFTextStripper textStripper = new PDFTextStripper();
String text = textStripper.getText(document);
System.out.println(text);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the above code, we use the PDDocument
class to load the PDF file, and the PDFTextStripper
class to extract the text content from the document. This extracted text can then be used for further processing or analysis.
Extracting Images from PDFs using PDFBox
In addition to text extraction, PDFBox can also be used to extract images from PDF documents. This can be useful for scenarios where images need to be processed separately from the text content.
Here's an example of extracting images from a PDF using PDFBox:
import org.apache.pdfbox.pdmodel.PDDocument;
import org.apache.pdfbox.pdmodel.PDPage;
import org.apache.pdfbox.pdmodel.PDResources;
import org.apache.pdfbox.pdmodel.graphics.image.PDImageXObject;
import java.io.File;
import java.io.IOException;
public class PDFImageExtractor {
public static void main(String[] args) {
try (PDDocument document = PDDocument.load(new File("sample.pdf"))) {
for (PDPage page : document.getPages()) {
PDResources resources = page.getResources();
for (COSName name : resources.getXObjectNames()) {
if (resources.isImageXObject(name)) {
PDImageXObject image = (PDImageXObject) resources.getXObject(name);
image.write2file("image" + name.getName() + ".png");
}
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
In the code above, we iterate through each page of the PDF document and extract any images present on the page. This allows for further processing or analysis of the extracted images.
Leveraging PDFBox for Metadata Extraction
In addition to text and images, PDFBox can also be used to extract metadata from PDF documents. Metadata, such as author, title, subject, and keywords, can provide valuable information about the document.
Let's take a look at how to extract metadata from a PDF using PDFBox:
import org.apache.pdfbox.pdmodel.PDDocument;
import java.io.File;
import java.io.IOException;
public class PDFMetadataExtractor {
public static void main(String[] args) {
try (PDDocument document = PDDocument.load(new File("sample.pdf"))) {
PDDocumentInformation info = document.getDocumentInformation();
System.out.println("Title: " + info.getTitle());
System.out.println("Author: " + info.getAuthor());
System.out.println("Subject: " + info.getSubject());
System.out.println("Keywords: " + info.getKeywords());
} catch (IOException e) {
e.printStackTrace();
}
}
}
The code above utilizes the PDDocument
class to load the PDF file and then retrieves the document's metadata using the documentInformation
object. This extracted metadata can be used for various purposes, such as categorization and organization of documents.
Final Thoughts
In this blog post, we have explored how Java, with the help of Apache PDFBox, can be used to extract text, images, and metadata from PDF documents. The ability to programmatically extract information from PDFs can streamline various processes and enable more efficient handling of document-based content. Whether it's extracting text content for analysis, processing images separately, or retrieving metadata for categorization, PDFBox provides a versatile solution for working with PDF documents in Java.
By leveraging the capabilities of PDFBox, developers can unlock the power of PDFs and seamlessly integrate PDF content into their Java applications.
For further information and advanced usage of PDFBox, you can explore the Apache PDFBox Documentation.