Seamless Integration: Kafka Meets Lightstreamer for Airports
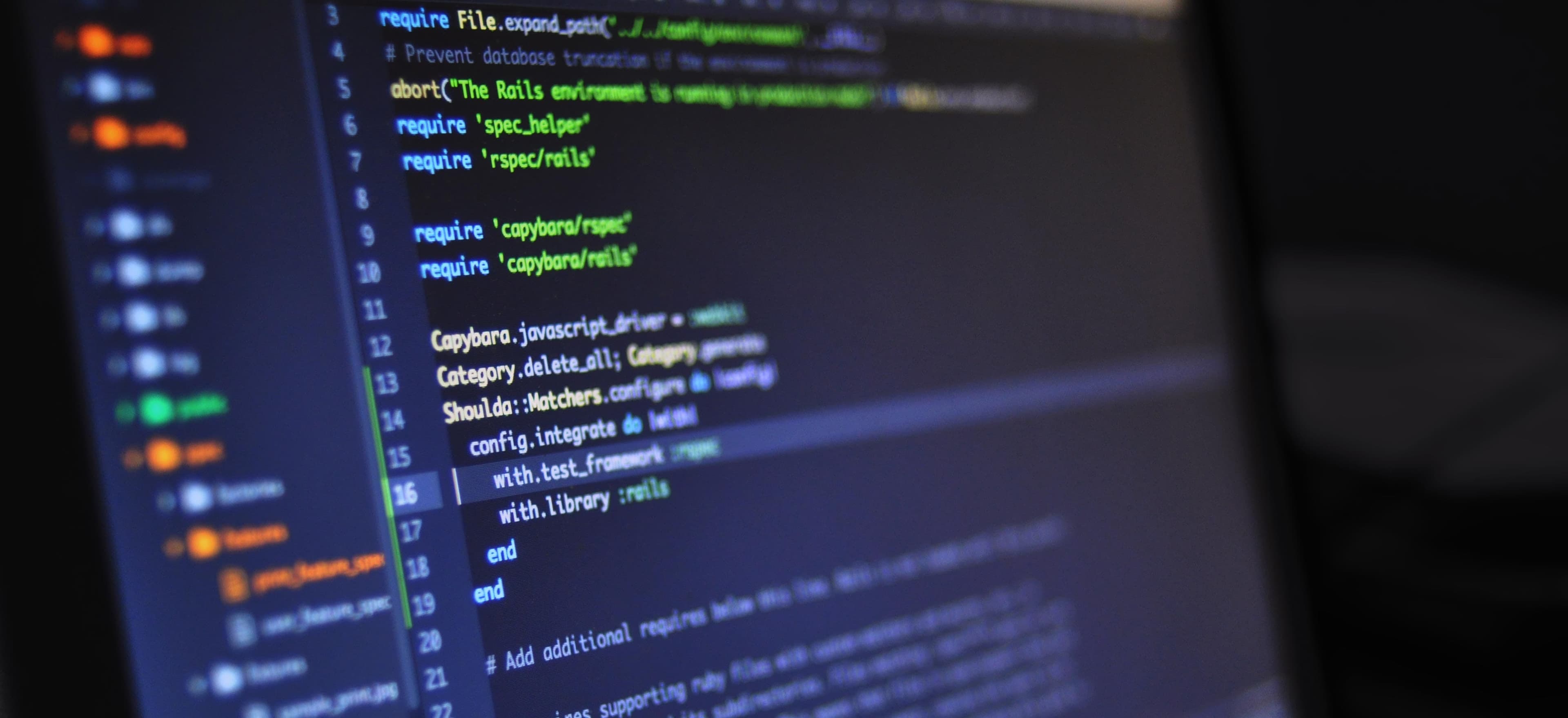
- Published on
Seamless Integration: Kafka Meets Lightstreamer for Airports
Airports, being dynamic and complex environments, require real-time data processing and communication systems to ensure smooth operations and passenger safety. In this article, we'll explore the seamless integration of Apache Kafka and Lightstreamer to meet the real-time data challenges of airports. We'll delve into the use case, architecture, and the technical implementation of this integration using Java.
Understanding the Use Case
Imagine an airport that needs to handle real-time data from various sources such as flight schedules, passenger information, baggage handling systems, security checkpoints, and more. This data needs to be processed, analyzed, and disseminated in real-time to ensure efficient operations and a positive passenger experience.
For such a scenario, a robust data streaming platform is essential to handle the high volume and velocity of data. Apache Kafka, known for its distributed, fault-tolerant, and real-time data streaming capabilities, fits perfectly in this scenario. On the other hand, Lightstreamer, with its real-time messaging and data streaming server, provides seamless integration with backend systems and web applications, making it an ideal candidate for real-time data delivery in airports.
Architecture Overview
Before diving into the technical details, let's outline the architecture of our solution. We'll have Kafka acting as a data source, producing real-time events such as flight updates, baggage status, and security checkpoint alerts. These events will be consumed by a Java application that acts as a bridge between Kafka and Lightstreamer. The Java application will transform and publish the events to Lightstreamer, which in turn will deliver the real-time data to various airport systems and applications.
This architecture ensures that the airport's various systems and applications are seamlessly integrated and kept up to date with real-time data, enabling efficient operations and decision-making.
Technical Implementation using Java
Setting Up Kafka
Firstly, we need to set up Kafka and create a topic for our real-time events. Using the Kafka Java API, we'll produce sample flight update events to the Kafka topic. Here's a simplified example of how we can achieve this:
Properties props = new Properties();
props.put("bootstrap.servers", "localhost:9092");
props.put("key.serializer", "org.apache.kafka.common.serialization.StringSerializer");
props.put("value.serializer", "org.apache.kafka.common.serialization.StringSerializer");
Producer<String, String> producer = new KafkaProducer<>(props);
ProducerRecord<String, String> flightUpdate = new ProducerRecord<>("flight-updates", "LH401", "Delayed");
producer.send(flightUpdate);
producer.close();
In this code snippet, we set up the Kafka producer with the necessary configuration and then produce a sample flight update event to the "flight-updates" topic. This event contains the flight number and its status, which will be consumed and processed further in our pipeline.
Bridging Kafka and Lightstreamer with Java
Now, let's take a look at how we can bridge Kafka and Lightstreamer using a Java application. We'll consume the flight update events from Kafka, transform them if necessary, and publish them to Lightstreamer. Here's a simplified example of how we can achieve this using the Lightstreamer Java Client Library:
// Create a Lightstreamer connection
LightstreamerClient client = new LightstreamerClient("https://push.lightstreamer.com", "DEMO");
// Define a subscription for the flight updates
Subscription flightSubscription = new Subscription("DISTINCT", new String[]{"flightNumber", "status"}, "flight-updates", "UPDATE");
// Add a subscription listener to handle real-time updates
flightSubscription.addListener(new SubscriptionListener() {
@Override
public void onItemUpdate(ItemUpdate itemUpdate) {
// Publish the real-time update to Lightstreamer
client.sendMessage("flightUpdate", itemUpdate.getValue("flightNumber") + " is " + itemUpdate.getValue("status"));
}
});
// Connect to Lightstreamer server and subscribe to the flight updates
client.connect();
client.subscribe(flightSubscription);
In this code snippet, we create a connection to the Lightstreamer server, define a subscription for the flight updates, and handle real-time updates using a subscription listener. Whenever a flight update event is received from Kafka, it is transformed and published to Lightstreamer for real-time delivery.
Ensuring Seamless Integration
By bridging Kafka and Lightstreamer using Java, we ensure seamless integration of airport systems and applications with real-time data. The high-volume flight updates, baggage status, and security checkpoint alerts are efficiently processed and delivered in real-time, enabling airport staff to make informed decisions and ensuring a smooth passenger experience.
Final Considerations
In this article, we've explored the seamless integration of Apache Kafka and Lightstreamer for real-time data processing in airports. We've understood the use case, outlined the architecture, and delved into the technical implementation using Java. Through this integration, airports can efficiently handle the high volume and velocity of real-time data, ensuring smooth operations and passenger safety.
For more insights on Apache Kafka, Lightstreamer, and their applications in real-time data processing, feel free to explore the following resources:
- Apache Kafka Documentation
- Lightstreamer Documentation
By leveraging the power of Apache Kafka and Lightstreamer, airports can ensure seamless integration and real-time data delivery, setting a new standard for efficient operations and passenger experience.