Acing Geek Interviews: The Ultimate Prep Guide
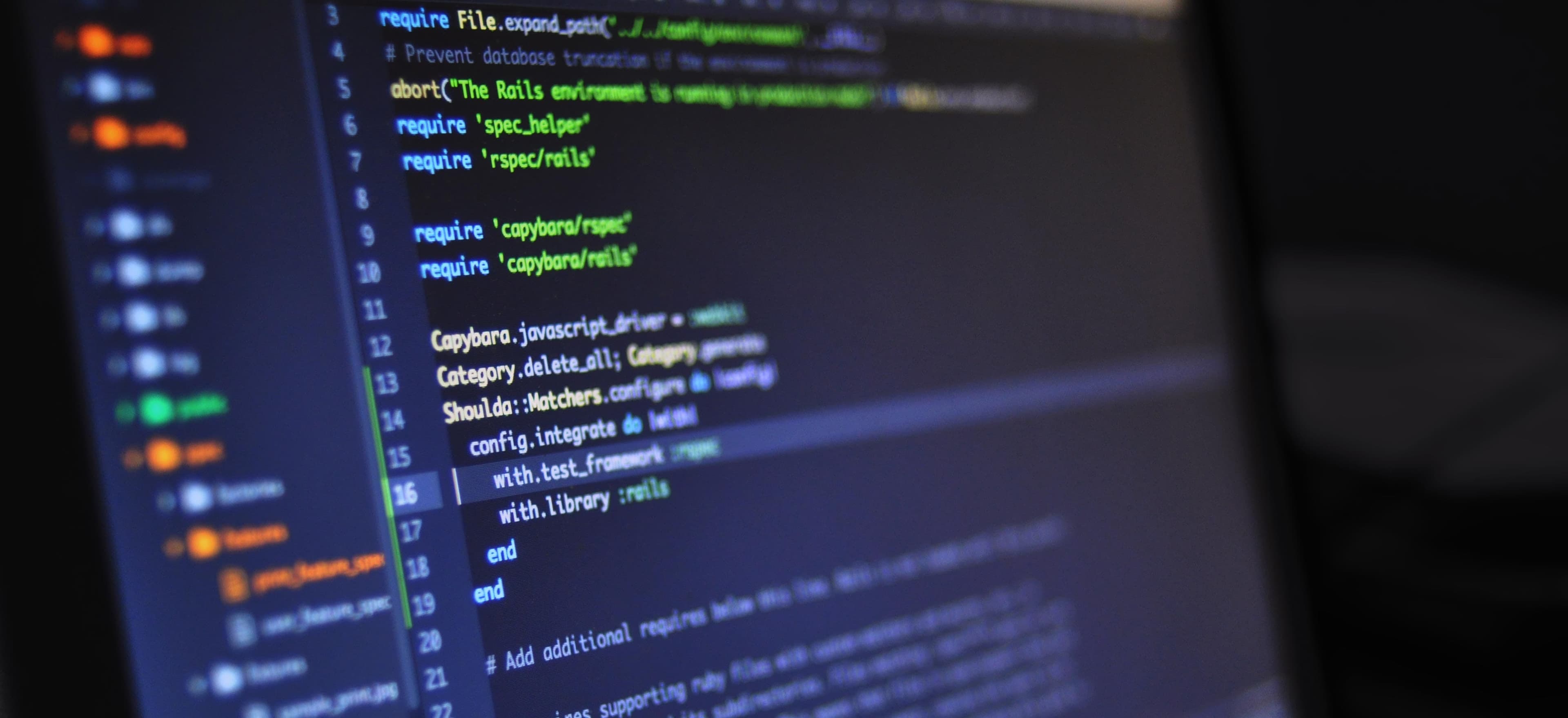
- Published on
Mastering Java Technical Interviews: A Complete Guide
Are you gearing up for a technical interview for a Java developer role? Are you looking to brush up on your Java skills and prep yourself to ace the interview process with confidence? If so, you're in the right place! This comprehensive guide will walk you through the essential aspects of preparing for a Java technical interview.
Understanding the Interview Process
Technical interviews for Java developers typically encompass a range of topics such as data structures, algorithms, object-oriented programming, design patterns, and Java-specific concepts. Additionally, you might encounter questions regarding multi-threading, exception handling, memory management, and performance optimization.
Sharpening Your Java Skills
To excel in a Java technical interview, it's crucial to have a strong grasp of the fundamentals. Brushing up on core Java concepts such as data types, control structures, and object-oriented principles is a great place to start. Additionally, understanding Java Collections Framework, I/O operations, and Java concurrency is pivotal.
Example:
public class QuickSort {
public void sort(int[] arr, int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
sort(arr, low, pi - 1);
sort(arr, pi + 1, high);
}
}
private int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j < high; j++) {
if (arr[j] < pivot) {
i++;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i + 1];
arr[i + 1] = arr[high];
arr[high] = temp;
return i + 1;
}
}
In the provided QuickSort
class, the sort
method implements the classic QuickSort algorithm, which is a fundamental sorting technique widely used in Java development. Understanding and being able to explain such algorithms in an interview context can significantly boost your standing as a candidate.
Emphasizing Problem-Solving & Algorithms
Technical interviews often involve solving algorithmic problems. It's imperative to practice solving various types of problems such as array manipulation, string manipulation, tree traversal, and searching algorithms. Additionally, understanding time and space complexity along with the efficiency of algorithms is essential.
Preparing for System Design Interviews
In addition to algorithmic problem-solving, many Java technical interviews include system design discussions. Familiarize yourself with concepts related to scalability, system architecture, database design, and microservices. It's beneficial to review design principles and patterns such as SOLID, DRY, and CQRS, and understand their application in Java-based systems.
Showcasing Your Java Knowledge
Interviewers often inquire about your experience with Java-related technologies and frameworks. Be prepared to discuss your proficiency with frameworks such as Spring, Hibernate, and Apache Wicket. Highlight your expertise in Java 8 features like streams, lambdas, and the new date and time API, as these are frequently used in modern Java development.
Practicing Mock Interviews
Engaging in mock interviews can significantly enhance your confidence and preparedness. Seek out platforms or peers to conduct mock interviews, and request constructive feedback. This process can help identify areas needing improvement, refine your communication skills, and enable you to experience an interview-like scenario, thus reducing anxiety during the actual interview.
The Closing Argument
In conclusion, mastering a Java technical interview requires a combination of solid theoretical understanding, practical problem-solving skills, and the ability to articulate your knowledge effectively. By thoroughly studying core Java concepts, practicing algorithmic problems, familiarizing yourself with system design principles, and honing your communication and interpersonal skills, you can significantly boost your chances of standing out in a competitive technical interview landscape.
Are you ready to take your Java technical interview preparation to the next level? With dedication, practice, and a strategic approach, you can confidently showcase your Java expertise and secure your dream job as a Java developer!
Checkout our other articles