Breaking Down Walls: Overcoming Unit Testing Barriers
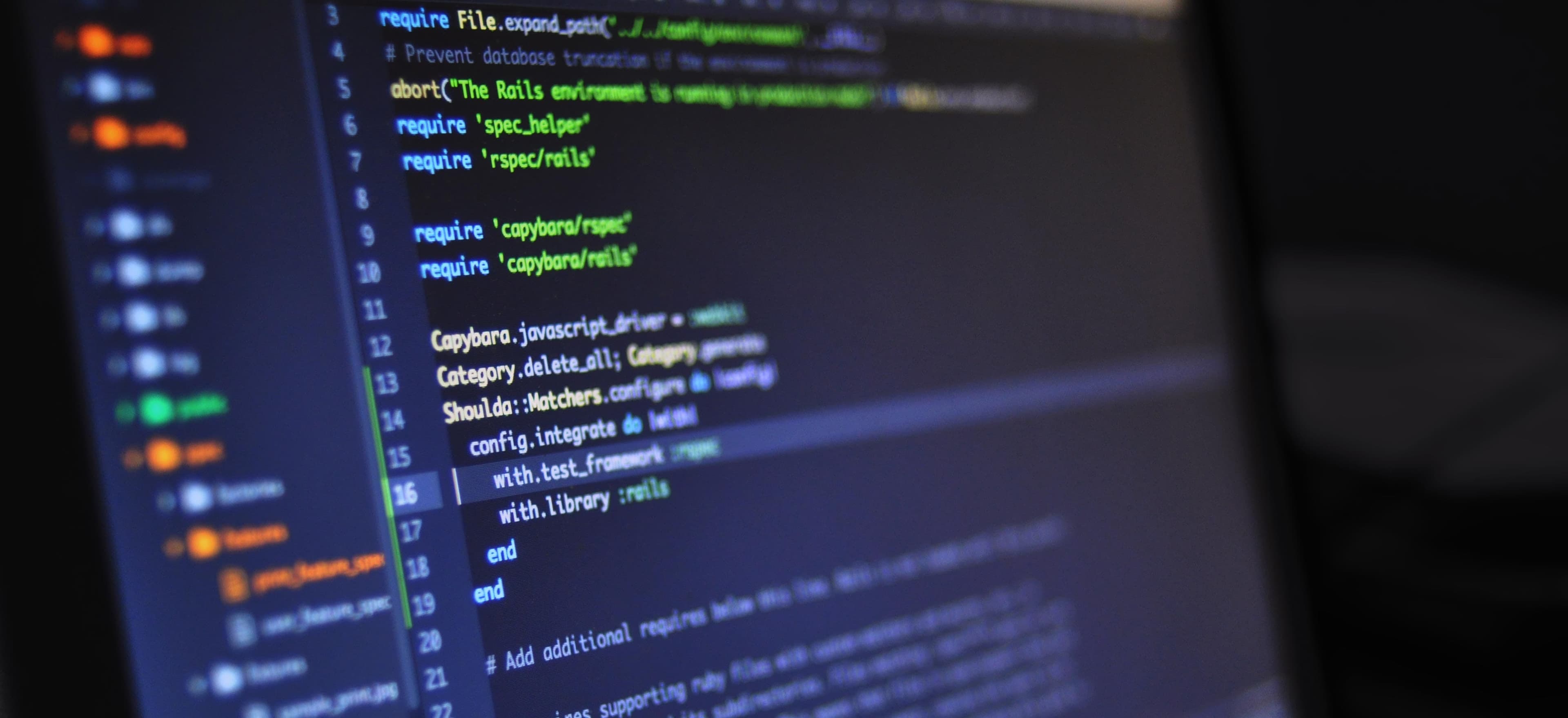
- Published on
Breaking Down Walls: Overcoming Unit Testing Barriers
In the world of Java development, unit testing plays a pivotal role in ensuring the quality and reliability of software applications. However, despite its importance, many developers encounter barriers that hinder their ability to effectively implement and maintain unit tests. In this article, we'll explore some of the common challenges associated with unit testing in Java and discuss strategies for overcoming these barriers.
The Importance of Unit Testing
Before delving into the challenges of unit testing, let's first reiterate its significance. Unit testing involves testing individual units or components of a software application in isolation to ensure they function as intended. This approach not only helps detect bugs early in the development process but also facilitates code maintainability and refactoring.
By writing unit tests, developers can gain confidence in the correctness of their code and streamline the debugging process. However, reaping these benefits hinges on the ability to overcome the obstacles that often impede successful unit testing practices.
Common Unit Testing Barriers in Java
Tight Coupling and Dependency Injection
One of the primary challenges in unit testing Java applications is dealing with tight coupling between classes and the lack of proper dependency injection. When classes are tightly coupled, it becomes difficult to isolate individual components for testing, leading to cumbersome and brittle test suites.
To address this issue, developers can leverage dependency injection frameworks like Spring or Guice to decouple classes and simplify the process of mocking dependencies during unit tests. By adopting a dependency injection approach, developers can effectively isolate units for testing without being hindered by tight coupling.
Legacy Code and Testability
Legacy code presents another significant barrier to effective unit testing. Oftentimes, legacy applications are riddled with code that was not designed with testability in mind, making it arduous to retrofit unit tests into such codebases.
In such scenarios, developers can employ techniques like refactoring and test-driven development (TDD) to gradually refactor and add tests to legacy code. By incrementally improving test coverage and refactoring for testability, developers can overcome the challenges posed by legacy code and pave the way for comprehensive unit testing.
Strategies for Overcoming Unit Testing Barriers
Embracing Test-Driven Development (TDD)
One effective strategy for overcoming unit testing barriers is to adopt a test-driven development approach. TDD involves writing tests before writing the actual code, thereby driving the design and ensuring testability from the outset.
By following TDD practices, developers can design cohesive and testable components while iteratively building up a suite of comprehensive unit tests. This approach not only fosters a testable design but also instills a testing mindset that permeates the entire development process, ultimately breaking down barriers to effective unit testing.
public class ExampleUnitTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertEquals(5, result);
}
}
In the above example, we can see a simple unit test for a Calculator
class, where the test is written before the implementation, in line with TDD principles.
Mocking Frameworks and Dependency Injection
To mitigate the impact of tight coupling and facilitate unit testing, the use of mocking frameworks such as Mockito and PowerMock can be instrumental. These frameworks enable developers to create mock objects for dependencies, thereby breaking dependencies and isolating units for testing.
Moreover, as mentioned earlier, embracing dependency injection frameworks like Spring or Guice can significantly enhance testability by decoupling classes and simplifying the process of injecting mock dependencies during unit tests.
public class ExampleServiceTest {
@Test
public void testBusinessLogic() {
ExampleDao mockDao = Mockito.mock(ExampleDao.class);
when(mockDao.retrieveData()).thenReturn("mockedData");
ExampleService service = new ExampleService(mockDao);
String result = service.performBusinessLogic();
assertEquals("expectedResult", result);
}
}
The above snippet demonstrates the use of Mockito to create a mock ExampleDao
and inject it into an ExampleService
for unit testing, effectively breaking dependencies and enabling isolated testing.
Continuous Integration and Automated Testing
Integrating unit testing into the continuous integration (CI) pipeline through tools like Jenkins or Travis CI can streamline the testing process and ensure that unit tests are run consistently with every code change.
By automating the execution of unit tests as part of the CI process, developers can catch regressions early and maintain a high level of test coverage. This approach not only fosters a culture of testing but also provides immediate feedback on the correctness of code changes, thereby promoting confidence in the software's stability and reliability.
Closing the Chapter
Unit testing in Java presents various challenges, ranging from tight coupling and legacy code to testability and maintainability. However, by strategically leveraging approaches such as TDD, mocking frameworks, and CI integration, developers can overcome these barriers and establish robust unit testing practices.
Ultimately, effective unit testing is not just about writing tests—it's about cultivating a testing mindset, embracing best practices, and persistently breaking down the obstacles that stand in the way of comprehensive test coverage. By doing so, developers can fortify their codebases, foster a culture of quality, and deliver software solutions that are reliable and resilient in the face of change.
In conclusion, while unit testing barriers may loom large, the strategies and practices discussed in this article can empower developers to conquer these obstacles and elevate the quality of their Java applications through effective unit testing.