Revolutionize Design: Domain Objects Over Infrastructure
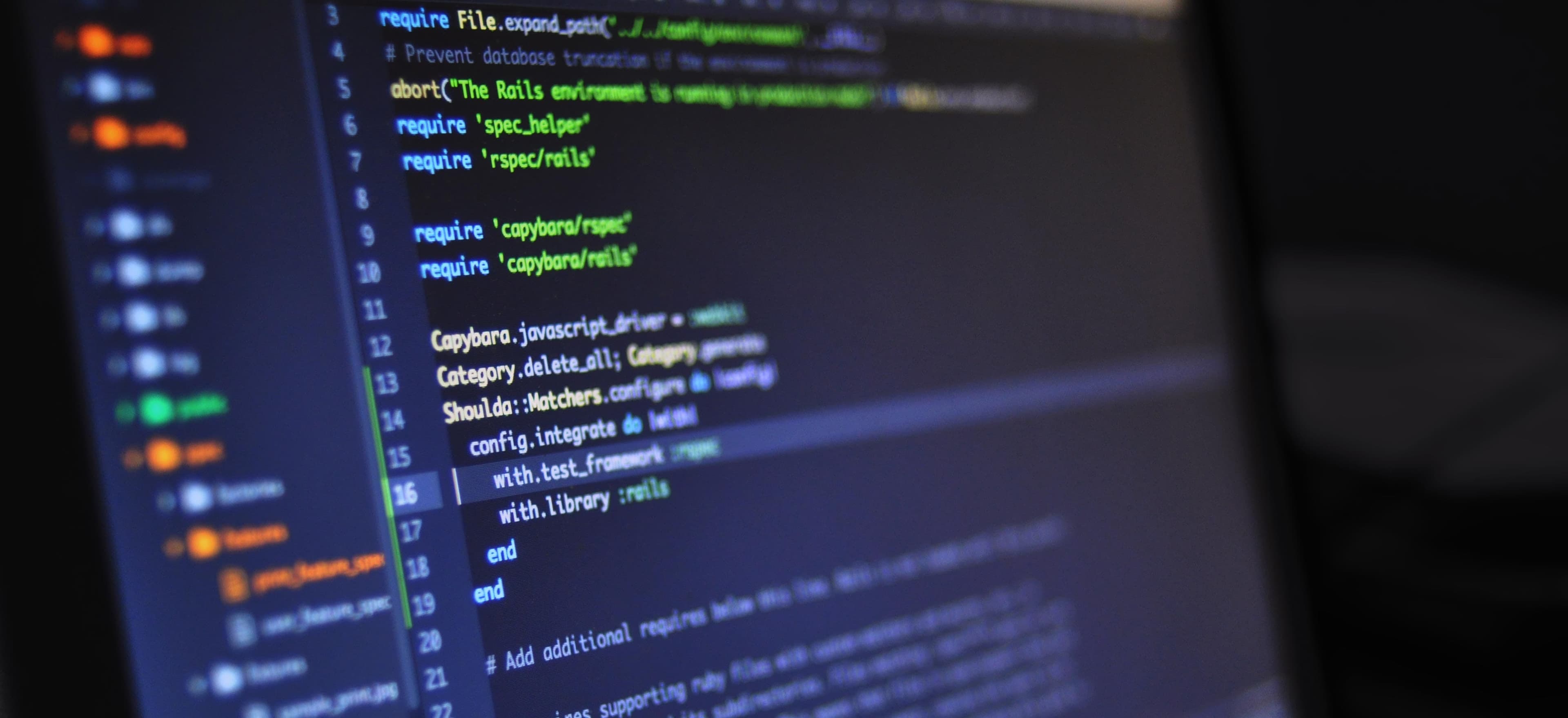
- Published on
Revolutionize Design: Domain Objects Over Infrastructure
When it comes to building robust and maintainable Java applications, the design of your domain objects plays a critical role. Embracing a domain-driven design approach empowers developers to create software that accurately represents real-world concepts and behaviors. In this article, we will delve into the importance of placing domain objects at the forefront of your design, emphasizing the benefits of this approach and demonstrating how it can lead to more cohesive, scalable, and maintainable systems.
The Pitfalls of Infrastructure-Centric Design
In many Java applications, the design process often starts with infrastructure concerns such as databases, frameworks, and external services. As a result, domain objects end up being shaped and constrained by the technical aspects of the system, leading to a tightly coupled and rigid architecture. This approach can hinder the adaptability and evolution of the software, as changes in the domain logic become arduous due to the entanglement with infrastructure dependencies.
Embracing Domain-Driven Design
Domain-Driven Design (DDD) advocates for placing the domain model at the center of the application's design, allowing it to drive the development process. By delineating the core domain concepts and behaviors and encapsulating them within domain objects, developers can create a more expressive and comprehensible system that aligns closely with the problem domain.
Benefits of Domain Objects-Centric Design
Improved Maintainability
When domain objects take precedence in the design, they become the focal point for business logic implementation. This separation allows for better maintainability, as changes to business rules and requirements can be localized within the domain objects without causing cascading modifications across the entire codebase.
Enhanced Testability
Domain objects, when decoupled from infrastructure concerns, are inherently more testable. By isolating the domain logic from external dependencies, unit testing becomes more straightforward and effective, leading to higher test coverage and confidence in the correctness of the system's behavior.
Increased Flexibility
A domain-driven design approach promotes a more flexible and adaptable architecture. As domain objects encapsulate the core business rules, they can evolve independently of the infrastructure, allowing the system to respond more gracefully to changing business needs and technical requirements.
Clearer Communication
By closely modeling the domain concepts in the code, domain objects facilitate clearer communication between developers and domain experts. The ubiquitous language used in DDD ensures that the terminology used in the code aligns with the language used in the business domain, fostering better collaboration and understanding.
Implementing Domain Object-Centric Design in Java
Let's explore how to implement domain-driven design principles in Java by placing a spotlight on domain objects and emphasizing their primacy in shaping the application.
Defining Domain Objects
public class Order {
private String orderId;
private List<OrderItem> orderItems;
private Money totalAmount;
private boolean isComplete;
// Constructors, getters, setters, and domain-specific methods
}
public class OrderItem {
private String productId;
private int quantity;
// Constructors, getters, setters, and domain-specific methods
}
public class Money {
private BigDecimal amount;
private Currency currency;
// Constructors, getters, setters, and domain-specific methods
}
In the example above, we define domain objects representing the fundamental concepts within an e-commerce domain: Order
, OrderItem
, and Money
. These objects encapsulate the essential attributes and behaviors related to orders and financial transactions, devoid of any infrastructure-related concerns.
Encapsulating Business Rules
public class Order {
// ...
public void addOrderItem(OrderItem orderItem) {
// Business logic for adding an order item
}
public void completeOrder() {
if (isComplete) {
throw new IllegalStateException("Order is already complete");
}
// Business logic for completing the order
isComplete = true;
}
// Other domain-specific methods
}
By encapsulating business rules and behaviors within the domain objects, we ensure that the integrity and consistency of the domain model are upheld. This encapsulation enables the domain logic to evolve independently, fostering a more resilient and adaptable system.
Keeping Infrastructure Separate
public interface OrderRepository {
void save(Order order);
Order findById(String orderId);
// Other domain-specific methods
}
public class JpaOrderRepository implements OrderRepository {
// Implementation of the repository interface using JPA
}
public class OrderService {
private OrderRepository orderRepository;
public void placeOrder(Order order) {
// Business logic for placing an order
orderRepository.save(order);
}
// Other domain-specific methods
}
Separating infrastructure concerns, such as persistence and external services, from the domain objects is pivotal. By employing repository interfaces and implementing them with technology-specific details, we keep the domain objects untainted by infrastructure intricacies.
Closing the Chapter
In the realm of Java development, prioritizing domain objects over infrastructure yields an array of benefits that are pivotal to creating resilient, adaptable, and maintainable software systems. By leveraging domain-driven design principles, developers can distill the core business concepts into expressive and testable domain objects, fostering clearer communication and better aligning the codebase with the problem domain.
As you embark on your journey to build Java applications, make the conscious decision to revolutionize your design by placing domain objects at the helm of your architectural decisions. Embrace domain-driven design, and witness the transformation it brings to your software's design, implementation, and longevity.
Incorporate domain objects in your design, empower your developers and enhance the maintainability of your system, following these principles will lead to a success story for your software development project.
Remember to always prioritize domain objects over infrastructure, and unlock the potential of your software architecture.
So, are you ready to revolutionize your design by embracing domain objects over infrastructure? Let's embark on this transformative journey and unlock the full potential of your Java applications.
The future of your software design awaits - in the embrace of domain objects-centric architecture.
Comment below with your thoughts and experiences on domain objects-centric design in Java, and let's start a conversation!