Slash Project Costs: The Hidden Impact of Delay!
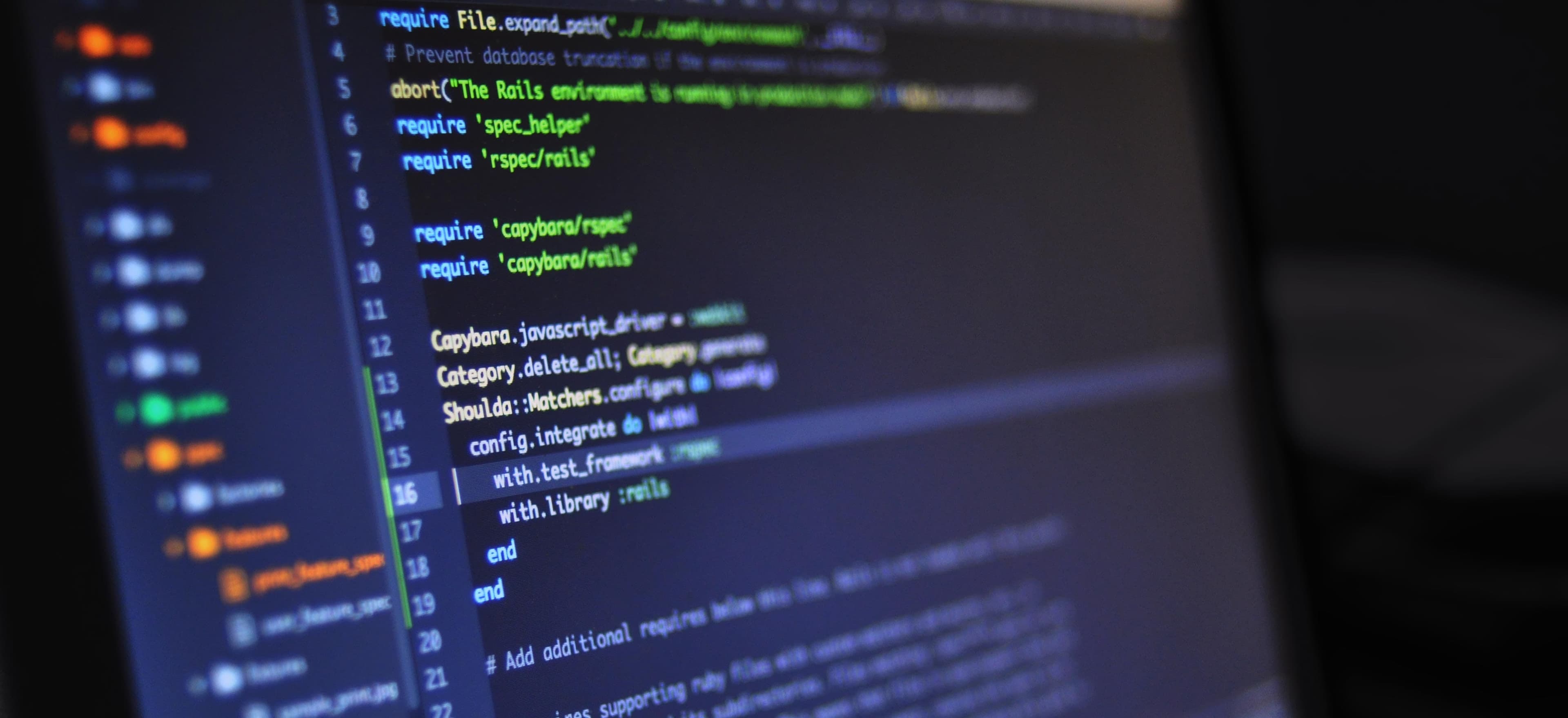
- Published on
The Hidden Impact of Delay on Project Costs
As a Java developer, you understand the critical nature of project timelines and the impact of delays on the overall cost of a project. In this post, we'll delve into the often underestimated costs associated with project delays and explore how Java development can help mitigate these challenges.
Understanding the Impact of Delay
Delay in software development projects can significantly impact the overall cost in several ways. First and foremost, delayed timelines often lead to increased labor costs. Developers, testers, and other team members continue to work on the project, potentially even incurring overtime costs, stretching the project budget thin.
Furthermore, delayed projects can result in missed market opportunities, leading to potential revenue loss. The longer it takes to bring a product to market, the longer it takes to start reaping the financial benefits. In addition, delays can also result in increased opportunity costs, as resources that could be allocated to other projects are tied up in the delayed endeavor.
How Java Development Can Mitigate Project Delays
Java, known for its robustness and versatility, offers several features and tools that can help mitigate the impact of project delays.
1. Code Reusability
Java's emphasis on code reusability allows developers to leverage existing code components and libraries, streamlining development and reducing the time required to build new features. By reusing code, developers can expedite the development process, mitigating the risk of delays and associated cost implications.
// Example of code reusability in Java
public class ReusableComponent {
public void performCommonTask() {
// Common task implementation
}
}
public class FeatureA {
private ReusableComponent component;
public FeatureA(ReusableComponent component) {
this.component = component;
}
public void useReusableComponent() {
component.performCommonTask();
}
}
In the above example, the ReusableComponent
class encapsulates a common task that can be reused across multiple features, showcasing the power of code reusability in Java.
2. Robust Exception Handling
Java's robust exception handling mechanisms enable developers to anticipate and handle errors effectively, reducing the likelihood of unexpected issues derailing the project timeline. By addressing potential issues proactively, Java development can contribute to smoother project execution and minimize the impact of delays caused by unhandled exceptions.
// Example of robust exception handling in Java
try {
// Risky operation
} catch (SpecificException ex) {
// Exception handling
} catch (AnotherException ex) {
// Exception handling
} finally {
// Cleanup code
}
The above code snippet demonstrates how Java's try-catch-finally block facilitates effective exception handling, promoting project stability and resilience in the face of potential setbacks.
3. Multi-threading Capabilities
Java's support for multi-threading allows developers to design concurrent, efficient applications. By leveraging multi-threading, Java applications can handle multiple tasks simultaneously, improving performance and responsiveness. This capability can contribute to optimized project timelines, reducing the risk of delays associated with sluggish application performance.
// Example of multi-threading in Java
public class ConcurrentTask implements Runnable {
public void run() {
// Task execution
}
}
public class Main {
public static void main(String[] args) {
Thread threadA = new Thread(new ConcurrentTask());
Thread threadB = new Thread(new ConcurrentTask());
threadA.start();
threadB.start();
}
}
In the above example, the ConcurrentTask
class implements the Runnable
interface to facilitate concurrent task execution, showcasing how Java's multi-threading capabilities can enhance project efficiency.
Leveraging Java for Project Success
By leveraging the inherent strengths of Java for development projects, teams can navigate challenges and mitigate the impact of delays on project costs. With its emphasis on code reusability, robust exception handling, and multi-threading capabilities, Java empowers developers to build resilient, efficient applications while adhering to project timelines and budget constraints.
In conclusion, understanding and addressing the hidden impact of delay on project costs is essential for successful project management. By incorporating Java's best practices and leveraging its powerful features, development teams can proactively minimize the risks associated with project delays and pave the way for successful, cost-effective project delivery.
Remember, the true cost of delay is not just the additional time and resources required to get back on track. It's also about missed opportunities, potential revenue loss, and the overall impact on the organization. By recognizing these implications and harnessing the capabilities of Java development, you can ensure that your projects stay on course, minimizing the hidden impact of delay on project costs.