Overcoming Twitter API Call Limits with Scala & Java
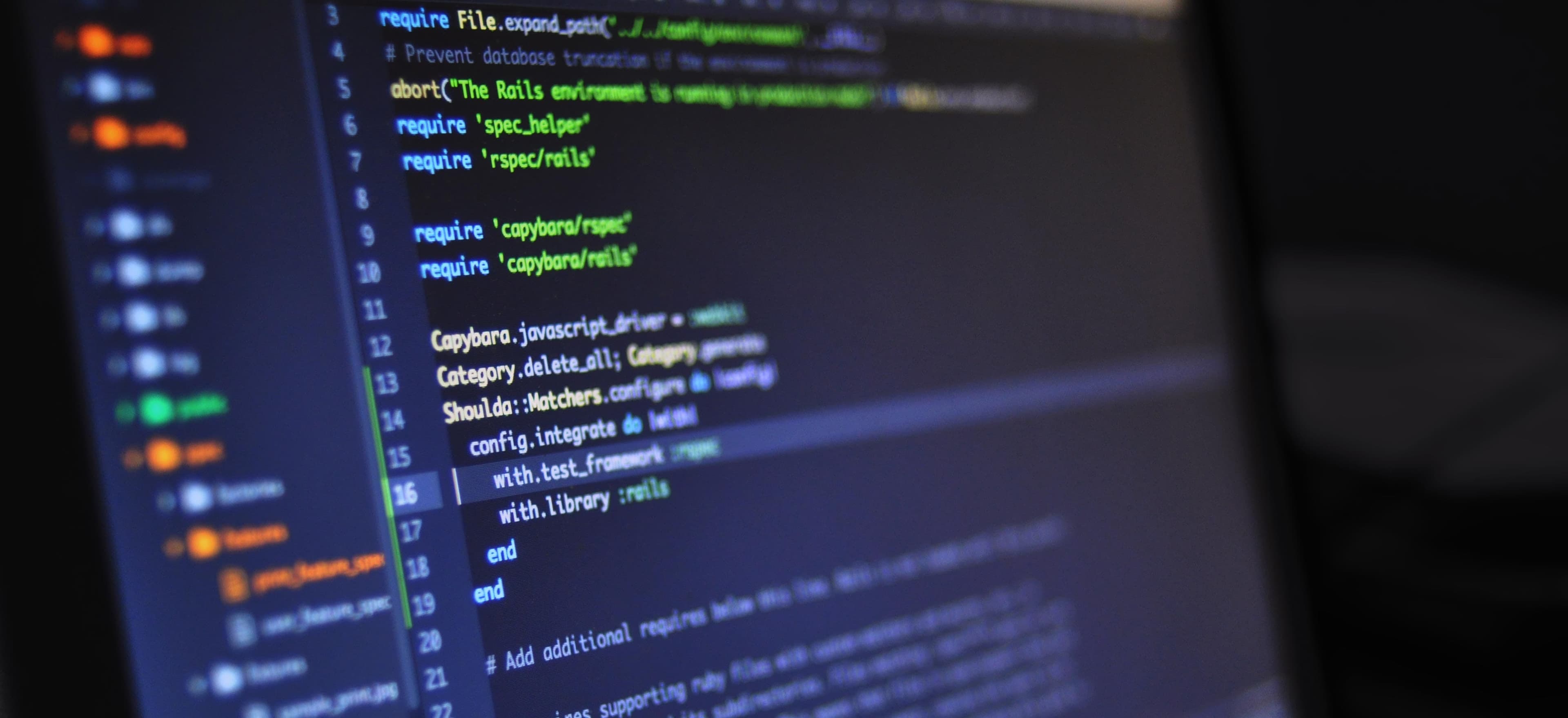
- Published on
Overcoming Twitter API Call Limits with Scala & Java: A Comprehensive Guide
In today’s social-driven world, Twitter stands out as a major platform for collecting vast amounts of real-time data for various uses, from sentiment analysis to brand monitoring. However, the Twitter API, like many other web APIs, imposes rate limits. These limitations can significantly throttle your data collection efforts, but with smart programming practices, we can effectively work around these constraints. This blog delves into how you can leverage Scala and Java to overcome Twitter API call limits, ensuring your data-driven projects remain unhindered.
Understanding Twitter API Rate Limits
Before diving into solutions, let’s clarify what we’re dealing with. Twitter's API rate limits are essentially thresholds set to prevent excessive use of their resources. For instance, the standard search API might limit you to 180 requests per 15-minute window. Exceeding these limits can result in your application being temporarily blocked from making further requests.
To navigate these waters effectively, visit Twitter’s official documentation on rate limits: Twitter API Rate Limits.
Leveraging Scala & Java: A Dual Approach
Why Scala and Java? Both languages run on the JVM (Java Virtual Machine), which means they can be used interchangeably in many scenarios. Java brings its robustness and vast ecosystem to the table, while Scala offers concise syntax and functional programming capabilities, making it ideal for data manipulation tasks.
Threading in Java: Maximizing Your API Call Efficiency
Java’s concurrency utilities can be a game-changer when dealing with rate limits. By spreading API calls across multiple threads, you can ensure you're utilizing your rate limit window to its fullest potential.
Consider a simple scenario where you need to fetch a large list of tweets. Instead of making sequential calls and risking hitting the rate limit, you can create multiple threads, each responsible for a portion of the task.
Example:
public class TwitterFetcher implements Runnable {
private String query;
public TwitterFetcher(String query) {
this.query = query;
}
@Override
public void run() {
// Your code to make API calls here
}
public static void main(String[] args) {
ExecutorService executor = Executors.newFixedThreadPool(5); // Using 5 threads for example
for(int i = 0; i < 5; i++) {
Runnable worker = new TwitterFetcher("java");
executor.execute(worker);
}
executor.shutdown();
while (!executor.isTerminated()) {
}
System.out.println("Finished all threads");
}
}
Why This Works: Distributing the workload over multiple threads minimizes the risk of hitting API limits for any single thread. It also ensures that while one thread is waiting for an API call to return, others are actively making additional requests.
Caveat:
While threading increases efficiency, it’s crucial to remain compliant with Twitter's rules to avoid being blacklisted. Always monitor your usage to ensure you’re within the limits.
Embracing Scala: Functional Programming to Manage Data
Scala's functional programming aspects become incredibly powerful when you need to process the data you receive from Twitter. Its ability to handle collections cleanly and concisely can save significant amounts of time and reduce boilerplate code.
For example, after fetching tweets, you might want to filter them based on certain criteria, such as hashtags or mentions. Scala lets you do this elegantly.
Example:
val tweets: List[Tweet] = // Assume this is your list of fetched tweets
val filteredTweets = tweets.filter(_.hashtags.contains("Scala"))
filteredTweets.foreach(println)
Why This Works: The filter method allows us to quickly sift through collections based on our specified conditions. This streamlined approach to data manipulation is where Scala shines, enabling more readable and maintainable code.
Combining Scala and Java
Given Scala’s interoperability with Java, you can easily integrate Scala’s concise and functional code into your Java projects. This is particularly useful for projects where Java's verbosity becomes a hindrance, or when leveraging existing Java libraries for Twitter API interactions.
Here's a simple integration technique:
- Use Java for threading and managing API calls, taking advantage of mature libraries and frameworks.
- Utilize Scala for data manipulation and processing, benefiting from its functional programming features and concise syntax.
This combination allows you to build efficient, scalable applications that can handle Twitter’s API rate limit challenges effectively.
Essential Tips for Managing Rate Limits
- Caching Results: Cache responses whenever possible to reduce redundant calls for the same data.
- Monitoring Usage: Keep a close eye on your API usage. Consider using Twitter’s “Application-only authentication” for higher rate limits where possible.
- Graceful Handling of Rate Limits: Implement logic to automatically detect rate limit errors and pause or slow down requests as needed.
In Conclusion, Here is What Matters
Overcoming Twitter’s API rate limits requires a strategic approach, leveraging both the functional prowess of Scala and the robustness of Java. By employing smart threading strategies in Java and embracing Scala for succinct data manipulation, developers can maximize their efficiency and stay within the imposed limits.
Remember, the objective is to be efficient and respectful of Twitter’s resources while extracting valuable data insights. By adhering to this guide, you're well on your way to achieving that balance, ensuring your data-driven projects can thrive without interruption.
For further reading on API management and optimization strategies, check out this resource: Effective API Management.
Keep experimenting, keep learning, and happy coding!
Checkout our other articles