Simplify Selenium Tests: Tackling Multiple Browser Mayhem
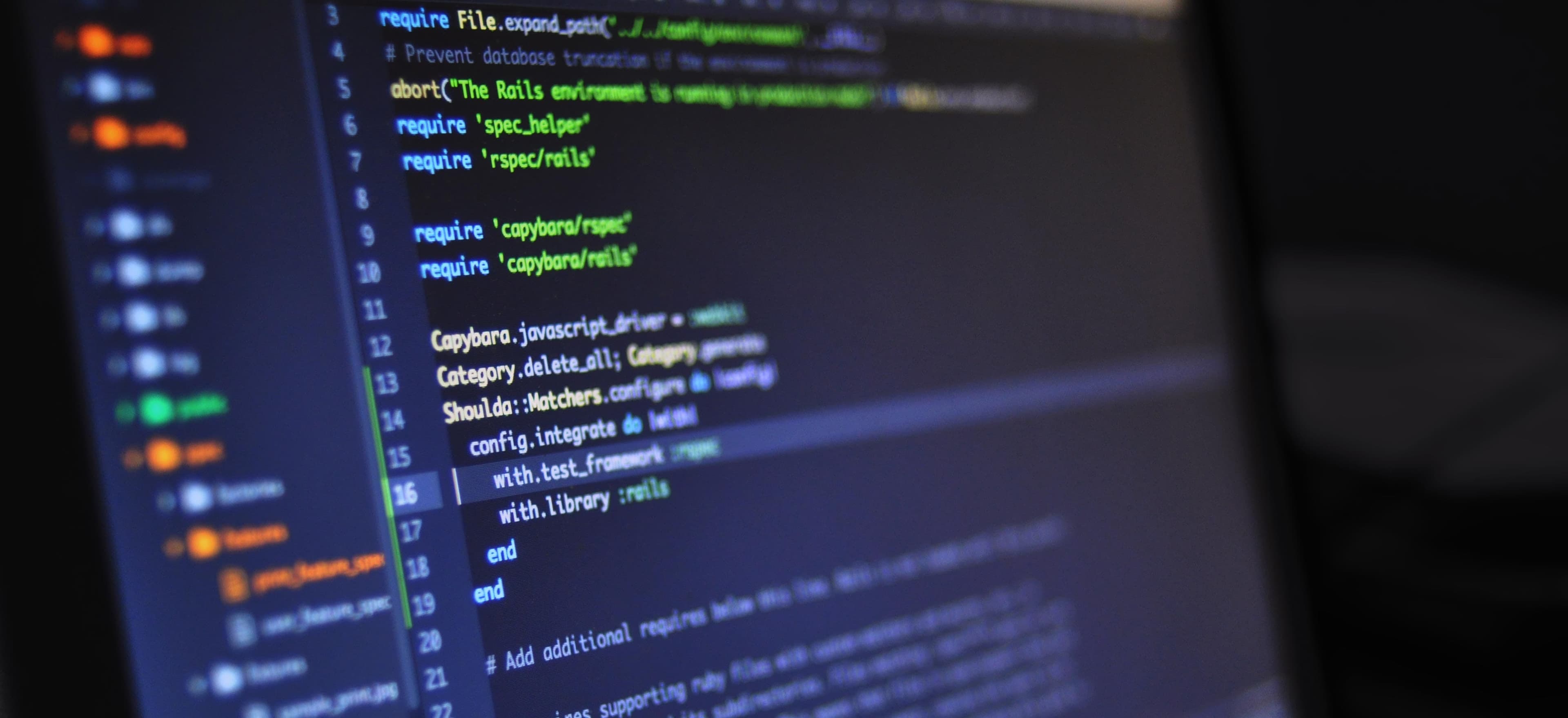
- Published on
Simplify Selenium Tests: Tackling Multiple Browser Mayhem
When it comes to automated testing of web applications, using Selenium for cross-browser testing is a must. However, managing tests across different browsers can be challenging and time-consuming. In this blog post, we will explore how to simplify Selenium tests, specifically tackling the mayhem of testing across multiple browsers.
The Challenge of Multiple Browser Testing
Testing web applications across multiple browsers is crucial to ensure a consistent user experience. However, writing and maintaining Selenium tests for different browsers can become complex and cumbersome. Each browser may have its unique quirks, and ensuring that tests run smoothly across all target browsers adds an extra layer of complexity to the test suite.
Simplifying Selenium Tests
1. Utilize WebDriver Factory
One common approach to managing tests across multiple browsers is to use a WebDriver factory. This factory pattern allows you to create WebDriver instances based on the browser type required for the test. Let's take a look at a simple example:
public class WebDriverFactory {
public static WebDriver createWebDriver(String browserType) {
if (browserType.equalsIgnoreCase("chrome")) {
return new ChromeDriver();
} else if (browserType.equalsIgnoreCase("firefox")) {
return new FirefoxDriver();
} else if (browserType.equalsIgnoreCase("edge")) {
return new EdgeDriver();
} else {
throw new IllegalArgumentException("Invalid browser type: " + browserType);
}
}
}
By using a WebDriver factory, you can easily instantiate the appropriate WebDriver based on the browser type needed for your test, reducing the boilerplate code and improving maintainability.
2. Leverage Configuration Files
Another effective way to simplify Selenium tests for multiple browsers is by utilizing configuration files. Instead of hardcoding browser types and their respective WebDriver instantiation in the test code, you can externalize this configuration into a properties file, XML, or JSON file. This allows for easier management of browser configurations without touching the test code.
3. Cross-Browser Testing Tools
Consider using cross-browser testing tools such as BrowserStack or Sauce Labs. These platforms offer a cloud-based Selenium grid that allows you to run tests across various browsers and devices concurrently. By leveraging these tools, you can offload the infrastructure setup and focus on writing and maintaining your tests.
In Conclusion, Here is What Matters
In conclusion, simplifying Selenium tests for multiple browsers is essential for efficient test maintenance and reliability. By employing strategies such as WebDriver factory, configuration files, and cross-browser testing tools, you can streamline your test suite and tackle the mayhem of testing across multiple browsers with ease.
For further reading on Selenium testing and browser automation, refer to the Selenium official documentation.
Streamline your Selenium tests today and say goodbye to multiple browser mayhem!
Checkout our other articles