Master Core Java: Avoid These Common Pitfalls!
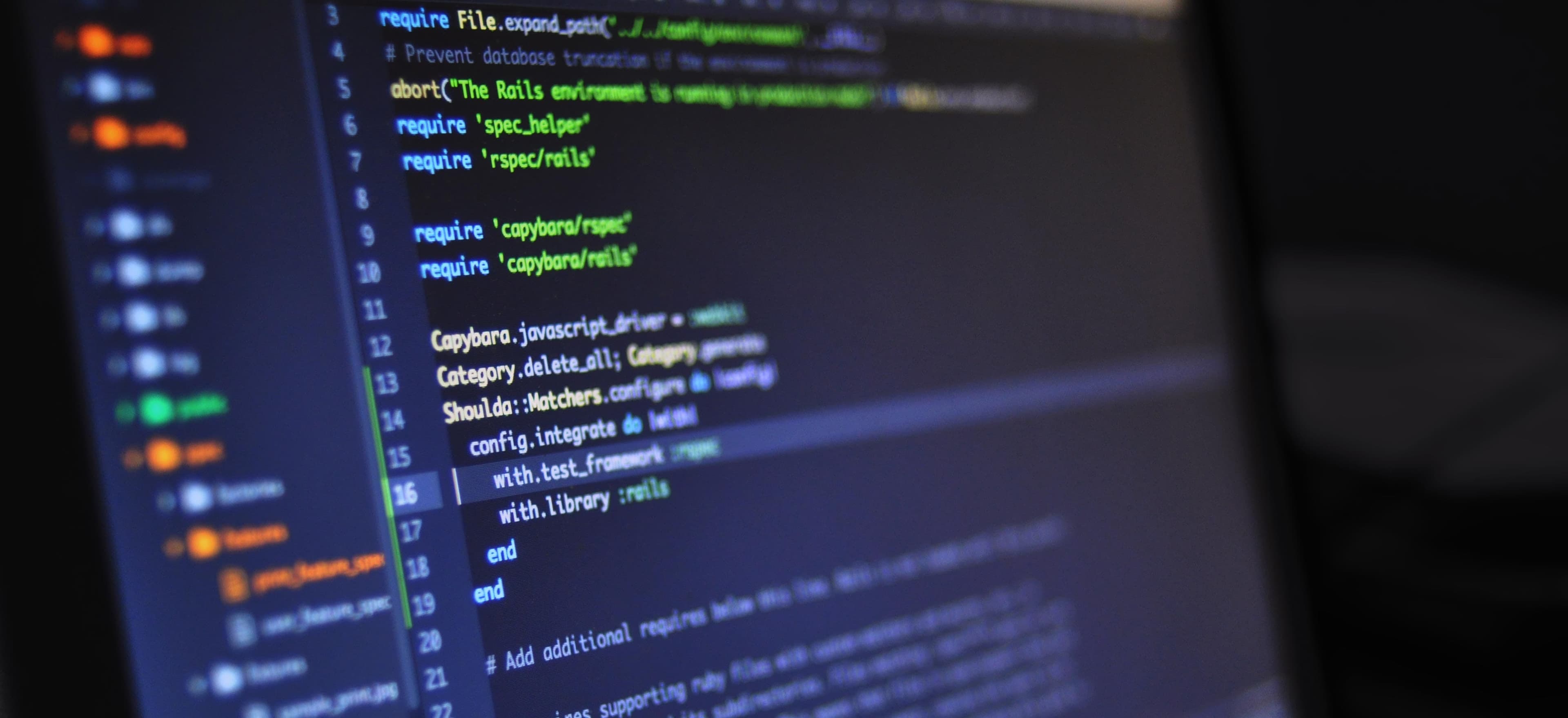
- Published on
Master Core Java: Avoid These Common Pitfalls!
As a Java developer, mastering the core aspects of the language is crucial for writing efficient, maintainable, and error-free code. However, even experienced developers can stumble upon common pitfalls that can lead to bugs, performance issues, or maintenance nightmares. In this post, we will explore some common mistakes and pitfalls in Java programming and learn how to avoid them.
Pitfall 1: Ignoring Exception Handling
One of the most common pitfalls in Java programming is ignoring proper exception handling. Many developers tend to either ignore exceptions altogether or handle them inappropriately, leading to unstable and unreliable applications.
try {
// Some code that may throw an exception
} catch (Exception e) {
// Ignoring the exception or just printing the stack trace
}
Ignoring exceptions or simply printing the stack trace can hide critical issues in the code and make debugging a nightmare. It's essential to handle exceptions properly by either recovering from the error or propagating it up the call stack with meaningful error messages.
Pitfall 2: Misusing String Concatenation
Another common pitfall is the misuse of string concatenation, especially in performance-critical sections of the code. Using the +
operator for concatenating strings in loops or large operations can result in significant performance overhead.
String result = "";
for (int i = 0; i < 1000; i++) {
result += "value" + i;
}
In the above example, each iteration of the loop creates a new string object, leading to unnecessary memory allocations and degraded performance. Instead, using StringBuilder
or StringBuffer
for string manipulation in such scenarios can significantly improve performance.
StringBuilder resultBuilder = new StringBuilder();
for (int i = 0; i < 1000; i++) {
resultBuilder.append("value").append(i);
}
String result = resultBuilder.toString();
Pitfall 3: Neglecting Memory Management
Java's automatic memory management via garbage collection often leads developers to neglect memory management considerations. While the garbage collector takes care of reclaiming unused objects, improper memory management can still lead to memory leaks and degraded performance.
One common pitfall is holding onto references of objects longer than necessary, preventing them from being garbage collected.
List<Object> objects = new ArrayList<>();
while (true) {
objects.add(new Object());
// Do something with the objects
}
In the above scenario, the objects
list keeps growing, consuming more memory, and potentially causing an OutOfMemoryError
. It's essential to release references to objects when they are no longer needed to allow the garbage collector to reclaim memory.
Pitfall 4: Overusing Synchronized Blocks
Synchronization is vital for managing concurrent access to shared resources in a multi-threaded environment. However, overusing synchronized blocks or methods can lead to performance bottlenecks and potential deadlocks.
public synchronized void performSomeOperation() {
// Critical section of code
}
Using synchronized methods or blocks on non-critical sections or entire methods can limit parallelism and degrade performance. It's important to use synchronization judiciously and consider more granular concurrency control mechanisms, such as ReentrantLock
or ConcurrentHashMap
, where appropriate.
Pitfall 5: Neglecting Java API Best Practices
Java provides a rich set of APIs and libraries, and neglecting best practices while using them can lead to code that is difficult to maintain and error-prone.
For example, using raw types instead of parameterized types in collections can lead to runtime type errors and reduce the code's readability and safety.
List list = new ArrayList(); // Using raw type
list.add("Java");
String str = (String) list.get(0); // Casting required
It's crucial to adhere to best practices, such as using parameterized types and leveraging the enhanced for-loop (for-each loop) for iteration, to write safer, more readable code.
List<String> list = new ArrayList<>();
list.add("Java");
String str = list.get(0); // No casting required
Pitfall 6: Tight Coupling and Lack of Abstraction
Tight coupling and lack of abstraction can make the code rigid, difficult to maintain, and resistant to change. When classes and modules are tightly coupled, making changes or introducing new features becomes challenging and error-prone.
public class OrderProcessor {
private PaymentProcessor paymentProcessor;
public OrderProcessor() {
this.paymentProcessor = new PaymentProcessor();
}
public void processOrder(Order order) {
// Process order and payment
paymentProcessor.processPayment(order);
}
}
In the above example, the OrderProcessor
is tightly coupled with the concrete PaymentProcessor
, making it hard to switch or extend different payment processing implementations. Introducing abstraction through interfaces and dependency injection can make the code more flexible and maintainable.
Bringing It All Together
Avoiding these common pitfalls in Java programming can significantly improve code quality, performance, and maintainability. By paying attention to proper exception handling, string concatenation, memory management, synchronization, API best practices, and abstraction, developers can write robust and efficient Java applications.
Mastering core Java requires not only understanding the language features but also being aware of common pitfalls and best practices. By avoiding these pitfalls and following best practices, developers can write more reliable, performant, and maintainable Java code.
Remember, the devil is in the details, and mastering these nuances sets apart a good Java developer from a great one.
Happy coding!
As a reference, you can check out Oracle's Java SE Documentation for detailed information on Java best practices and language features.
Checkout our other articles