Push vs Pull Strategy: Boosting Agile Project Success
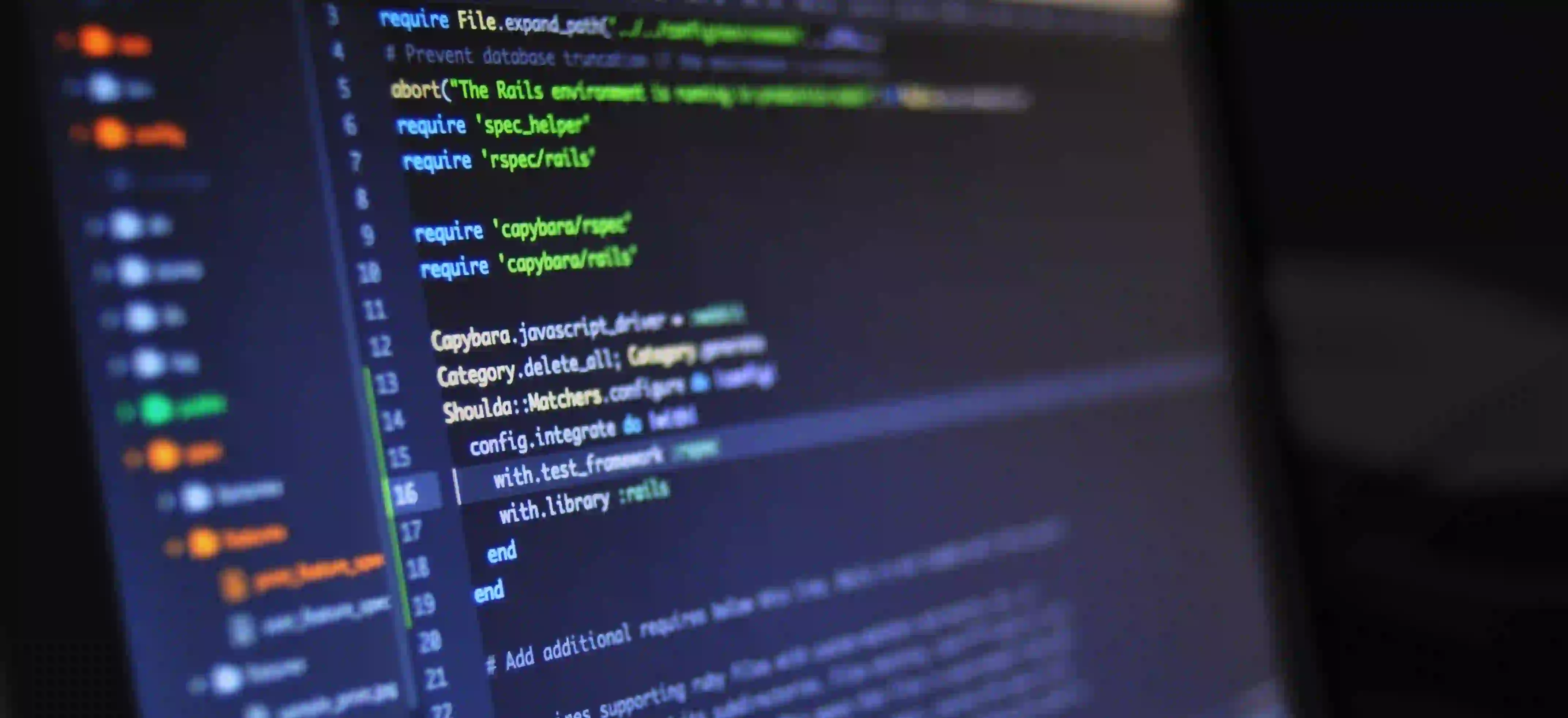
Push vs Pull Strategy: Boosting Agile Project Success
In the realm of Agile project management, the debate between push and pull strategies continues to provoke interesting discussions. Understanding when to push and when to pull is crucial for the success of Agile projects. This blog post aims to delve into the differences between push and pull strategies, how they impact Agile projects, and how to use them effectively to boost project success.
Understanding Push and Pull Strategies
Push Strategy
A push strategy, in the context of Agile project management, refers to the traditional approach of assigning tasks and setting deadlines by the project manager or team lead. This method is based on the assumption that team members require explicit direction and that work needs to be pushed onto them to ensure progress. However, this approach can lead to micromanagement and reduced autonomy among team members, inhibiting creativity and innovation.
Pull Strategy
Conversely, a pull strategy involves allowing team members to pull work as they have the capacity and availability to do so. It empowers team members to take ownership of their responsibilities and decide when and how they will contribute to the project. This strategy aligns well with Agile principles, promoting self-organization, collaboration, and adaptability.
Impact on Agile Projects
Push Strategy Impact
While push strategies may work in certain situations, they often lead to a lack of motivation, decreased productivity, and a rigid project environment. Team members may feel disengaged and constrained by the imposed deadlines and tasks, resulting in a higher likelihood of missed deadlines and lower-quality work. Push strategies can also hinder the adaptation to changing requirements, as they prioritize adherence to an initial plan over responsiveness.
Pull Strategy Impact
In contrast, pull strategies tend to foster a more dynamic and responsive project environment. By allowing team members to pull work based on their capacity and expertise, Agile projects can benefit from increased motivation, higher productivity, and improved collaboration. The empowerment of team members to make decisions regarding their work fosters a sense of ownership and accountability, leading to better results and adaptability to evolving project requirements.
Choosing the Right Strategy for Agile Projects
Context and Project Dynamics
The decision to adopt a push or pull strategy should be informed by the specific context and dynamics of the Agile project. Factors such as the experience and expertise of team members, the project timeline, the level of uncertainty in requirements, and the organizational culture all play a significant role in determining the most suitable strategy.
Hybrid Approach
In practice, a hybrid approach that combines elements of both push and pull strategies can often yield the best results for Agile projects. For instance, while certain high-priority tasks or time-sensitive deliverables may require a push approach to ensure timely completion, allowing flexibility and autonomy for less critical tasks can be managed through a pull strategy. Striking the right balance is essential for optimizing productivity and engagement within the team.
Implementing Pull Strategy in Agile Projects: A Java Example
Now, let's explore a code example that demonstrates the implementation of a pull strategy within an Agile project, using Java as the programming language. In this example, we'll consider a simplified task management system where team members can pull tasks from a backlog based on their availability and expertise.
import java.util.ArrayList;
import java.util.List;
class Task {
private String description;
public Task(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
}
class TaskBoard {
private List<Task> backlog;
public TaskBoard() {
this.backlog = new ArrayList<>();
}
public void addTaskToBacklog(Task task) {
backlog.add(task);
}
public Task pullTask() {
if (!backlog.isEmpty()) {
return backlog.remove(0);
}
return null;
}
}
class TeamMember {
private String name;
public TeamMember(String name) {
this.name = name;
}
public void pullAndWorkOnTask(TaskBoard taskBoard) {
Task task = taskBoard.pullTask();
if (task != null) {
System.out.println(name + " pulled and started working on task: " + task.getDescription());
} else {
System.out.println("No tasks available for " + name + " to work on at the moment.");
}
}
}
public class AgileTaskManagement {
public static void main(String[] args) {
TaskBoard taskBoard = new TaskBoard();
taskBoard.addTaskToBacklog(new Task("Implement user authentication"));
taskBoard.addTaskToBacklog(new Task("Refactor database access layer"));
TeamMember member1 = new TeamMember("Alice");
TeamMember member2 = new TeamMember("Bob");
member1.pullAndWorkOnTask(taskBoard);
member2.pullAndWorkOnTask(taskBoard);
}
}
In this Java example, the TaskBoard
represents the backlog of tasks available for the team to pull from. TeamMember
instances can pull tasks from the backlog and start working on them based on their availability. This pull-based approach empowers team members to take ownership of their work and contributes to a more autonomous and agile project environment.
Key Benefits of Pull Strategy in Agile Projects
- Autonomy and Ownership: Team members have the freedom to choose tasks based on their expertise and availability, fostering a sense of ownership and accountability.
- Flexibility and Adaptability: The pull strategy allows for increased flexibility, enabling the team to adapt to changing priorities and requirements more effectively.
- Enhanced Collaboration: By empowering team members to pull work, the approach encourages collaboration and knowledge sharing, leading to better outcomes for the project.
Final Considerations
In the Agile project management landscape, understanding the nuances of push and pull strategies is essential for maximizing project success. While push strategies may have their time and place, the pull approach aligns closely with Agile principles, promoting autonomy, collaboration, and adaptability. By choosing the right strategy based on the project context and dynamics, and potentially adopting a hybrid approach, Agile projects can unleash the full potential of their teams and achieve remarkable outcomes.
Embracing a pull strategy in Agile projects isn't just about code; it's about fostering a culture of empowerment and collaboration that propels projects towards success.
When you need further guidance on implementing Agile methodologies, tools, and best practices for Java development, check out this in-depth guide to Agile software development and explore how Java enables Agile teams to thrive.
Remember, success in Agile project management often hinges on the delicate balance between pushing for results and pulling for empowerment.