Solving Java 7 NIO.2 File Filtering Issues Like a Pro
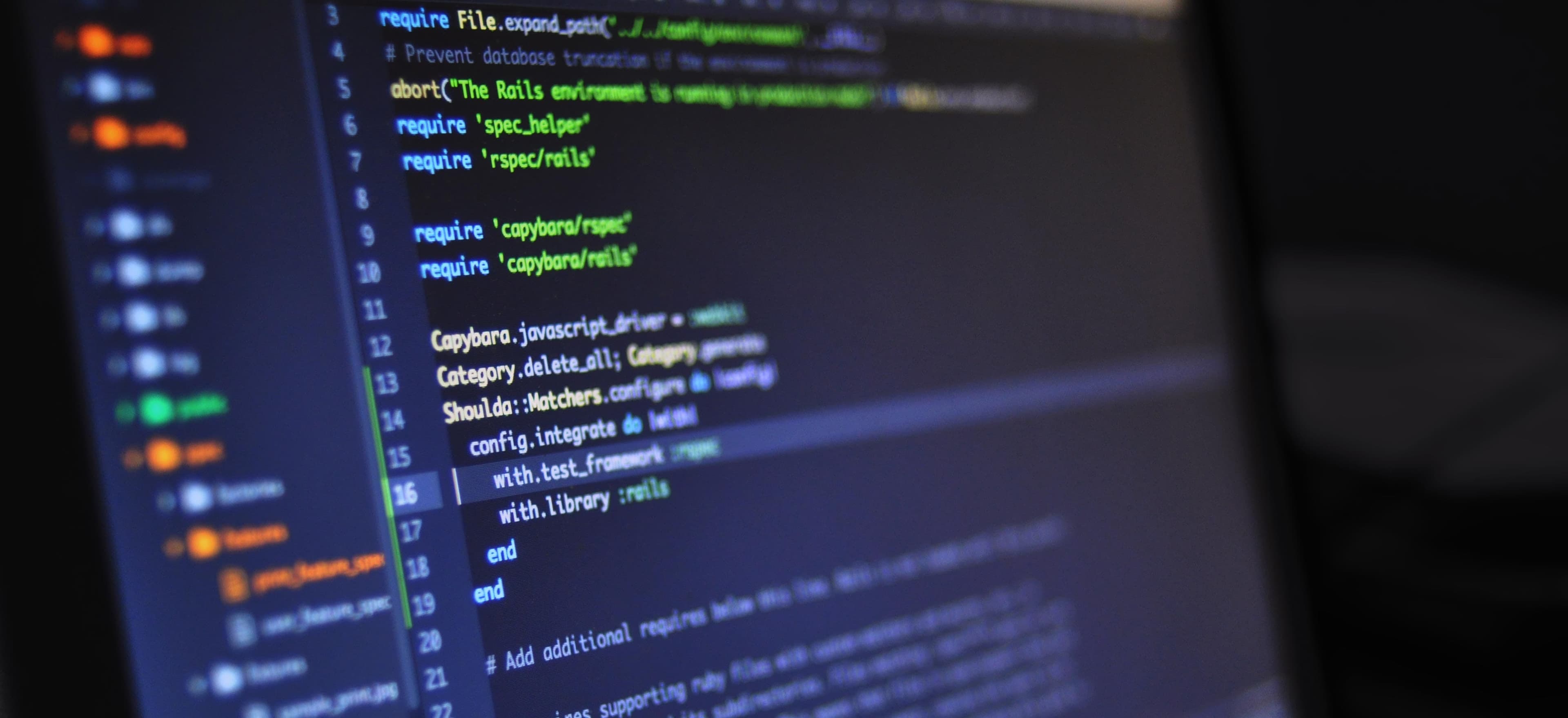
- Published on
Solving Java 7 NIO.2 File Filtering Issues Like a Pro
Java 7 NIO.2 (New I/O) provides a powerful API for file operations. However, when it comes to file filtering, many developers struggle to leverage its potential. In this article, we'll dive into solving Java 7 NIO.2 file filtering issues like a pro. We'll explore common filtering scenarios and demonstrate effective solutions using Java code examples.
Understanding the Problem
File filtering is essential when working with a large number of files to process only specific files based on certain conditions. The java.nio.file
package in Java 7 comes with a comprehensive set of classes and interfaces for file operations, including filtering.
The Files
Class
The java.nio.file.Files
class is a powerhouse for file manipulation. With methods like newDirectoryStream
, walk
, and find
, it provides various ways to traverse a file system and apply filters.
The DirectoryStream
Interface
The java.nio.file.DirectoryStream
interface represents a directory stream, which is a sequence of file and directory entries. It provides an iterator-like API for iterating over the entries in a directory.
Common Filtering Scenarios
Let's consider a few common file filtering scenarios:
1. Filtering by File Extension
Filtering files based on their extensions is a common requirement. For instance, we might want to process only .txt files from a directory containing various file types.
2. Filtering by File Name
Sometimes, we need to filter files based on their names. This could involve selecting files that start with a specific prefix or match a particular pattern.
3. Filtering by File Attributes
Filtering based on file attributes, such as size, creation date, or last modified date, is another common scenario. We might want to process files that meet certain attribute criteria.
Solution: Java 7 NIO.2 File Filtering
Let's now tackle these filtering scenarios using Java 7 NIO.2. We'll provide practical code examples with detailed explanations.
Filtering by File Extension
Path dir = Paths.get("/path/to/directory");
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir, "*.txt")) {
for (Path entry : stream) {
// Process the .txt files
}
} catch (IOException e) {
// Handle exception
}
In this example, the Files.newDirectoryStream
method is used to obtain a directory stream of .txt files. The glob pattern "*.txt" serves as the filter to include only files with the .txt extension.
Filtering by File Name
Path dir = Paths.get("/path/to/directory");
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir, "myfile*")) {
for (Path entry : stream) {
// Process the files with names starting with "myfile"
}
} catch (IOException e) {
// Handle exception
}
Here, the directory stream is filtered to include only files starting with "myfile" using the pattern "myfile*".
Filtering by File Attributes
Path dir = Paths.get("/path/to/directory");
try (DirectoryStream<Path> stream = Files.newDirectoryStream(dir, entry -> Files.size(entry) > 1024)) {
for (Path entry : stream) {
// Process files with size greater than 1024 bytes
}
} catch (IOException e) {
// Handle exception
}
In this case, a lambda expression is used to filter the directory stream based on the file size. Only files with a size greater than 1024 bytes will be processed.
Final Considerations
Java 7 NIO.2 provides robust capabilities for file filtering, empowering developers to efficiently work with files based on specific criteria. By leveraging the Files
class and the DirectoryStream
interface, we can effectively address various filtering scenarios. Understanding these techniques equips developers with the skills to handle diverse file processing requirements with elegance and precision.
By mastering file filtering in Java 7 NIO.2, developers can streamline their file-related operations and foster more efficient, scalable, and maintainable codebases.
In conclusion, by mastering file filtering in Java 7 NIO.2, developers can streamline their file-related operations and foster more efficient, scalable, and maintainable codebases.
For further reading, check out the official Oracle documentation on file I/O in Java.