Why the Strategy Pattern Clashes with Spring's Charm
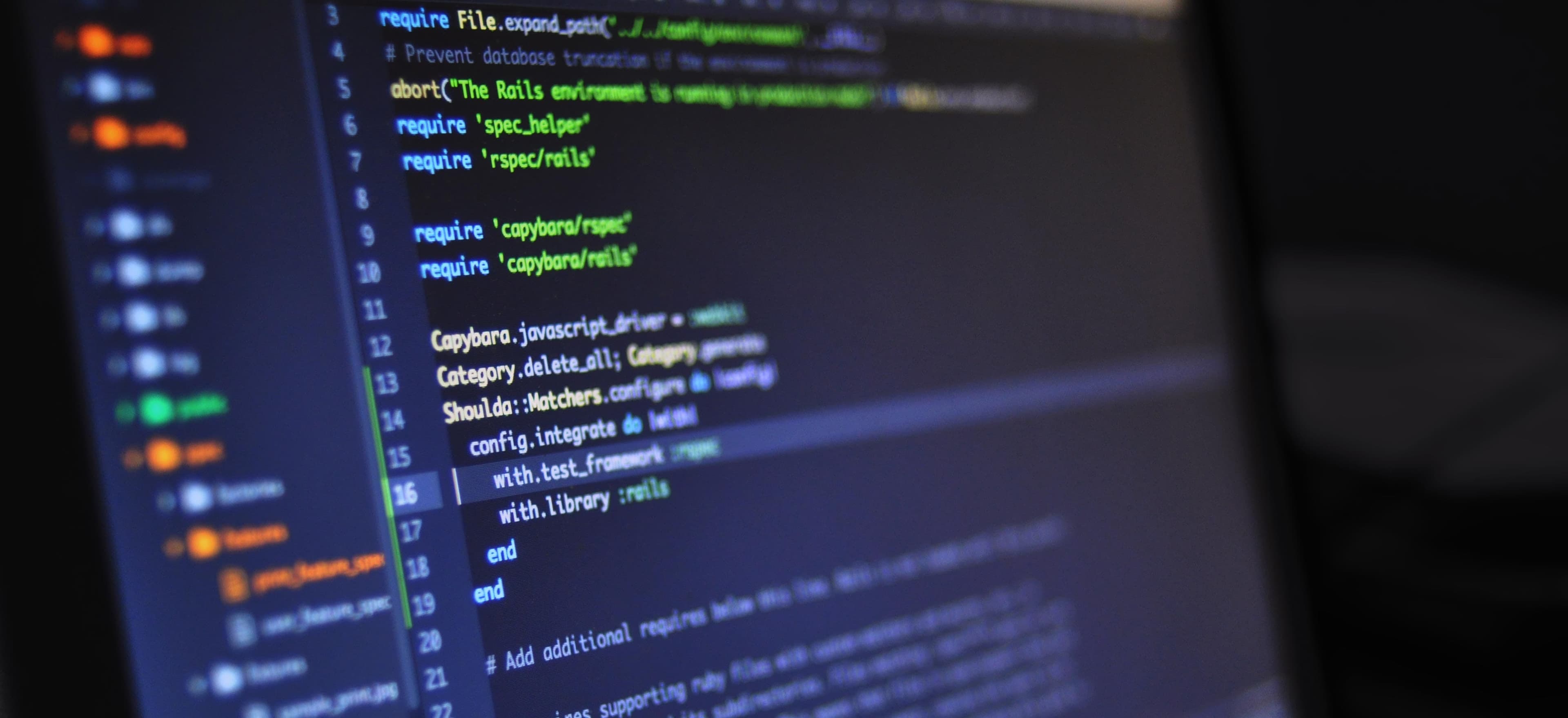
- Published on
Why the Strategy Pattern Clashes with Spring's Charm
When it comes to developing enterprise-level applications in Java, the Spring Framework has established itself as a leading choice due to its robust features and ease of use. However, there are times when certain design patterns, such as the Strategy Pattern, can clash with Spring's inherently charming features. In this blog post, we will delve into the conflict between the Strategy Pattern and Spring, and explore potential solutions to reconcile these differences.
Understanding the Strategy Pattern
Before we delve into the clash with Spring, let's first understand the Strategy Pattern. The Strategy Pattern is a behavioral design pattern that enables defining a family of algorithms, encapsulating each one, and making them interchangeable. This pattern allows the algorithm to vary independently from clients that use it, promoting a clean and maintainable codebase.
An example of the Strategy Pattern in action can be seen in the implementation of different sorting algorithms. By encapsulating each sorting algorithm (such as Bubble Sort, Quick Sort, or Merge Sort) within its own strategy class, the client can utilize these algorithms interchangeably without modifying the client code.
Here's a simple example of the Strategy Pattern in Java:
// Define the strategy interface
public interface SortingStrategy {
void sort(int[] array);
}
// Implement different sorting strategies
public class BubbleSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement Bubble Sort algorithm
}
}
public class QuickSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement Quick Sort algorithm
}
}
// Client class using the strategy
public class SortClient {
private SortingStrategy sortingStrategy;
public SortClient(SortingStrategy sortingStrategy) {
this.sortingStrategy = sortingStrategy;
}
public void performSort(int[] array) {
sortingStrategy.sort(array);
}
}
In the above example, the SortClient
class can utilize different sorting strategies interchangeably without altering its core implementation.
Spring's Dependency Injection and the Clash with Strategy Pattern
Spring Framework's core functionality revolves around the concept of dependency injection (DI), promoting loose coupling and facilitating easier unit testing. However, when using the Strategy Pattern with Spring's dependency injection, a clash arises.
One of the primary issues is that the Strategy Pattern promotes creating separate strategy classes for each algorithm, often leading to a proliferation of classes. While this approach promotes clean and modular code, it contradicts Spring's philosophy of managing beans through configuration and dependency injection.
Additionally, the Strategy Pattern often involves selecting and using a specific strategy at runtime based on certain conditions. This dynamic nature of strategy selection is not easily harmonized with Spring's typical approach of configuring and initializing beans at application startup.
The Interface-Driven Approach to Strategy Pattern in Spring
To reconcile the clash between the Strategy Pattern and Spring, an interface-driven approach can be adopted. Instead of defining separate strategy classes for each algorithm, we can leverage the flexibility of interfaces and integrate them seamlessly with Spring's DI mechanism.
Let's revisit the sorting strategy example using the interface-driven approach:
// Define the strategy interface
public interface SortingStrategy {
void sort(int[] array);
}
// Bubble Sort implementation
@Component("bubbleSortStrategy")
public class BubbleSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement Bubble Sort algorithm
}
}
// Quick Sort implementation
@Component("quickSortStrategy")
public class QuickSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement Quick Sort algorithm
}
}
// Client class using the strategy with Spring DI
@Component
public class SortClient {
private final SortingStrategy sortingStrategy;
// Inject the strategy based on the implementation
@Autowired
public SortClient(@Qualifier("quickSortStrategy") SortingStrategy sortingStrategy) {
this.sortingStrategy = sortingStrategy;
}
public void performSort(int[] array) {
sortingStrategy.sort(array);
}
}
In this revised example, the SortingStrategy
interface is utilized to abstract the sorting algorithm, and concrete implementations such as BubbleSortStrategy
and QuickSortStrategy
are annotated as Spring components. The SortClient
class leverages constructor injection to dynamically select the sorting strategy based on the specific implementation annotated with the @Qualifier
annotation.
By utilizing Spring's dependency injection with an interface-driven approach to the Strategy Pattern, we can elegantly manage and integrate the strategy implementations while adhering to Spring's DI principles.
Enabling Runtime Strategy Selection with Spring Profiles
In scenarios where the selection of a strategy needs to be determined at runtime based on specific conditions, Spring provides a powerful feature known as profiles. Spring profiles allow for the conditional activation of beans based on the active profile, providing a solution for dynamic strategy selection.
Let's consider an example where different sorting strategies need to be selected based on the size of the input array:
// Interface-driven strategy implementations with Spring components
@Profile("smallArray")
@Component("bubbleSortStrategy")
public class BubbleSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement Bubble Sort algorithm for small arrays
}
}
@Profile("largeArray")
@Component("quickSortStrategy")
public class QuickSortStrategy implements SortingStrategy {
@Override
public void sort(int[] array) {
// Implement Quick Sort algorithm for large arrays
}
}
// Client class using the strategy with Spring DI and profile-based selection
@Component
public class SortClient {
private final SortingStrategy smallArraySortingStrategy;
private final SortingStrategy largeArraySortingStrategy;
@Autowired
public SortClient(@Qualifier("bubbleSortStrategy") SortingStrategy smallArraySortingStrategy,
@Qualifier("quickSortStrategy") SortingStrategy largeArraySortingStrategy) {
this.smallArraySortingStrategy = smallArraySortingStrategy;
this.largeArraySortingStrategy = largeArraySortingStrategy;
}
public void performSort(int[] array) {
if (array.length < 100) {
smallArraySortingStrategy.sort(array);
} else {
largeArraySortingStrategy.sort(array);
}
}
}
In this example, the BubbleSortStrategy
is associated with the "smallArray" profile, while the QuickSortStrategy
is linked to the "largeArray" profile. The SortClient
class uses constructor injection to inject the respective strategies based on the active profile. At runtime, the appropriate strategy is selected depending on the size of the input array.
By leveraging Spring profiles, we can achieve dynamic strategy selection based on specific runtime conditions, harmonizing the dynamic nature of the Strategy Pattern with Spring's powerful DI capabilities.
Wrapping Up
While the Strategy Pattern and Spring may seemingly clash due to their differing philosophies, adopting an interface-driven approach and harnessing Spring's advanced features such as dependency injection and profiles can effectively bridge the gap.
By understanding the intricacies of both the Strategy Pattern and Spring's DI mechanisms, developers can harmoniously integrate these concepts to create flexible, maintainable, and dynamic solutions for a wide range of use cases.
Incorporating the Strategy Pattern within the Spring ecosystem not only enhances the modularity and extensibility of the application but also showcases the adaptability and versatility of Spring's feature set.
In your experience, have you encountered conflicts between design patterns and frameworks? How did you reconcile them? Share your thoughts and experiences in the comments below.
To further explore the intersection of design patterns and enterprise Java development, delve into the Official Spring Documentation and Design Patterns: Elements of Reusable Object-Oriented Software.
Happy coding!