Boost Your Spring App with gRPC: A How-To Guide
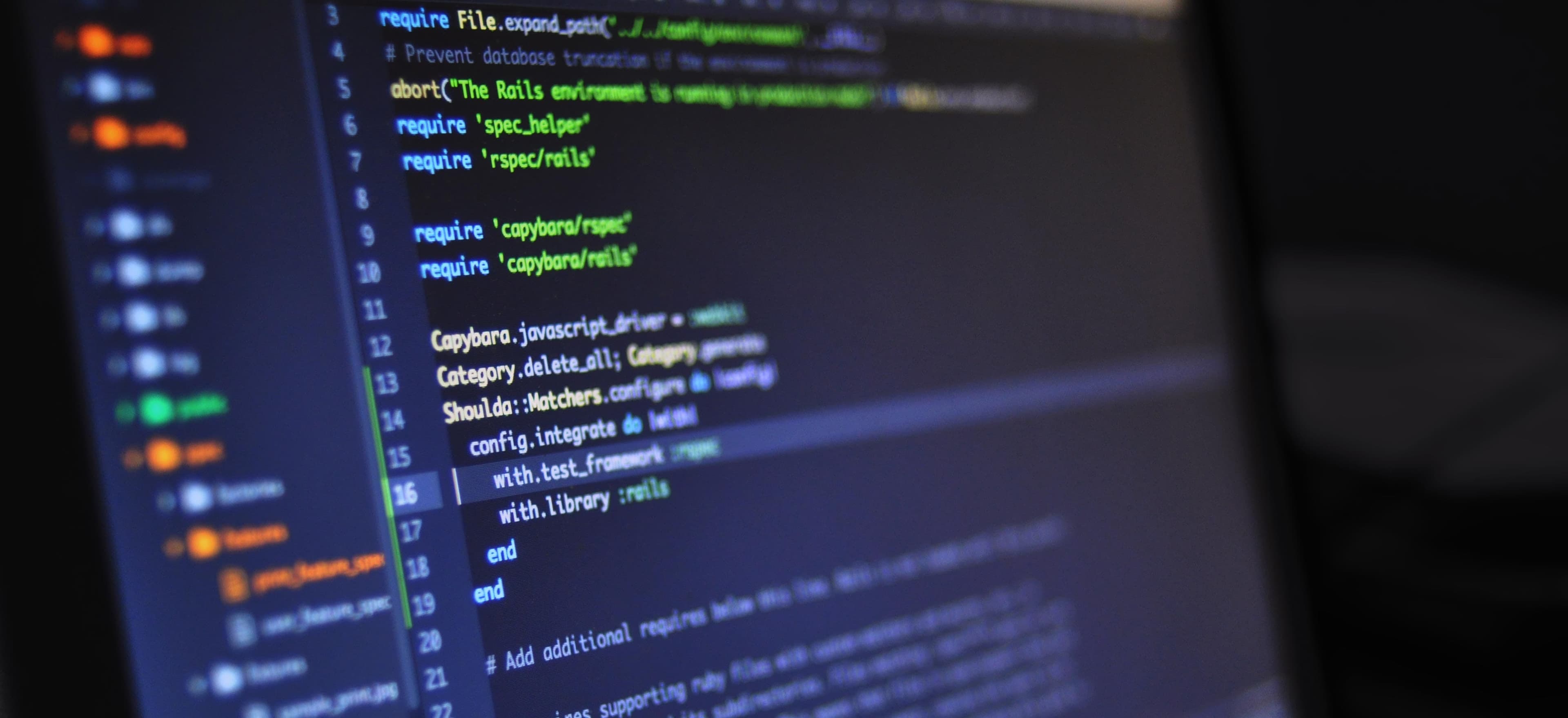
- Published on
Boost Your Spring App with gRPC: A How-To Guide
In the ever-evolving landscape of microservices and distributed systems, optimizing communication between services is key to performance and scalability. This is where gRPC, Google's open-source remote procedure call system, comes into play. Integrating gRPC with your Spring Boot application can dramatically improve inter-service communication efficiency, thanks to its lightweight, high-performance nature. In this post, we'll delve into the fundamentals of gRPC, why it's a game-changer for Spring applications, and provide a step-by-step guide on how to implement gRPC in a Spring Boot app.
Understanding gRPC and Its Advantages
gRPC stands for gRPC Remote Procedure Calls. It is a modern, open-source, high-performance remote procedure call (RPC) framework that can run in any environment. It efficiently connects services in and across data centers with pluggable support for load balancing, tracing, health checking, and authentication. It is also applicable in the last mile of distributed computing to connect devices, mobile apps, and browsers to backend services.
The key advantages of gRPC include:
- Efficiency: gRPC messages are serialized using Protobuf (short for Protocol Buffers), Google's language-neutral, platform-neutral, extensible mechanism for serializing structured data. This is much more efficient than JSON or XML.
- Performance: gRPC employs HTTP/2 for transport, enabling multiplexing and other enhancements over HTTP/1.x. This leads to significant performance improvements.
- Streaming: gRPC supports streaming requests and responses, providing an avenue for building sophisticated, high-performing applications.
- Language Independence: gRPC offers tools to generate client and server code in various programming languages, making it versatile for use in diverse environments.
For more details on gRPC and its capabilities, visit the official gRPC website.
Integrating gRPC in a Spring Boot Application
Now, let's move on to how we can integrate gRPC into a Spring Boot application. We will walk through setting up a simple gRPC server and client within a Spring Boot app.
Pre-requisites
- Java Development Kit (JDK) 8 or later
- Spring Boot
- Maven or Gradle (for dependency management)
- Basic understanding of Spring Boot and Protobuf
Step 1: Setting Up Your Project
First, create a Spring Boot project. You can do this easily using the Spring Initializr. Choose your preferred project meta-data and add the Spring Web
dependency for simplicity.
Step 2: Adding gRPC Dependencies
In your pom.xml
(for Maven users) or build.gradle
(for Gradle users), add the following dependencies to include gRPC and Protobuf in your project.
For Maven, add:
<dependencies>
<dependency>
<groupId>net.devh</groupId>
<artifactId>grpc-server-spring-boot-starter</artifactId>
<version>2.12.0.RELEASE</version>
</dependency>
<dependency>
<groupId>net.devh</groupId>
<artifactId>grpc-client-spring-boot-starter</artifactId>
<version>2.12.0.RELEASE</version>
</dependency>
</dependencies>
For Gradle, add:
dependencies {
implementation 'net.devh:grpc-server-spring-boot-starter:2.12.0.RELEASE'
implementation 'net.devh:grpc-client-spring-boot-starter:2.12.0.RELEASE'
}
Step 3: Defining the gRPC Service
Create a .proto
file under src/main/proto
. This file defines the structure of your gRPC service and messages. For instance, a simple GreetingService
can be defined as follows:
syntax = "proto3";
option java_multiple_files = true;
option java_package = "com.example.demo.grpc";
option java_outer_classname = "GreetingProto";
package greeting;
// The greeting service definition.
service GreetingService {
// Sends a greeting
rpc greet (GreetingRequest) returns (GreetingResponse) {}
}
// The request message containing the user's name.
message GreetingRequest {
string name = 1;
}
// The response message containing the greetings
message GreetingResponse {
string message = 1;
}
Step 4: Generating gRPC Code
To generate Java classes from your .proto
files, you need to add the Protobuf Maven Plugin for Maven users or the equivalent for Gradle. Here’s how you do it with Maven:
<build>
<plugins>
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<configuration>
<protocArtifact>
com.google.protobuf:protoc:3.7.1:exe:${os.detected.classifier}
</protocArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>test-compile</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
When you build your project (mvn clean install
for Maven users), Java classes corresponding to the service definitions in your .proto
files will be automatically generated.
Step 5: Implementing the gRPC Service
Now, implement the GreetingService
in your Spring Boot application. Create a class that extends the generated GreetingServiceGrpc.GreetingServiceImplBase
class and override the greet
method:
package com.example.demo.grpc;
import io.grpc.stub.StreamObserver;
import net.devh.boot.grpc.server.service.GrpcService;
@GrpcService
public class GreetingServiceImpl extends GreetingServiceGrpc.GreetingServiceImplBase {
@Override
public void greet(GreetingRequest req, StreamObserver<GreetingResponse> responseObserver) {
String message = "Hello, " + req.getName();
GreetingResponse response = GreetingResponse.newBuilder().setMessage(message).build();
responseObserver.onNext(response);
responseObserver.onCompleted();
}
}
Step 6: Creating a gRPC Client
In a real-world scenario, you'll often need to consume a gRPC service from a different microservice or application. Let's quickly look at how to create a gRPC client within our Spring Boot app for demonstration purposes:
package com.example.demo.grpc;
import net.devh.boot.grpc.client.inject.GrpcClient;
import org.springframework.stereotype.Service;
@Service
public class GreetingClientService {
@GrpcClient("local-grpc-server")
private GreetingServiceGrpc.GreetingServiceBlockingStub greetingServiceStub;
public String greet(String name) {
GreetingRequest request = GreetingRequest.newBuilder().setName(name).build();
GreetingResponse response = greetingServiceStub.greet(request);
return response.getMessage();
}
}
Step 7: Testing Your gRPC Service
To test your gRPC service, you can write a Spring Boot test or simply run your application and use a tool like BloomRPC to interact with your gRPC server.
To Wrap Things Up
Bringing gRPC into your Spring Boot application offers a blend of efficiency, performance, and cross-language support that is hard to match with traditional REST APIs. Whether you're building microservices that need to talk to each other efficiently or developing a back-end service for a mobile app, gRPC and Protobuf offer a compelling solution.
The steps outlined here provide a solid foundation, but there's much more to explore, including authentication, error handling, and gRPC streaming capabilities. Dive deeper into these topics, and keep experimenting. The high-performance and efficiency gains are worth the effort.
For further reading and an in-depth look at advanced features, the Spring documentation and the gRPC documentation are invaluable resources. Happy coding!