Fixing Display Glitches in Android Game Development
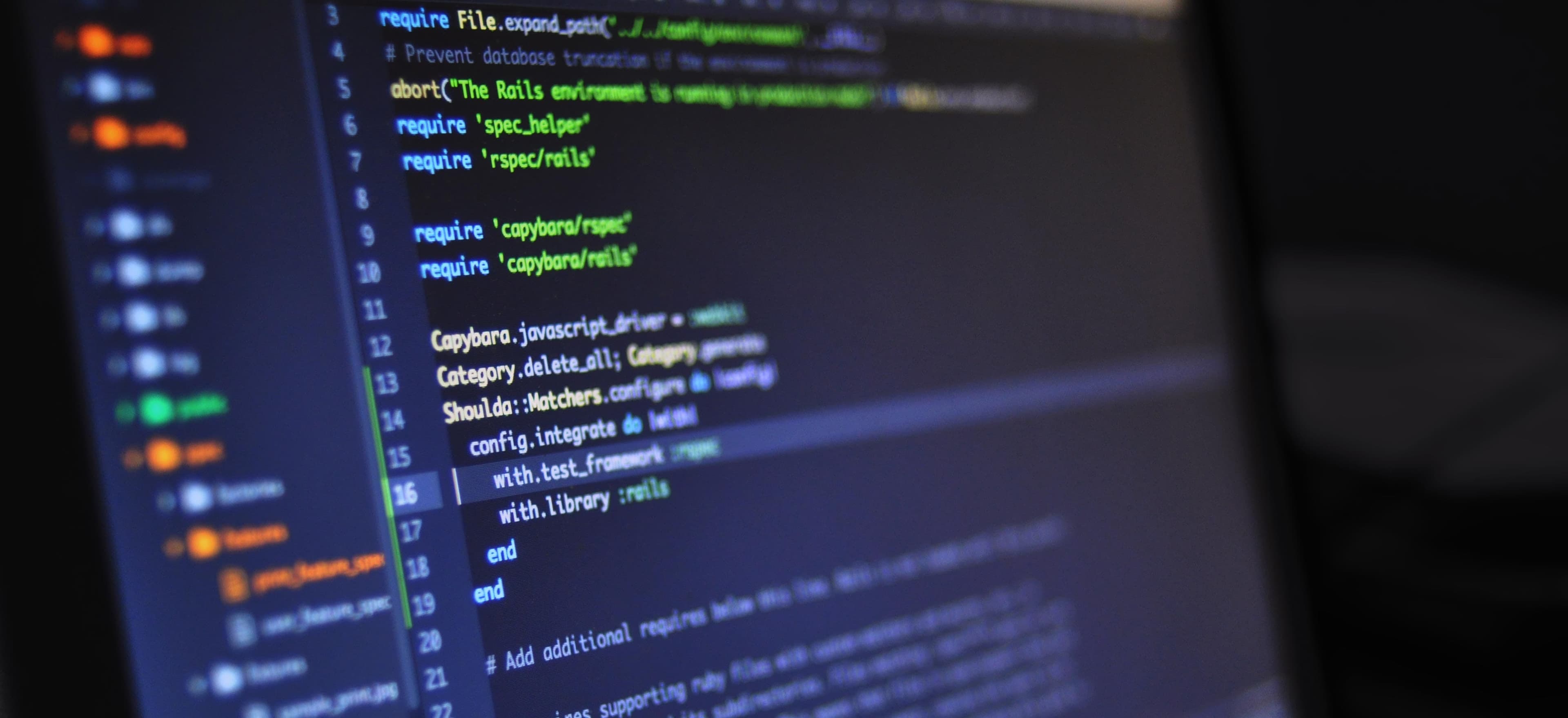
- Published on
Fixing Display Glitches in Android Game Development: A Comprehensive Guide
In Android game development, the visual allure of your game is just as crucial as its gameplay. Unfortunately, display glitches can mar this visual charm, turning an otherwise captivating gaming experience into a frustrating ordeal for players. These glitches can range from minor annoyances, like flickering textures, to game-breaking issues, such as missing UI elements or entire models. Therefore, addressing these issues swiftly and efficiently is paramount for maintaining your game's appeal and user satisfaction.
In this post, we'll dive deep into the common causes of display glitches in Android game development and outline effective strategies for identifying and resolving them. We'll leverage Java, the backbone of Android development, to demonstrate practical solutions that will enhance your debugging arsenal. Our goal is to provide you with actionable insights that will help you elevate your game's visual quality, ensuring a smooth and immersive experience for your players.
Understanding Display Glitches in Android Games
Before delving into the solutions, let's briefly discuss what display glitches are and why they occur. Display glitches can manifest in various forms, including but not limited to:
- Texture Flickering: Unstable rendering of textures, causing them to flicker.
- Missing Graphics: Invisible or missing game assets.
- Screen Tearing: Parts of the screen displaying frames out of sync.
- Incorrect Rendering: Objects appearing distorted or in the wrong place.
These issues often stem from improper handling of game assets, coding errors in the rendering pipeline, issues with the game engine, or even the hardware limitations of the device.
How to Identify Display Glitches
Identifying display glitches can be straightforward or incredibly challenging, depending on the nature of the glitch. Using tools like the Android Profiler in Android Studio can help monitor your game’s performance in real-time and identify potential bottlenecks that could lead to display issues. More information on the Android Profiler can be found in the official Android Studio documentation.
Moreover, thorough testing across a range of devices with varying hardware capabilities is essential. Since Android operates on a vast ecosystem of devices, what works flawlessly on one device might not on another.
Strategies for Fixing Display Glitches
1. Optimize Game Assets
Large or unoptimized game assets can cause performance bottlenecks leading to display glitches. Ensure that your textures and models are optimized for mobile devices without compromising quality. Tools like Android’s aapt (Android Asset Packaging Tool) can help you reduce the size of your assets.
2. Correcting Rendering Pipeline Errors
Errors in the rendering pipeline are a common cause of display glitches. This usually involves invalid calculations or improper state management in your game's rendering code.
Let’s examine a simple code snippet that corrects a common error in the rendering pipeline:
public void renderGameObjects() {
// Ensure the rendering pipeline is in the correct state
GLES20.glClearColor(0.0f, 0.0f, 0.0f, 1.0f);
GLES20.glClear(GLES20.GL_COLOR_BUFFER_BIT | GLES20.GL_DEPTH_BUFFER_BIT);
// Correctly set the view and projection matrices for your 3D space
Matrix.setLookAtM(viewMatrix, 0, eyeX, eyeY, eyeZ, centerX, centerY, centerZ, upX, upY, upZ);
Matrix.perspectiveM(projectionMatrix, 0, fieldOfView, aspectRatio, near, far);
// Render your game objects here
}
In this snippet, we first reset the color and depth buffers to ensure that we're starting with a clean slate for our scene. The view and projection matrices are then correctly set up, ensuring our game objects are rendered from the correct perspective. This precision avoids common glitches like rendering objects at incorrect depths or angles. For more information on OpenGL ES, you might want to explore its official documentation.
3. Debugging on Various Devices
Since Android games are played on a myriad of devices, it's crucial to test your game extensively across a range of devices. This can highlight specific issues that may only occur on certain hardware configurations.
One effective method for catching device-specific glitches is to use device farms, either physical or cloud-based, like Firebase Test Lab. This allows you to run your games on multiple devices simultaneously, speeding up the testing process.
4. Use Android's Built-in Debugging Tools
Android Studio comes packed with tools designed to help developers identify and fix display glitches. The GPU Debugger, for example, lets you step through your rendering code and visualize how your game’s frames are constructed. This can be invaluable for pinpointing the exact stage where a glitch occurs.
Best Practices Moving Forward
- Continuous Learning: The landscape of Android game development is always evolving. Keep abreast with the latest in game development techniques, OpenGL ES versions, and Android SDK tools.
- Community Engagement: Platforms like Stack Overflow and the Android Developer Community are invaluable resources. Engaging with these communities can provide insights and solutions from fellow developers who might have tackled similar issues.
- Iterative Testing: Adopt an iterative approach to testing and debugging. Fixing display glitches often requires multiple attempts and strategies before identifying a viable solution.
- Performance Profiling: Regularly profile your game’s performance, even if no immediate issues are evident. Catching potential problems early can save a significant amount of time and resources down the line.
Closing Remarks
Fixing display glitches in Android game development is a multifaceted challenge that requires a thorough understanding of your game's assets, code, and the Android ecosystem at large. By optimizing game assets, correcting rendering pipeline errors, conducting extensive device testing, and leveraging Android’s debugging tools, you can significantly improve your game's visual performance. Remember, the key to successfully resolving display glitches — and avoiding them in the future — lies in a combination of diligent testing, continuous learning, and community engagement. Happy coding!