Why Use Featured Enums Over Traditional Switch Statements?
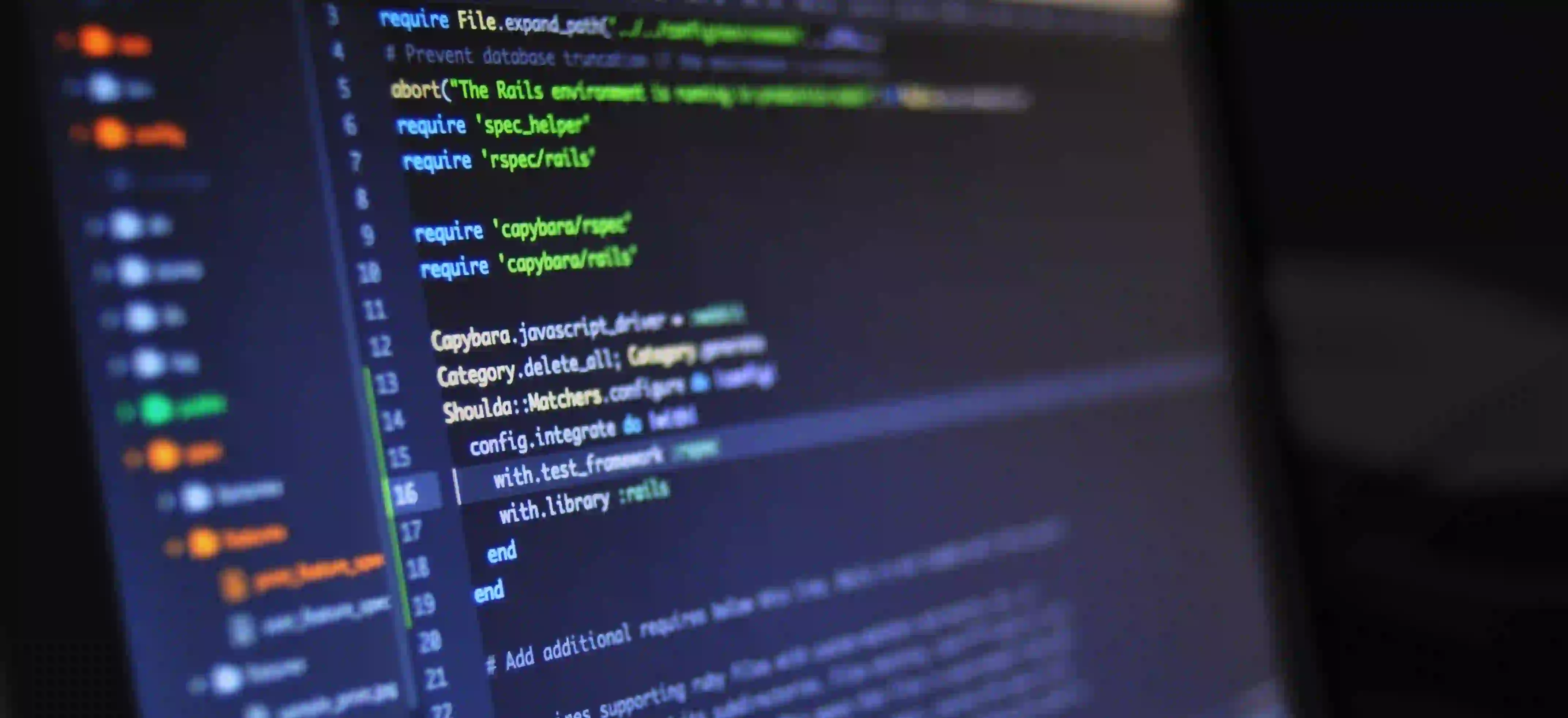
The Power of Enums in Java: Making the Case for Featured Enums
In the world of Java programming, the enum
type is a powerful tool that can be harnessed in various ways to enhance the readability, maintainability, and performance of your code. Particularly, featured enums bring significant advantages over traditional switch statements, making them an invaluable resource for Java developers. In this post, we will explore the benefits of using featured enums and understand why they make a compelling choice over conventional switch statements.
Understanding Traditional Switch Statements
In Java, switch statements are commonly used to perform different actions based on the value of a specific variable or expression. While switch statements offer a degree of control flow, they can become unwieldy and difficult to maintain as the number of cases grows. Additionally, they may not be easily extensible, and handling complex scenarios can result in convoluted code.
Consider the following example of a traditional switch statement:
public class SwitchExample {
public String getDayOfWeek(int day) {
String dayOfWeek;
switch (day) {
case 1:
dayOfWeek = "Monday";
break;
case 2:
dayOfWeek = "Tuesday";
break;
// Additional cases for the rest of the week
default:
dayOfWeek = "Invalid day";
break;
}
return dayOfWeek;
}
}
While the above code accomplishes the task, it becomes less manageable when more cases are added. This is where featured enums come into play, offering a more elegant and maintainable solution.
Leveraging Featured Enums
Improving Readability
Featured enums provide a way to represent a fixed set of constants, offering improved readability and context to your code. By using enums, you can clearly define and encapsulate a collection of related constants, making the code more expressive and self-documenting. For instance, let's redefine the getDayOfWeek
method using a featured enum:
public enum DayOfWeek {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY;
}
public class EnumExample {
public String getDayOfWeek(DayOfWeek day) {
// Perform operations based on the enum value
return day.toString();
}
}
By leveraging enums, the code becomes more readable and intuitive, as the enum values clearly represent the days of the week.
Ensuring Type Safety
One of the key advantages of featured enums is the inherent type safety they provide. Unlike traditional switch statements, which rely on integer-based cases, enums allow for a strict type check. This means that the compiler can catch errors at compile time, ensuring that only valid enum values are used in the code.
Adding Behavior to Enum Constants
Featured enums can also encapsulate behavior within each constant, allowing for enhanced modularity and extensibility. By adding methods to enum constants, you can define specific behavior for each constant, enabling a more object-oriented approach. Let's expand our DayOfWeek
enum to include a method that returns the abbreviated name of the day:
public enum DayOfWeek {
MONDAY("Mon"), TUESDAY("Tue"), WEDNESDAY("Wed"), THURSDAY("Thu"), FRIDAY("Fri"), SATURDAY("Sat"), SUNDAY("Sun");
private final String abbreviation;
DayOfWeek(String abbreviation) {
this.abbreviation = abbreviation;
}
public String getAbbreviation() {
return abbreviation;
}
}
public class EnumExample {
public String getAbbreviatedDay(DayOfWeek day) {
return day.getAbbreviation();
}
}
By enhancing the enum with behavior, we have created a more cohesive and maintainable solution compared to using a switch statement.
Switching to Featured Enums: A Compelling Choice
In summary, the use of featured enums in Java offers a range of benefits over traditional switch statements. By leveraging enums, you can improve the readability, ensure type safety, and encapsulate behavior within the constants, resulting in more maintainable and extensible code.
So, the next time you find yourself considering a switch statement in your Java code, pause for a moment and evaluate whether a featured enum could provide a more elegant and efficient solution. Chances are, it will not only streamline your code but also enhance its robustness and clarity.
By incorporating featured enums into your Java development repertoire, you can elevate the quality and maintainability of your code while embracing a more structured and object-oriented approach. The superiority of featured enums over traditional switch statements lies in their ability to enhance readability, ensure type safety, and encapsulate behavior within constants. If you are ready to refine your Java programming skills and harness the full potential of enums, the journey starts with embracing the power of featured enums.