Streamlining Data: Mastering JAX-RS 2.0 Server Pipelines
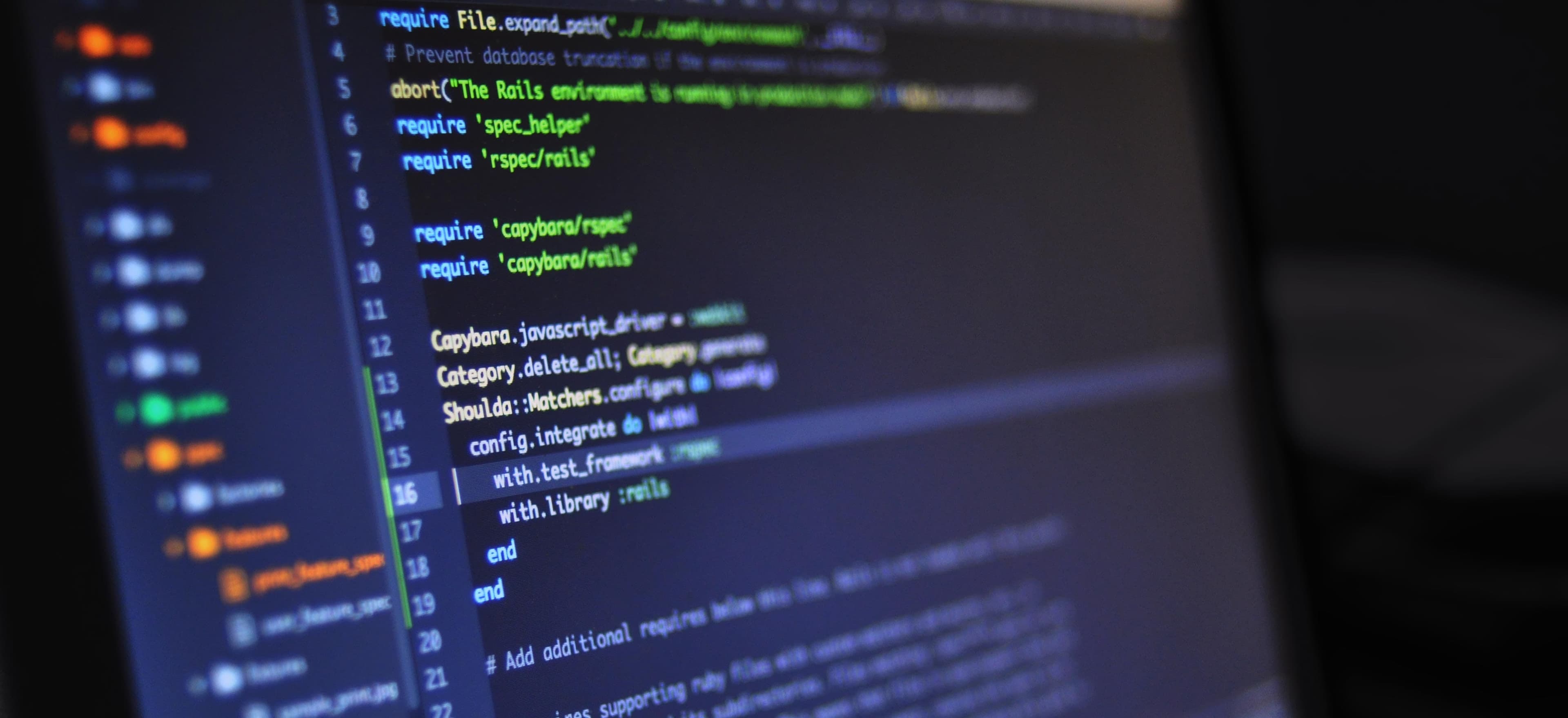
- Published on
Streamlining Data: Mastering JAX-RS 2.0 Server Pipelines
In the world of web applications, efficiently processing and managing data is crucial for delivering high-performing and responsive services. Java developers often turn to JAX-RS (Java API for RESTful Web Services) to build robust and scalable server-side applications. With the release of JAX-RS 2.0, the framework has introduced significant improvements, particularly in the area of server pipelines, enabling developers to streamline data processing and optimize server performance.
In this post, we will delve into the intricacies of JAX-RS 2.0 server pipelines and explore how they can be leveraged to enhance data handling in server-side applications. We will cover practical examples and best practices for effectively utilizing JAX-RS 2.0 features to optimize server pipelines and elevate the performance of your Java web applications.
Understanding JAX-RS 2.0 Server Pipelines
Server pipelines in JAX-RS 2.0 facilitate the processing of incoming HTTP requests and outgoing responses through a series of filters and handlers. This pipeline architecture allows developers to intercept requests and responses, perform pre-processing and post-processing tasks, and manipulate data as it flows through the server.
The Anatomy of JAX-RS Server Pipeline
At the core of the JAX-RS server pipeline are filters and interceptors.
-
Filters are used to modify the request or response headers and entities. They can be applied globally to all resources or to specific resource methods.
-
Interceptors provide a more fine-grained control over the request and response processing. They operate on individual JAX-RS method calls and are used for tasks such as logging, security, and authentication.
Both filters and interceptors are essential components for customizing the behavior of your JAX-RS server and optimizing data processing.
Leveraging JAX-RS Server Pipelines for Data Optimization
Now, let's explore how to leverage JAX-RS 2.0 server pipelines to optimize data handling in your Java web applications.
Example 1: GZIP Compression for Response
One common technique to optimize data transfer between the server and clients is by compressing the response data using GZIP. Let's see how we can use a JAX-RS WriterInterceptor
to apply GZIP compression to the response entity.
@Provider
public class GZIPWriterInterceptor implements WriterInterceptor {
@Override
public void aroundWriteTo(WriterInterceptorContext context) throws IOException, WebApplicationException {
MultivaluedMap<String, Object> headers = context.getHeaders();
headers.add("Content-Encoding", "gzip");
context.setOutputStream(new GZIPOutputStream(context.getOutputStream()));
context.proceed();
}
}
In this example, we implement a WriterInterceptor
named GZIPWriterInterceptor
, which adds the Content-Encoding: gzip
header to the response and wraps the output stream with a GZIPOutputStream
to compress the response entity. By applying this interceptor, we can efficiently compress the response data before transmitting it to the clients, reducing network bandwidth and improving overall performance.
Example 2: Request Logging with Filters
Logging incoming requests is a common practice for monitoring and debugging server interactions. We can use a JAX-RS ContainerRequestFilter
to log incoming requests and gather valuable insights about the data being processed.
@Provider
public class RequestLoggingFilter implements ContainerRequestFilter {
@Override
public void filter(ContainerRequestContext requestContext) throws IOException {
// Log the incoming request data
String method = requestContext.getMethod();
URI uri = requestContext.getUriInfo().getRequestUri();
// Log or process the request data as needed
// ...
}
}
In this example, we define a ContainerRequestFilter
named RequestLoggingFilter
to capture and log the incoming request information. By inserting this filter into the server pipeline, we can gain visibility into the data flowing into our server, enabling us to monitor and analyze the incoming requests effectively.
Best Practices for JAX-RS Server Pipelines
To maximize the effectiveness of JAX-RS server pipelines for data optimization, consider the following best practices:
-
Keep Filters and Interceptors Lightweight: Strive to keep the logic within filters and interceptors minimal and efficient to avoid impacting the overall performance of the server. Heavy computations or long-running tasks should be offloaded to asynchronous processes.
-
Apply Conditional Processing: Utilize filters and interceptors to conditionally process data based on specific criteria, such as request headers, response statuses, or content types. This selective processing can improve resource utilization and enhance data optimization.
-
Combine Multiple Filters and Interceptors: Instead of relying on a single filter or interceptor to handle all aspects of data optimization, consider combining multiple filters and interceptors to compartmentalize and streamline the processing of different tasks, such as compression, authentication, and logging.
-
Testing and Validation: Thoroughly test and validate the behavior of filters and interceptors to ensure they do not introduce unintended side effects or disrupt the normal flow of data within the server pipeline.
A Final Look
In conclusion, JAX-RS 2.0 server pipelines offer a powerful mechanism for optimizing data processing and enhancing the performance of Java web applications. By strategically utilizing filters and interceptors, developers can fine-tune the handling of incoming requests and outgoing responses, leading to efficient data transmission, improved resource utilization, and enhanced server performance.
As you continue to explore the capabilities of JAX-RS 2.0, consider the practical examples and best practices outlined in this post to harness the full potential of server pipelines for data optimization in your Java web applications.
Remember, mastering JAX-RS server pipelines is not just about processing data - it's about streamlining it to deliver exceptional user experiences and robust web services.
For further information on JAX-RS 2.0 and server pipelines, refer to the official JAX-RS documentation.
Checkout our other articles