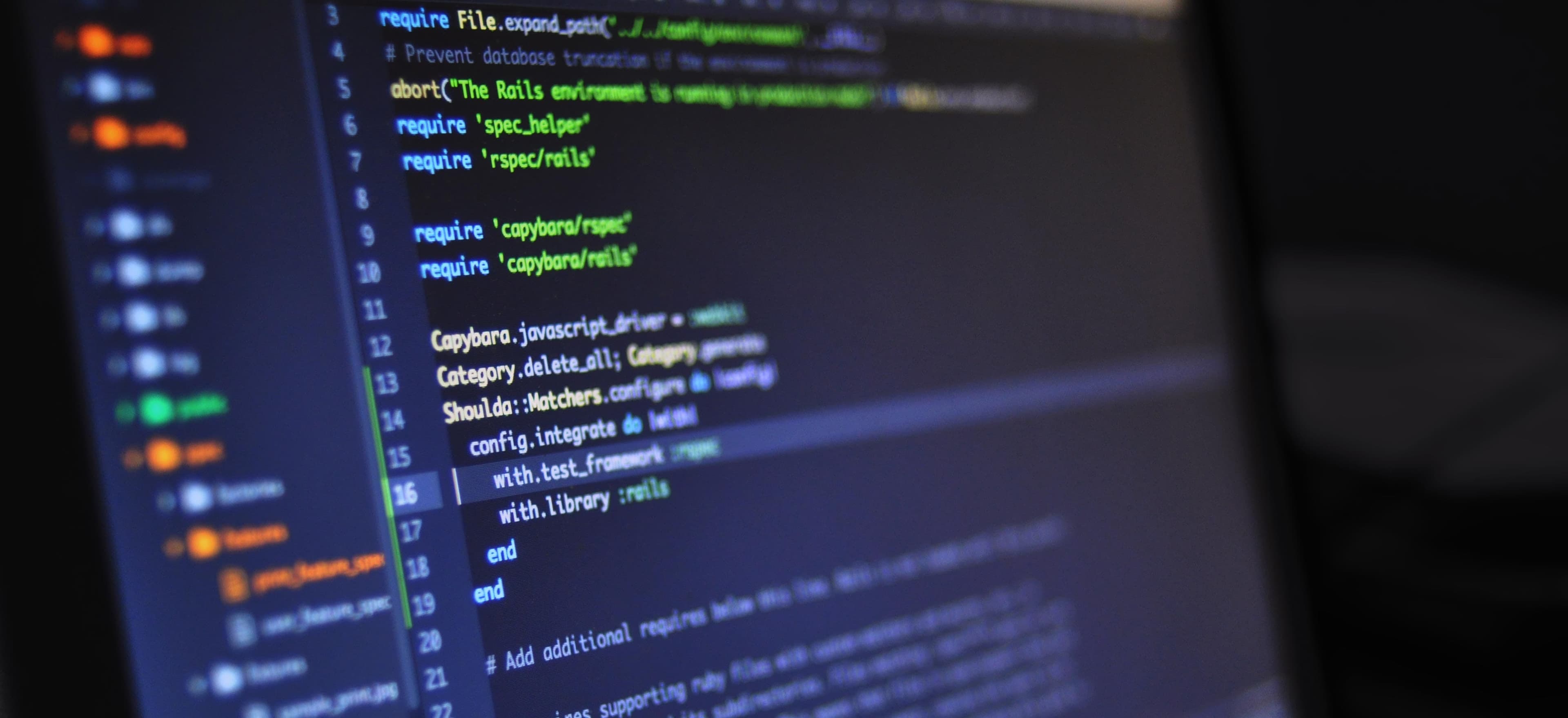
- Published on
Seamless Jersey & Spring MVC Integration: A How-To Guide
In the modern world of web development, building robust and scalable web applications is a necessity. Leveraging the power of Java and its various frameworks can help achieve this goal. One such powerful combination is the integration of Jersey, the reference implementation of JAX-RS, and Spring MVC. This integration allows developers to combine the strengths of both frameworks, resulting in a seamless and efficient web application architecture.
In this guide, we will walk through the process of integrating Jersey with Spring MVC, exploring the benefits and providing practical examples along the way.
Why Integrate Jersey with Spring MVC?
Before diving into the integration process, let's understand why integrating Jersey with Spring MVC can be beneficial. Both frameworks are widely used in the Java ecosystem.
Benefits of Jersey
Jersey provides a robust and efficient way to build RESTful web services in Java. It is compliant with the JAX-RS (Java API for RESTful Web Services) standard, offering a rich set of features for building and consuming RESTful APIs. Jersey comes with features such as resource classes, JAX-RS annotations, request and response entities, and more, making it a popular choice for implementing RESTful services.
Benefits of Spring MVC
On the other hand, Spring MVC is a part of the larger Spring Framework and is widely used for building web applications in Java. It provides a flexible and powerful model-view-controller architecture, along with features like request mapping, data binding, and form handling. Spring MVC's integration with other Spring modules, such as Spring Core and Spring Security, makes it a versatile choice for web application development.
By integrating Jersey with Spring MVC, developers can leverage the strengths of both frameworks. They can use Jersey for building RESTful services and Spring MVC for handling web requests, managing controllers, and integrating with other Spring features.
Getting Started with Jersey & Spring MVC Integration
Now that we understand the benefits of integrating Jersey with Spring MVC, let's dive into the process of setting up this integration.
Dependencies
To get started, we need to include the necessary dependencies in our project. We will use Maven for managing dependencies, but the same can be achieved with Gradle or any other build tool.
<dependencies>
<!-- Jersey dependencies -->
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet</artifactId>
<version>2.32</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.32</version>
</dependency>
<!-- Spring dependencies -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.9</version>
</dependency>
</dependencies>
In this example, we're including the Jersey Servlet container for integrating with Spring MVC, as well as the Jackson JSON provider for Jersey. Additionally, we're including the Spring Web and Spring Web MVC dependencies to enable Spring MVC integration.
Configuration
Once we have the necessary dependencies in place, we need to configure our application to integrate Jersey with Spring MVC.
Web.xml Configuration
We start by configuring the web.xml
file to define the DispatcherServlet
and map it to a URL pattern.
<web-app>
<servlet>
<servlet-name>JerseySpringIntegration</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>javax.ws.rs.Application</param-name>
<param-value>com.example.MyApplication</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>JerseySpringIntegration</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
</web-app>
In this configuration, we define the ServletContainer
provided by Jersey and specify its initialization parameters. We also map this servlet to a URL pattern (/api/*
in this example) that will be handled by Jersey.
Spring Configuration
Next, we need to configure Spring MVC by creating a DispatcherServlet
configuration.
public class WebAppInitializer implements WebApplicationInitializer {
@Override
public void onStartup(ServletContext container) {
AnnotationConfigWebApplicationContext ctx = new AnnotationConfigWebApplicationContext();
ctx.register(WebConfig.class);
container.addListener(new ContextLoaderListener(ctx));
ServletRegistration.Dynamic servlet = container.addServlet(
"dispatcher", new DispatcherServlet(ctx));
servlet.setLoadOnStartup(1);
servlet.addMapping("/");
}
}
Here, we create a class that implements WebApplicationInitializer
and configure the DispatcherServlet
with the context configuration.
Integration in Action
With the dependencies and configuration in place, we can now see the integration of Jersey with Spring MVC in action. Let's create a simple RESTful resource using Jersey and a controller using Spring MVC.
Jersey Resource
@Path("/hello")
public class HelloResource {
@GET
@Produces(MediaType.TEXT_PLAIN)
public String sayHello() {
return "Hello, Jersey!";
}
}
In this example, we define a simple HelloResource
using Jersey's annotations. The resource is mapped to the /hello
path and responds with a plain text "Hello, Jersey!".
Spring MVC Controller
@Controller
public class GreetingController {
@RequestMapping("/greet")
public ModelAndView greet() {
ModelAndView modelAndView = new ModelAndView("greet");
modelAndView.addObject("message", "Hello from Spring MVC");
return modelAndView;
}
}
Here, we create a GreetingController
using Spring MVC's @Controller
annotation. The controller responds to the /greet
path and returns a ModelAndView
with a message attribute.
With this integration, we can seamlessly use both Jersey and Spring MVC in the same application, leveraging the strengths of both frameworks.
In Conclusion, Here is What Matters
Integrating Jersey with Spring MVC allows developers to harness the power of both frameworks to build robust and feature-rich web applications. By following the steps outlined in this guide, you can seamlessly integrate Jersey with Spring MVC, enabling the development of RESTful services and web request handling in a single application.
While this integration provides flexibility and efficiency, it's important to consider the specific requirements of your project and choose the most suitable approach for web application development.
In conclusion, the combination of Jersey and Spring MVC offers a potent framework for crafting modern Java web applications, empowering developers to meet the demands of today's web development landscape.
By mastering the integration of Jersey with Spring MVC, you can elevate your Java web development skills and stay ahead in the ever-evolving world of web technologies.
Happy coding!
Checkout our other articles