Streamlining Workflow: Mastering State Machine Implementation
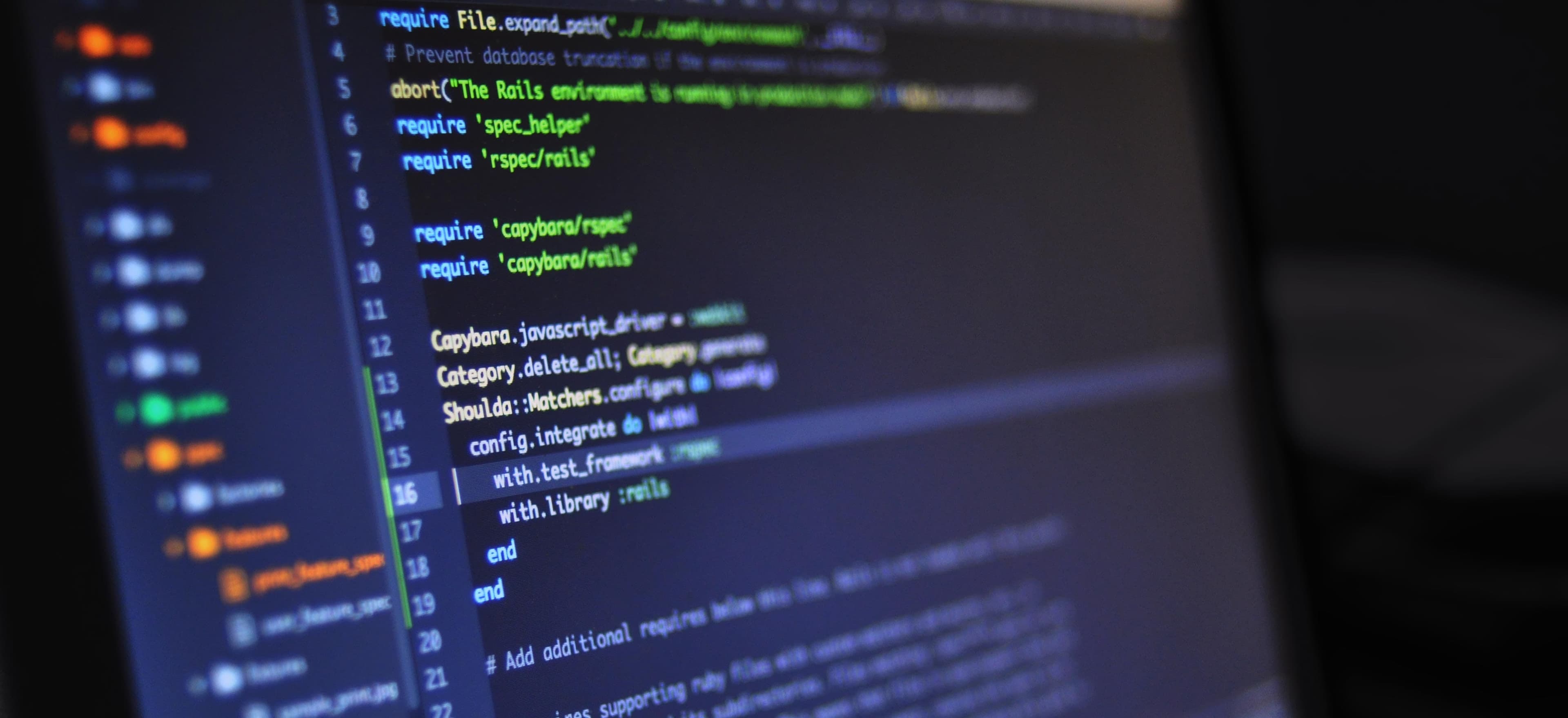
- Published on
Streamlining Workflow: Mastering State Machine Implementation in Java
In the realm of software development, efficiency and clarity are the pillars upon which successful projects are built. Among the various strategies to achieve this, implementing a state machine in Java stands out for its capacity to simplify complex flows and enhance code organization. For developers looking to streamline their workflow and introduce a higher level of professionalism into their projects, mastering state machine implementation can be a game-changer. This blog post delves into the what, why, and how of state machines in Java, complete with code examples and relevant links for deeper understanding.
What Is a State Machine?
At its core, a state machine is a design pattern used to manage complex states and transitions between those states within a system. It models the behavior of an entity by explicitly defining its states, the events that trigger a state change, and the actions that result from a state change. This conceptual simplicity and clarity make state machines incredibly useful for modeling workflows, user interfaces, protocol operations, and more.
Learn more about state machines and their theoretical background here: Introduction to State Machines.
Why Use State Machine in Java?
Java, with its object-oriented features and robust standard library, offers a fertile ground for implementing state machines. By tapping into Java’s capabilities, developers can:
- Simplify Complex Logic: Break down complicated if-else or switch cases that manage state into more manageable, discrete components.
- Improve Code Maintainability: State machines make future modifications easier by localizing state-related changes.
- Enhance Readability: A well-implemented state machine in Java can offer clear insights into the workflow and logic, making it easier for newcomers to grasp the system's workings.
- Reduce Bugs: Explicit state management reduces the chances of unexpected states, a common source of bugs in software development.
Implementing a State Machine in Java
Let’s dive into an example that illustrates how to implement a simple state machine in Java. We’ll design a traffic light system where the light transitions from Green to Yellow, Yellow to Red, and Red back to Green.
Step 1: Define States and Events
First, we define the possible states and events in our system. Using enums is an elegant way to represent these in Java due to their simplicity and readability.
public enum TrafficLightState {
GREEN, YELLOW, RED;
}
public enum TrafficLightEvent {
TIMER_EXPIRED, POWER_OUTAGE, POWER_RESTORED;
}
Step 2: Create the State Machine
We then build the state machine itself. One approach is to create a class that encapsulates the current state and handles events.
public class TrafficLightStateMachine {
private TrafficLightState currentState;
public TrafficLightStateMachine() {
this.currentState = TrafficLightState.RED; // starting state
}
public void handleEvent(TrafficLightEvent event) {
switch(currentState) {
case RED:
if (event == TrafficLightEvent.TIMER_EXPIRED) {
currentState = TrafficLightState.GREEN;
System.out.println("Changed state to GREEN");
}
break;
case YELLOW:
if (event == TrafficLightEvent.TIMER_EXPIRED) {
currentState = TrafficLightState.RED;
System.out.println("Changed state to RED");
}
break;
case GREEN:
if (event == TrafficLightEvent.TIMER_EXPIRED) {
currentState = TrafficLightState.YELLOW;
System.out.println("Changed state to YELLOW");
}
break;
// Include logic for POWER_OUTAGE and POWER_RESTORED events
default:
throw new IllegalStateException("Unexpected state: " + currentState);
}
}
// Additional methods for state management could be added here
}
Commentary:
- Why Enums?: Enums in Java are a robust mechanism for representing fixed sets of constants, like states or events. They're more type-safe than using integers or strings for such purposes, minimizing errors.
- Why Switch on State?: Using the switch statement to manage state transitions keeps the logic clear and localized. It makes understanding and modifying the state transition logic more straightforward.
Step 3: Testing the State Machine
Finally, it's crucial to test our state machine to ensure it behaves as expected. A simple main method demonstrates how to trigger state transitions.
public class Main {
public static void main(String[] args) {
TrafficLightStateMachine trafficLight = new TrafficLightStateMachine();
// Simulate timer expiry events
trafficLight.handleEvent(TrafficLightEvent.TIMER_EXPIRED);
trafficLight.handleEvent(TrafficLightEvent.TIMER_EXPIRED);
trafficLight.handleEvent(TrafficLightEvent.TIMER_EXPIRED);
// This should transition through GREEN -> YELLOW -> RED.
}
}
Expanding Our Horizons
The example above illustrates a state machine's basic structure and functionality. However, real-world applications often require more sophisticated constructs. Libraries like Spring State Machine offer powerful features for state management in enterprise-level applications, further simplifying the development of complex software systems.
To Wrap Things Up
Implementing a state machine in Java can transform an unwieldy web of conditional logic into a clean, manageable structure, increasing both the reliability and comprehensibility of your code. Through the example of a traffic light system, we’ve seen how enums and a simple class can encapsulate state transitions in an understandable format. Encouraged by these principles, you're now better equipped to handle more complex scenarios, improving your Java applications' organization and maintainability.
Remember, mastering state machine implementation doesn't just enhance your current project; it equips you with a versatile tool for your software development toolkit, one that can be adapted to a wide range of programming challenges. So, embrace the clarity and structure that state machines bring to your Java applications – your future self will thank you.
For developers eager to dive deeper into Java and state machines, exploring advanced state machine frameworks like Spring State Machine can open new doors to efficiently managing application states in larger-scale projects. Happy coding!
Checkout our other articles