Choosing Between WAR Files and Embedded Servers in Java Apps
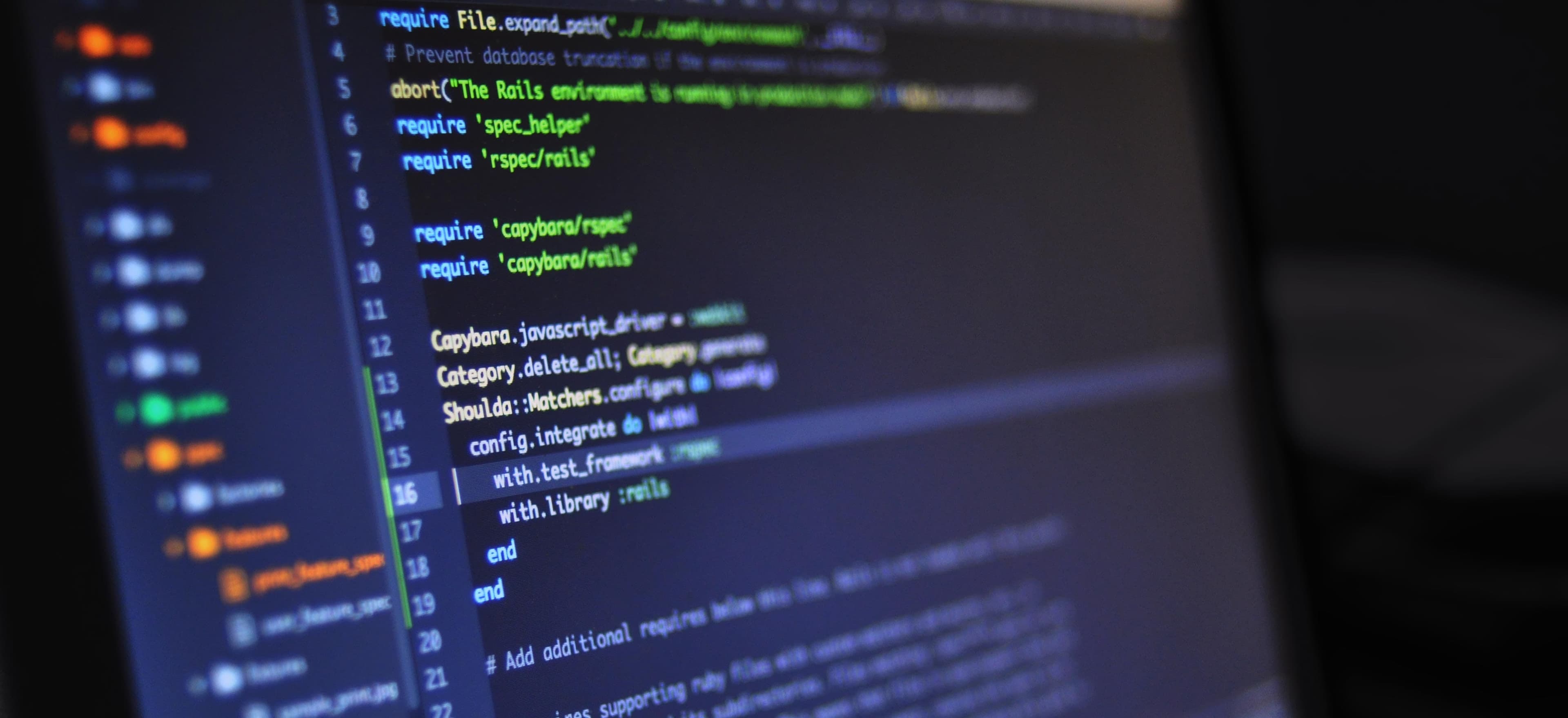
- Published on
Choosing Between WAR Files and Embedded Servers in Java Apps
Java is known for its flexibility and power in the world of web applications. When deploying Java applications, developers often face a pivotal decision: Should they use WAR (Web Application Archive) files or opt for embedded servers? Each approach has its own set of advantages and considerations, so it's essential to understand both before making a choice.
What are WAR Files?
A WAR file is essentially a packaged web application that contains all the necessary resources and configuration required to run on a server. This includes:
- Java classes
- JSP files
- Static resources (HTML, CSS, JavaScript)
- Required libraries (JAR files)
A WAR file allows developers to deploy applications on any Java EE compliant server, making it widely compatible.
Advantages of WAR Files
-
Separation of Concerns: WAR files promote the separation between application development and server management. The application can be developed independently and then deployed on a server without worrying about the server setup.
-
Standardization: As a standard deployment package, WAR files ensure that your Java web application can be easily shared and deployed across different environments.
-
Easy Scaling: Since WAR files are compatible with app servers like Tomcat, JBoss, or WildFly, it's straightforward to scale applications by deploying multiple instances of the WAR file across servers.
What are Embedded Servers?
Embedded servers, on the other hand, are integrated server instances bundled with the application itself. Popular frameworks like Spring Boot use embedded servers such as Tomcat, Jetty, or Undertow to run web applications.
Advantages of Embedded Servers
-
Simplicity in Deployment: With embedded servers, there’s no need to separately install and manage a web server. Your application can be run with a simple command.
-
Self-Contained Packages: The entire environment, including the server, is packaged with the application, creating a self-sufficient application that can run anywhere.
-
Faster Development Cycle: Developers can iterate faster as they have control over their deployment environment without needing server configurations.
WAR Files vs. Embedded Servers: A Comparison
| Feature | WAR Files | Embedded Servers | |-----------------------------|--------------------------------------------|---------------------------------------| | Deployment | Requires server installation | Standalone, just run the JAR file | | Configuration | Server configuration needed | Minimal configuration, ready to run | | Simplicity | More complex; involves more steps | Simple and straightforward | | Scaling | Easy to scale across multiple servers | More limited, but can be integrated |
When to Choose WAR Files
WAR files are often preferred for enterprise-grade applications where:
- Separation of Concerns: There is a clear distinction between application and server management.
- Existing Server Infrastructure: If the organization already has server infrastructure in place.
- Compliance and Standards: Compliance requirements dictate deployment on specific platforms.
Example: Deploying a WAR File on Apache Tomcat
To illustrate deploying a WAR file, we'll set up a simple Java web application and deploy it on Tomcat.
-
Create a Directory Structure:
myapp/ ├── WEB-INF/ │ ├── web.xml │ └── classes/ └── index.jsp
-
Sample web.xml:
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1"> <servlet> <servlet-name>MyServlet</servlet-name> <servlet-class>com.example.MyServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>MyServlet</servlet-name> <url-pattern>/hello</url-pattern> </servlet-mapping> </web-app>
-
Pack it into a WAR file:
Use the command to create a WAR file:
jar cvf myapp.war -C myapp .
-
Deploy on Tomcat:
Place the
myapp.war
file into thewebapps
directory of your Tomcat installation and start the server.
When to Choose Embedded Servers
Embedded servers are a better choice for scenarios such as:
- Microservices Architecture: Lightweight applications that can run in isolation.
- Rapid Prototyping: When speed of development is critical.
- Spring Boot Applications: Since Spring Boot's philosophy embraces convention over configuration, embedded servers are often the default choice.
Example: Running a Spring Boot Application
This is how an embedded server works in a typical Spring Boot application.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MyApplication {
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
In this case, when you build this application with Maven or Gradle, it packages an embedded Tomcat server. You can run your application simply with:
mvn spring-boot:run
Or by creating an executable JAR file:
mvn clean package
java -jar target/myapp-0.0.1-SNAPSHOT.jar
The Closing Argument
Choosing between WAR files and embedded servers can't be taken lightly. Both methods offer distinct pros and cons, often aligned with specific project requirements and team structures.
If you value isolation, simplicity, and modern development practices, embedded servers might be the most appealing option. However, if your project requires robust standards, enterprise management, and separation of concerns, WAR files are still an exceptional choice.
For more detailed insights on deploying Java applications, check out the Spring Boot documentation and Apache Tomcat documentation.
The best way forward often lies in your project's needs, team expertise, and long-term maintainability. Make your choice wisely, and happy coding!
Checkout our other articles